Page Navigation using CodeIgniter Pagination
Submitted by admin on Friday, November 16, 2012 - 09:52.
In our previous tutorial called “Getting Started with CodeIgniter” I discuss on how to install and configure CodeIgniter. This time I will teach you on how to create a simple “Page Navigation”. This is just a comparison of my previous code found at PHP Page Navigation. You’ll learn the difference between the standard PHP and using a CodeIgniter framework.
Now let us configure the CodeIgniter.
- Open autoload.php under Config folder using your PHP Editor.
- Open config.php under Config folder.
- Open database.php under Config folder.
- Open routes.php under Config folder.
- Now create a file called pagination.php under controllers folder and paste the following code:
- class Pagination extends CI_Controller
- {
- public function __construct() {
- parent:: __construct();
- $this->load->helper("url");
- $this->load->model("Countries_Model");
- $this->load->library("pagination");
- }
- public function index() {
- $config["base_url"] = base_url() . "pagination";
- $config["total_rows"] = $this->Countries_Model->record_count();
- $config["per_page"] = 10;
- $config["uri_segment"] = 2;
- $this->pagination->initialize($config);
- $page = ($this->uri->segment(2))? $this->uri->segment(2) : 0;
- $data["results"] = $this->Countries_Model
- ->get_countries($config["per_page"], $page);
- $data["links"] = $this->pagination->create_links();
- $this->load->view("pagination", $data);
- }
- }
- Create a file called countries_model.php under models folder and paste the following code:
- class Countries_Model extends CI_Model
- {
- public function __construct() {
- parent::__construct();
- }
- public function record_count() {
- return $this->db->count_all("tblcountries");
- }
- public function get_countries($limit, $start) {
- $this->db->limit($limit, $start);
- $query = $this->db->get("tblcountries");
- if ($query->num_rows() > 0) {
- foreach ($query->result() as $row) {
- $data[] = $row;
- }
- return $data;
- }
- return false;
- }
- }
- Lastly, create a file called pagination.php under views folder and paste the following code:
- <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
- <html xmlns="http://www.w3.org/1999/xhtml">
- <head>
- <meta charset="utf-8">
- <title>Pagination with CodeIgniter</title>
- </head>
- <body>
- <div id="container">
- <h1 style="text-align:center">Page Navigation in PHP</h1>
- <table width="400" border="1" align="center">
- <tr>
- <td width="100" bgcolor="#CCCCCC"><p>Country ID</p></td>
- <td width="300" bgcolor="#CCCCCC">Country</td>
- </tr>
- <h1 style="text-align:center">Countries</h1>
- <div id="body">
- <?php
- foreach($results as $data) {
- ?>
- <tr>
- <td><?php echo $data->CountryID ?></td>
- <td><?php echo $data->Country ?></td>
- </tr>
- <?php
- }
- ?>
- </table>
- <div style="text-align:center"><?php echo $links; ?></div>
- </div>
- </div>
- </body>
- </html>
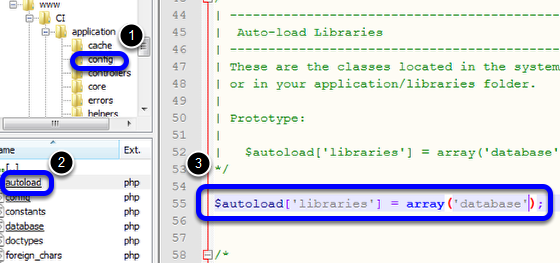
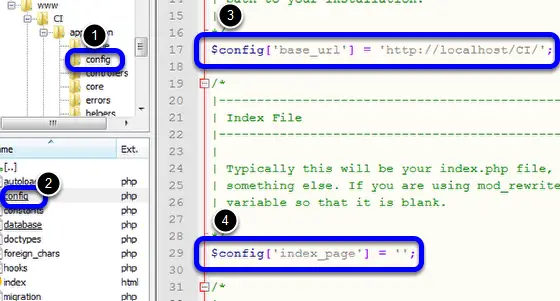
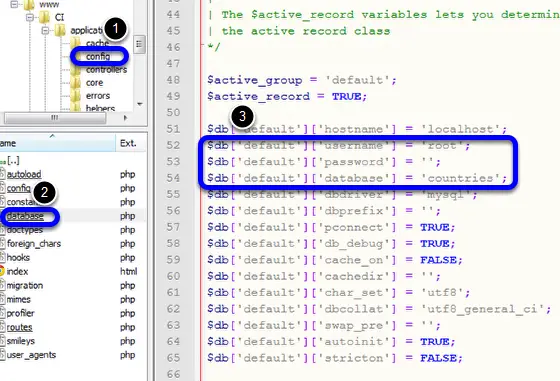
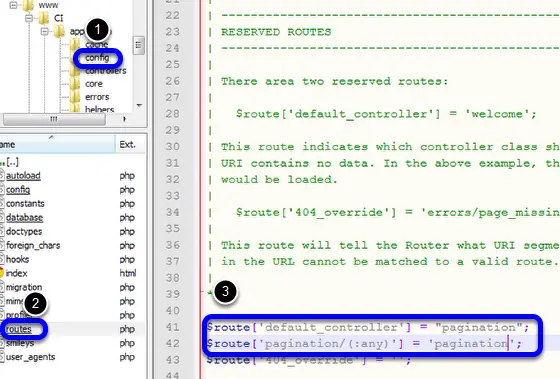
Now, open your browser and type:
http://localhost/CI/
Note: this assumes that you extract the CodeIgniter framework under www folder and named it as “CI”.
Comments
Just follow the tutorial and
Just follow the tutorial and your good to go...
Every code is already in here. Download the CodeIgniter framework.
codeigniter
why is it undefined variable: links, results,
in filename:pages/pagination.php
and invalid argument supplied for foreach()
An uncaught Exception was
An uncaught Exception was encountered
Type: RuntimeException
Message: Unable to locate the model you have specified: Countries_model
Filename: /opt/lampp/htdocs/CI/system/core/Loader.php
Line Number: 344
Source Code
This is really a good start up for life because of this website also. Because we know that Stackoverflow helps us to findout best resource.
Also this website inspire a developer to develop more and more skill about programming. Because of this .Website
.Thank you so much for create this website.
Add new comment
- Add new comment
- 663 views