CRUD Operation and SQL App using C# with Source Code
This is a simple Source Code called CRUD Operation with Search in C# and SQL. This simple prohram will help you to learn how create a C# program that has a Create, Read, Update and Delete features with Search data. The source code itself uses only a single form where the said operation/feature can be done.
Controls & Database
The below list are the controls I have used.
- Forms
- TextBox
- Label
- Button
- ComboBox
- DataGridView
I have created a test database in my SQL Server and created a "Register" Table as the storage of the data. The following list are the columns inside the Register Table
.
- id
- Name
- Salery
- Age
- Gender
- Tax
Interface
The image presented below the interface I created for this simple program.
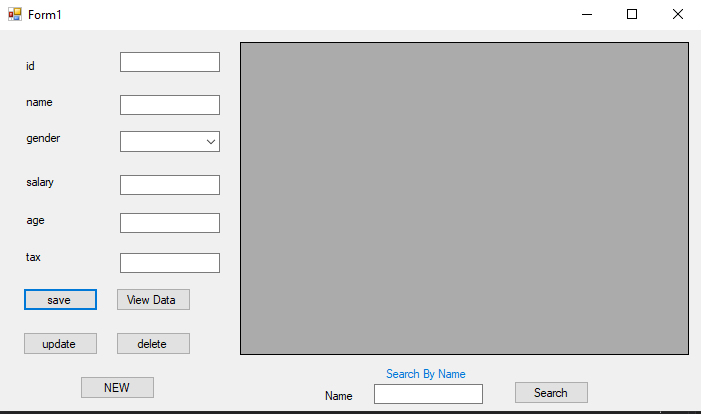
Scripts
The following scripts/code are ones I have used to manage the data.
Database Connection
- SqlConnection conn = new SqlConnection(@"Data Source=DESKTOP-2LLV29I\MSSQLSERVER01;Initial Catalog=test1;Integrated Security=True");
Save to Database
- private void btnsave_Click(object sender, EventArgs e)
- {
- if (txtid.Text == "" || txtname.Text == "" || txtsalery.Text == "" || txttax.Text == "" || txtage.Text == "" || comboBox1.Text == "")
- {
- MessageBox.Show("please fill the cell first");
- }
- else
- {
- conn.Open();
- SqlDataAdapter sda = new SqlDataAdapter("insert into Register(id,name,gender,salery,age,tax)values('" + txtid.Text + "','" + txtname.Text + "','" + comboBox1.Text + "','" + txtsalery.Text + "','" + txtage.Text + "','" + txttax.Text + "')", conn);
- sda.SelectCommand.ExecuteNonQuery();
- conn.Close();
- MessageBox.Show("data entered succesfully. . . .");
- panel1.Enabled = false;
- }
- }
View Data
Update Data
- private void btnUpdate_Click(object sender, EventArgs e)
- {
- if (panel1.Enabled == true)
- {
- if (txtid.Text == "" || txtname.Text == "" || txtsalery.Text == "" || txttax.Text == "" || txtage.Text == "" || comboBox1.Text == "")
- {
- MessageBox.Show("please fill the cell first");
- }
- else
- {
- conn.Open();
- SqlDataAdapter sda = new SqlDataAdapter("UPDATE Register SET id ='" + txtid.Text + "',name = '" + txtname.Text + "',Gender = '" + comboBox1.Text + "', Salery = '" + txtsalery.Text + "',age = '" + txtage.Text + "',tax = '" + txttax.Text + "' where id ='" + txtid.Text + "'", conn);
- sda.SelectCommand.ExecuteNonQuery();
- conn.Close();
- MessageBox.Show("data updated succesfully. . . .");
- load_data();
- panel1.Enabled = false;
- }
- }
- else
- {
- MessageBox.Show("please select what you want to update");
- }
- }
Delete Data
- private void btndelete_Click(object sender, EventArgs e)
- {
- if (panel1.Enabled == true)
- {
- if (txtid.Text == "" || txtname.Text == "" || txtsalery.Text == "" || txttax.Text == "" || txtage.Text == "" || comboBox1.Text == "")
- {
- MessageBox.Show("please select the record");
- }
- else
- {
- conn.Open();
- SqlDataAdapter sda = new SqlDataAdapter("delete from Register where id ='" + txtid.Text + "'", conn);
- sda.SelectCommand.ExecuteNonQuery();
- conn.Close();
- MessageBox.Show("data deleted succesfully. . . .");
- load_data();
- panel1.Enabled = false;
- }
- }
- else
- {
- MessageBox.Show("please select the record first");
- }
- }
The source code is free to download. Feel Free to download the source code to understand more about how does the program work.
How to Run
Requirements
- Download and Install Microsoft Visual Studio Software/li>
- Download and Install Microsoft SQL Server Express
- Download and Install SQL Server Management Studio
Installation/Setup
- Download and Extract the source code zip file. (download button is located the below)
- Locate the MDF File in the extracted source code folder. The file is known as "test1.mdf".
- Open your SQL Server Management Studio Software and connect to a server.
- Attach the MDF file in the database.
- Locate the solution file in the extracted source code folder. The file is known as "Data_Entry.sln".
- Open the solution file with your MS Visual Studio software.
- Configure the
SqlConnection
according to your database connection string. - Press the "F5" key to start the program.
Demo
That's it! I hope this CRUD (Create, Read, Update, and Delete) with Search Program in C# and SQL will help you to develop your programming skills.
Enjoy :)Note: Due to the size or complexity of this submission, the author has submitted it as a .zip file to shorten your download time. After downloading it, you will need a program like Winzip to decompress it.
Virus note: All files are scanned once-a-day by SourceCodester.com for viruses, but new viruses come out every day, so no prevention program can catch 100% of them.
FOR YOUR OWN SAFETY, PLEASE:
1. Re-scan downloaded files using your personal virus checker before using it.
2. NEVER, EVER run compiled files (.exe's, .ocx's, .dll's etc.)--only run source code.
Comments
Add new comment
- Add new comment
- 15099 views