Retrieve Database Tables from MySQL Database using Visual Basic.Net into ComboBox
Submitted by GeePee on Monday, April 13, 2015 - 23:04.
In this tutorial, I’m going to show you how to create an application that will load all the MySQL database and store it into a combobox displaying all the table fields in the datagridview.
To start in this program you need to open visual basic and create a new application and name it as “dbcreator01”. Then add some tools from the toolbox to our form, these tools are the following: seven labels, one button, one combobox and a datagridview.
Then change some property of the objects and arrange it same as shown below.
This time, we’re going to add functionality to our application. First, we need to create another module and name it as “mySelect” module, add the following code:
This code will handle the connections between the visual basic and MySQL database so that we could freely work with our MySQL Database and other functions especially in retrieving of data from a MySQL database in Visual Basic.
Next, double click our form, and add the following code:
Then, double the “btngo”. And add the following code:
Before executing this application, make sure that have installed MySQL database in your machine. If not you can download XAMPP or WAMP and install it to your computer.
To test this program, just press “F5”.
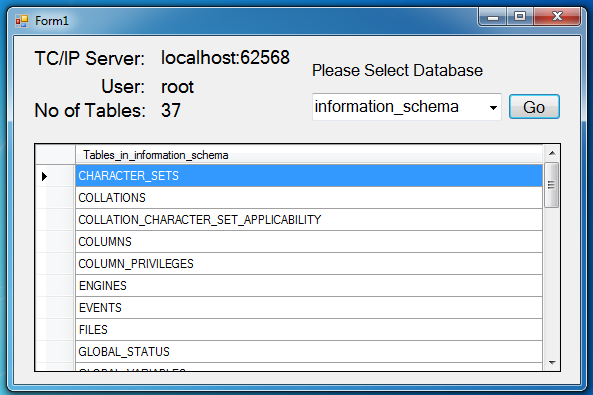
- Imports MySql.Data.MySqlClient
- Module mySelect
- Dim cmd As New MySqlCommand
- Dim da As New MySqlDataAdapter
- Public total As Integer
- Dim con As MySqlConnection = jokenconn()
- Public Function jokenconn() As MySqlConnection
- Return New MySqlConnection("server=localhost;user id=root;password=;database='" & Form1.cbdb.SelectedValue & "'")
- End Function
- Public Sub findThis(ByVal sql As String)
- Try
- con.Open()
- With cmd
- .Connection = con
- .CommandText = sql
- End With
- Catch ex As Exception
- Form1.Text = ex.Message
- End Try
- da.Dispose()
- End Sub
- Public Sub filltable(ByVal dtgrd As Object)
- Dim publictable As New DataTable
- Dim numrow As Integer
- Try
- da.SelectCommand = cmd
- da.Fill(publictable)
- dtgrd.DataSource = publictable
- numrow = publictable.Rows.Count
- ' dtgrd.Columns(0).Visible = False
- ' dtgrd.Columns(1).Visible = False
- da.Dispose()
- Catch ex As Exception
- End Try
- End Sub
- Public Sub filldbOBJ(ByVal obj As Object)
- Dim publictable As New DataTable
- Try
- da.SelectCommand = cmd
- da.Fill(publictable)
- ' publictable.Rows.Add("Please Select...")
- With obj
- .DataSource = publictable
- .DisplayMember = "Database"
- .ValueMember = "Database"
- End With
- ' dtgrd.Columns(1).Visible = False
- da.Dispose()
- Catch ex As Exception
- End Try
- End Sub
- Public Sub filltxtbox(ByVal obj As Object, ByVal obj1 As Object)
- Dim publictable As New DataTable
- Try
- da.SelectCommand = cmd
- da.Fill(publictable)
- obj.text = publictable.Rows(0).Item(1) 'user
- obj1.text = publictable.Rows(0).Item(2) 'server
- 'dtgrd.Columns(1).Visible = False
- da.Dispose()
- Catch ex As Exception
- End Try
- End Sub
- Public Sub fillcbOBJ(ByVal tableObj As Object, ByVal dbObj As Object)
- Dim publictable As New DataTable
- Try
- da.SelectCommand = cmd
- da.Fill(publictable)
- With tableObj
- .DataSource = publictable
- .DisplayMember = "Tables_in_" & dbObj
- .ValueMember = "Tables_in_" & dbObj
- End With
- ' dtgrd.Columns(1).Visible = False
- da.Dispose()
- Catch ex As Exception
- End Try
- End Sub
- Public Sub fillFieldsOBJ(ByVal obj As Object)
- Dim publictable As New DataTable
- Try
- da.SelectCommand = cmd
- da.Fill(publictable)
- With obj
- .DataSource = publictable
- End With
- ' dtgrd.Columns(1).Visible = False
- da.Dispose()
- Catch ex As Exception
- End Try
- End Sub
- Public Sub filltblname(ByVal obj As Object, ByVal tblname As String)
- Dim publictable As New DataTable
- Try
- da.SelectCommand = cmd
- da.Fill(publictable)
- With obj
- .DataSource = publictable
- .DisplayMember = "Tables_in_" & tblname
- .ValueMember = "Tables_in_" & tblname
- End With
- ' dtgrd.Columns(1).Visible = False
- da.Dispose()
- Catch ex As Exception
- End Try
- End Sub
- Public Sub findThisTableCount(ByVal sql As String)
- Try
- con.Open()
- With cmd
- .Connection = con
- .CommandText = sql
- End With
- Catch ex As Exception
- Form1.Text = ex.Message
- End Try
- da.Dispose()
- End Sub
- Public Sub fillNo_tblCount(ByVal obj As Object)
- Dim publictable As New DataTable
- Try
- da.SelectCommand = cmd
- da.Fill(publictable)
- obj.text = publictable.Rows(0).Item(0)
- ' dtgrd.Columns(1).Visible = False
- da.Dispose()
- Catch ex As Exception
- End Try
- End Sub
- End Module
- Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
- 'using modules we just simply call this sub routine
- 'and state a specific query, this query will show all the database found in Database
- findThis("SHOW DATABASES")
- 'list down all the database names into the combobox
- filldbOBJ(cbdb)
- End Sub
- Private Sub btngo_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btngo.Click
- 'it select all the datafrom Processlist
- findThis("SELECT * FROM information_schema.PROCESSLIST")
- 'get the USER and the HOST name used
- filltxtbox(lbluser, lblhost)
- 'it count all the number of table based on the selected database
- findThisTableCount("SELECT COUNT( * ) FROM(information_schema.tables) WHERE table_schema = '" & cbdb.Text & "'")
- 'display the count of table
- fillNo_tblCount(lblCount)
- 'display all the tables from a specific database
- findThis("SHOW TABLES FROM " & cbdb.SelectedValue)
- 'and fill it into the datagridview
- fillFieldsOBJ(DataGridView1)
- End Sub