Page Flip in VB.NET
Submitted by donbermoy on Thursday, June 4, 2015 - 22:48.
This tutorial will teach you how to create a flipping of image program as a page in vb.net.
Now, let's start this tutorial!
1. Let's start with creating a Windows Form Application for this tutorial by following the following steps in Microsoft Visual Studio: Go to File, click New Project, and choose Windows Application.
2. Next, add two Buttons named Button2 for flipping right the image and Button3 for the left flipping. Add 10 PictureBox and two Timer. You must design your interface like this:
3. Now, we will do the coding.
We will first declare and initialize variables and have the image put in the C directory with a filename of 'test.jpg'.
We will then code for Ticking the Timer1 as it will be turning to the right page.
Then for Ticking the Timer2 as it will be turning to the Left page.
Now, we will do a sub procedure named test up to test7.This will create Create different parallelogram for drawing image.
Now, we will code for Button2 for flipping the page into the right position.
And for Button3 for flipping the page into the left position.
Lastly, in the Form_Load put all the tests in their.
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
Landline: 826-9296
E-mail:donbermoy@engineer.com
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
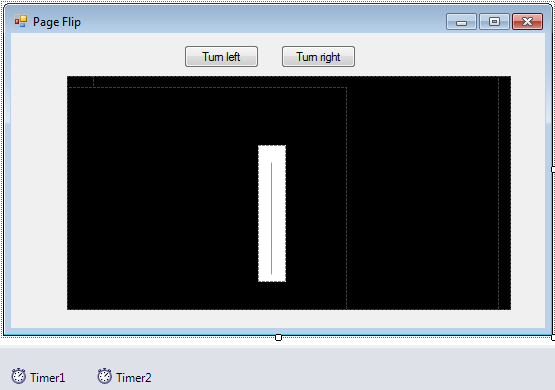
- Private count As Integer
- Private count1 As Integer
- Dim oImage As New Bitmap(500, 360)
- Dim oGraphics As Graphics = Graphics.FromImage(oImage)
- Dim imageFile1 As Image = Image.FromFile("c:/test.jpg")
- Private Sub Timer1_Tick(ByVal Sender As Object, ByVal e As EventArgs) Handles Timer1.Tick
- count = count + 1
- If count = 1 Then
- PictureBox2.Visible = True
- End If
- If count = 2 Then
- PictureBox2.Visible = False
- PictureBox3.Visible = True
- End If
- If count = 3 Then
- PictureBox3.Visible = False
- PictureBox4.Visible = True
- End If
- If count = 4 Then
- PictureBox4.Visible = False
- PictureBox5.Visible = True
- End If
- If count = 5 Then
- PictureBox5.Visible = False
- PictureBox6.Visible = True
- End If
- If count = 6 Then
- PictureBox6.Visible = False
- PictureBox7.Visible = True
- End If
- If count = 7 Then
- PictureBox7.Visible = False
- PictureBox8.Visible = True
- End If
- If count = 8 Then
- PictureBox8.Visible = False
- PictureBox9.Visible = True
- End If
- If count = 9 Then
- PictureBox9.Visible = False
- PictureBox10.Visible = True
- End If
- If count = 10 Then
- Exit Sub
- End If
- End Sub
- Private Sub Timer2_Tick(ByVal Sender As Object, ByVal e As EventArgs) Handles Timer2.Tick
- count1 = count1 - 1
- If count1 = 1 Then
- PictureBox2.Visible = True
- End If
- If count1 = 2 Then
- PictureBox3.Visible = False
- PictureBox2.Visible = True
- End If
- If count1 = 3 Then
- PictureBox4.Visible = False
- PictureBox3.Visible = True
- End If
- If count1 = 4 Then
- PictureBox5.Visible = False
- PictureBox4.Visible = True
- End If
- If count1 = 5 Then
- PictureBox6.Visible = False
- PictureBox5.Visible = True
- End If
- If count1 = 6 Then
- PictureBox7.Visible = False
- PictureBox6.Visible = True
- End If
- If count1 = 7 Then
- PictureBox8.Visible = False
- PictureBox7.Visible = True
- End If
- If count1 = 8 Then
- PictureBox9.Visible = False
- PictureBox8.Visible = True
- End If
- If count1 = 9 Then
- PictureBox10.Visible = False
- End If
- If count1 = 10 Then
- Exit Sub
- End If
- End Sub
- Private Sub test()
- Dim oImage As New Bitmap(500, 360)
- Dim oGraphics As Graphics = Graphics.FromImage(oImage)
- Dim imageFile1 As Image = Image.FromFile("c:/test.jpg")
- ' Create parallelogram for drawing image.
