Inheritance
Submitted by Muzammil Muneer on Sunday, October 26, 2014 - 23:45.
Inheritance
In this part you will learn: 1. What is inheritance? 2. Why we need inheritance? 3. Syntax 4. Program 5. Output Inheritance In c++, the concept of inheritance is just like inheritance in family. As we all are child of our parents, we have inherited their many things from them. Similar is the case in programming. We can create inheritance between classes, we can have more than one class in a program, we can make one class the base class, also known as parent class and declare the other one as derived class, or simply child class. There are different inheritance levels in class inheritance, i.e. the derived class can have access to either all the members of the base class or some of them. The level of inheritance is determined by the type of inheritance we are doing. It can be, • Private • Public • Protected All of these inheritances have different levels of access of the base class. The following are the effects of different inheritances on members of base class with different access specifications on the derived classes. • Private a. Private becomes inaccessible for both (class and public) b. Public becomes Private c. Protected becomes Private • Public a. Private becomes inaccessible for both (class and public) b. Public remains Public. c. Protected remains Protected • Protected a. Private becomes inaccessible for both (class and public) b. Public becomes Protected c. Protected remains Protected Note that there can be multiple levels of heritance, i.e. a class which is being derived from a base class itself can have a derived class of its own. Furthermore, there can be more than one derived classes from a base class. Syntax Following is the syntax of declaring one class the derived class of the other. class Class_Name1 { //class definition } class Class_Name2:Inheritance_type Class_name1 { //function definition } In the above syntax we have declared a base class Class_Name1 the base class of class Class_Name2. In the second class after defining the name we have used colon, then will write the Inheritance_type which can be private, public or protected. Then we will write the name of the base class. Program with Inheritance In this example I have defined the member function of a Class. Basic Step:- #include<iostream>
- #include<conio.h>
- #include<string.h>
- using namespace std;
- class person
- {
- public: //access specifier
- string name;
- string gender;
- int age;
- person(string n, string g, int a) :name(n), gender(g), age(a){
- }
- };
- class student:private person
- {
- public: //access specifier
- int roll_no;
- int no_of_subjects;
- student(string n, string g, int a, int r, int no) :person(n,g,a),roll_no(r), no_of_subjects(no){
- }
- void print_student_info(){
- cout << endl << "Student information:" << endl << endl;
- cout << "Name: " << name << endl;
- cout << "Gender: " << gender << endl;
- cout << "Age: " << age << endl << endl;
- cout << "Roll number: " << roll_no << endl;
- cout << "No of subjects: " << no_of_subjects << endl;
- }
- };
- class employee:private person
- {
- public:
- int employee_id;
- int experience;
- employee(string n, string g, int a, int e_id, int e) :person(n, g, a), employee_id(e_id), experience(e){
- }
- void print_employee_info(){
- cout << endl << endl << "Employee information:" << endl << endl;
- cout << "Name: " << name << endl;
- cout << "Gender: " << gender << endl;
- cout << "Age: " << age << endl << endl;
- cout << "Employee id: " << employee_id << endl;
- cout << "Experience: " << experience << endl;
- }
- };
- int main()
- {
- student s1("John", "male", 19, 4343, 5);
- employee e1("Elia", "female", 35,1067, 8);
- s1.print_student_info();
- e1.print_employee_info();
- }
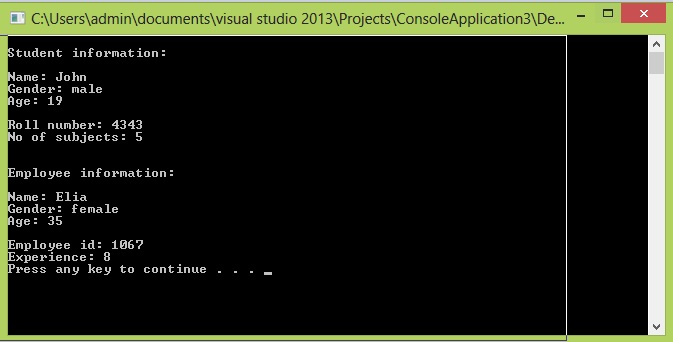
Comments
Add new comment
- Add new comment
- 129 views