How to fix "Fatal error: __autoload() is no longer supported" in PHP?
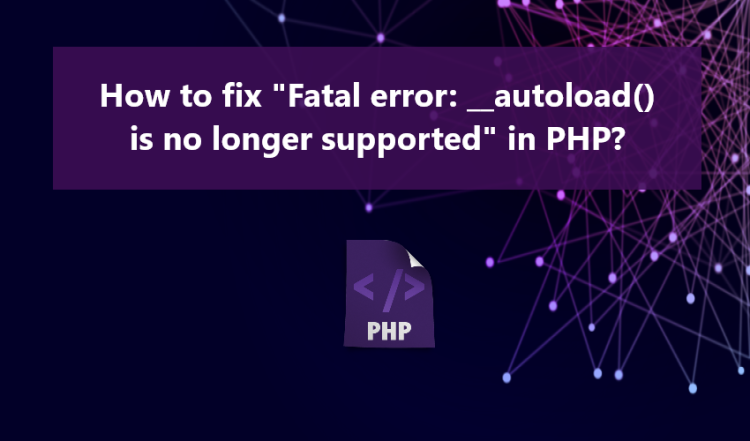
This article delves into an exploration of the PHP Error message that reads "Fatal error: __autoload() is no longer supported." If you currently encounter this error during your PHP project development, this article will provide you with insights and knowledge to resolve this issue.
PHP generates various types of errors, including the one we're discussing here. Besides the "Fatal error: __autoload() is no longer supported," Fatal Errors are often thowns with exceptions such as "PHP Fatal error: Uncaught TypeError: implode(): Argument ...", "Fatal Error: Cannot Redeclare class," "Fatal error: Uncaught Error: Using $this when not in object context," and more.
What is Fatal Error in PHP?
In PHP, a "Fatal Error" is a critical issue in your code that immediately stop the script execution. Common causes of such errors include undefined functions or methods, exceeding the maximum execution time or memory limit, fatal errors in included files, constructor problems in object-oriented code, troublesome class definitions, exceeding the nesting level, and PHP configuration errors. These errors produce informative messages indicating their nature, file, line number, and a stack trace. To resolve them, you must identify and rectify the underlying code issues or adjust the server's PHP configuration. Effective error handling and prevention are essential for maintaining smooth PHP applications and ensuring a seamless user experience.
Why does the "Fatal error: __autoload() is no longer supported" PHP error occur?
This error occurs when attempting to use the __autoload() built-in function in PHP. This function tries to load undefined classes from various PHP script files. The "Fatal error: __autoload() is no longer supported" arises because this function was only available and functional before PHP version 7.2. Starting from PHP version 8.0, this function has been removed.
Let's consider a simple PHP project with a PHP script file named MyClass.php, which contains a PHP class needed in our index file. Typically, we can include this file as shown in the following snippet:
Example #1:
- <?php
- require_once("./MyClass.php");
- $myClass = new MyClass;
- echo $myClass->test();
- ?>
While the snippet above is effective for smaller projects, it becomes time-consuming and impractical when dealing with larger-scale projects that may involve numerous class files. This situation is particularly common when working with PHP frameworks that follow the MVC (Model-View-Controller) architectural pattern. If we were to apply the same approach as shown in the previous snippet to such projects, the script's complexity would increase significantly:
Example #2:
- <?php
- require_once("./MyClass.php");
- require_once("./SecondClass.php");
- require_once("./ThirdClass.php");
- require_once("./FourthClass.php");
- //... and so on ...
- ?>
To avoid such as the scenario provided above, PHP offers a useful built-in function known as __autoload(). This function plays a crucial role in optimizing our PHP script by automatically loading undefined classes. Rather than adopting the approach shown in Example #2, we can simplify our code as follows:
Example #3:
However, it's important to note that the __autoload() function has been deprecated and subsequently removed in later versions of PHP. Utilizing this deprecated function will result in script execution termination and trigger an error message, specifically, the "Fatal error: __autoload() is no longer supported".
Example #4:
Running the above script using PHP version 7.2 or later version will trigger the error like the following:
Solution
The alternative to the deprecated PHP __autoload() function is the use of the spl_autoload_register() function. This function serves to register a specified function as the __autoload() function. When utilizing this function, it is essential to specify the autoload function for registration; otherwise, the default spl_autoload() will be registered instead.
With spl_autoload_register(), you can effortlessly load classes automatically by designating the __autoload() function as the callback function, as demonstrated below:
Example #5:
- <?php
- // Load or Require the class File
- require_once("./{$class}.php");
- }else{
- }
- });
- $myClass = new MyClass;
- echo $myClass->test();
- ?>
Running the snippet above using the latest PHP version will ouput like the following:
Conclusion
Put simply, the PHP "Fatal error: __autoload() is no longer supported" occurs when attempting to use the deprecated built-in function called __autoload() in PHP versions equal to or newer than 7.2. To resolve this error, you can utilize the spl_autoload_register() function as an alternative to the deprecated __autoload() function.
That's it! I hope this article will help you address the problem or error you are currently struggling. Explore more on this website for more Free Source Codes, Tutorials, and other informative articles covering PHP and various other programming languages.
Happy Coding =)
- 5705 views