Setters And Getters
Submitted by Muzammil Muneer on Friday, October 17, 2014 - 10:21.
Setters and Getters
In this part you will learn: 1. What are setters and getters? 2. Why we need setters and getters? 3. Program using setters and getters 4. Showing output What are Setters and Getters? As the name suggests, setters are functions used to set values whereas getters are used to get values. Setters and getters functions are usually public data members of the class. Setters and getters are used for private or protected member variables. As we know that me cannot directly access the private or protected member variables of a class, so we use indirect access method using setters and getters. For example we want to access a member variable namely “age”, we will use the function getage() and setage(int a) to get the value stored in member variable “age” and to set the value of “age” which is passed as parameter in variable a respectively. We will discuss a complete example of setters and getters at the end of this topic. Why we need Setters and Getters? While writing huge programs, programmers restrict themselves from directly changing or accessing the values of member variables of an object because it creates a lot of mess finding and removing the error in that case. As a solution we use setters and getters functions which are part of that class and can be used outside the class providing indirect access to private member variables of the class. Program using setters and getters Basic Step:- #include<iostream>
- #include<conio.h>
- using namespace std;
- class info
- {
- public:
- int id;
- protected:
- int contact;
- private:
- int age;
- public:
- int getcontact(){
- return contact;
- }
- void setcontact(int c){
- contact = c;
- }
- int getage(){
- return age;
- }
- void setage(int a){
- age = a;
- }
- };
- <strong>Main</strong>
- int main()
- {
- info muzzi; //initializing a variable muzzi of type info
- muzzi.id = 4156; //allowed because it is public member variable
- muzzi.setcontact(1234); //indirectly accessing protected member "contact"
- muzzi.setage(20); //indirectly accessing private member "age"
- cout << muzzi.id<<endl; //allowed because it is public member function
- cout << muzzi.getcontact()<<endl; //indirectly getting the value stored in "contact"
- cout << muzzi.getage()<<endl; //indirectly getting the value stored in "age"
- }
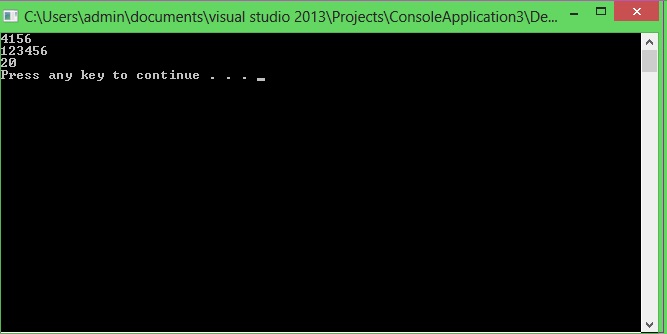
Add new comment
- 67 views