- Dim ulCorner1 As New Point(50, 92)
- Dim urCorner1 As New Point(360, 92)
- Dim llCorner1 As New Point(50, 340)
- Dim destPara1 As Point() = {ulCorner1, urCorner1, llCorner1}
- ' Create rectangle for source image.
- Dim srcRect As New Rectangle(50, 50, 800, 533)
- Dim units As GraphicsUnit = GraphicsUnit.Pixel
- oGraphics.DrawImage(imageFile1, destPara1, srcRect, units)
- Me.PictureBox2.Image = oImage
- End Sub
- Private Sub test1()
- Dim oImage As New Bitmap(500, 360)
- Dim oGraphics As Graphics = Graphics.FromImage(oImage)
- Dim imageFile1 As Image = Image.FromFile("c:/test.jpg")
- ' Create parallelogram for drawing image.
- Dim ulCorner1 As New Point(100, 35)
- Dim urCorner1 As New Point(360, 104)
- Dim llCorner1 As New Point(100, 275)
- Dim destPara1 As Point() = {ulCorner1, urCorner1, llCorner1}
- ' Create rectangle for source image.
- Dim srcRect As New Rectangle(50, 50, 800, 533)
- Dim units As GraphicsUnit = GraphicsUnit.Pixel
- oGraphics.DrawImage(imageFile1, destPara1, srcRect, units)
- Me.PictureBox3.Image = oImage
- End Sub
- Private Sub test2()
- Dim oImage As New Bitmap(500, 360)
- Dim oGraphics As Graphics = Graphics.FromImage(oImage)
- Dim imageFile1 As Image = Image.FromFile("c:/test.jpg")
- ' Create parallelogram for drawing image.
- Dim ulCorner1 As New Point(200, 7)
- Dim urCorner1 As New Point(360, 104)
- Dim llCorner1 As New Point(200, 250)
- Dim destPara1 As Point() = {ulCorner1, urCorner1, llCorner1}
- ' Create rectangle for source image.
- Dim srcRect As New Rectangle(50, 50, 800, 533)
- Dim units As GraphicsUnit = GraphicsUnit.Pixel
- oGraphics.DrawImage(imageFile1, destPara1, srcRect, units)
- Me.PictureBox4.Image = oImage
- End Sub
- Private Sub test3()
- Dim oImage As New Bitmap(500, 360)
- Dim oGraphics As Graphics = Graphics.FromImage(oImage)
- Dim imageFile1 As Image = Image.FromFile("c:/test.jpg")
- ' Create parallelogram for drawing image.
- Dim ulCorner1 As New Point(300, 7)
- Dim urCorner1 As New Point(360, 104)
- Dim llCorner1 As New Point(300, 250)
- Dim destPara1 As Point() = {ulCorner1, urCorner1, llCorner1}
- ' Create rectangle for source image.
- Dim srcRect As New Rectangle(50, 50, 800, 533)
- Dim units As GraphicsUnit = GraphicsUnit.Pixel
- oGraphics.DrawImage(imageFile1, destPara1, srcRect, units)
- Me.PictureBox5.Image = oImage
- End Sub
- Private Sub test4()
- Dim oImage As New Bitmap(500, 360)
- Dim oGraphics As Graphics = Graphics.FromImage(oImage)
- Dim imageFile1 As Image = Image.FromFile("c:/test.jpg")
- ' Create parallelogram for drawing image.
- Dim ulCorner1 As New Point(400, 7)
- Dim urCorner1 As New Point(360, 104)
- Dim llCorner1 As New Point(400, 250)
- Dim destPara1 As Point() = {ulCorner1, urCorner1, llCorner1}
- ' Create rectangle for source image.
- Dim srcRect As New Rectangle(50, 50, 800, 533)
- Dim units As GraphicsUnit = GraphicsUnit.Pixel
- oGraphics.DrawImage(imageFile1, destPara1, srcRect, units)
- Me.PictureBox6.Image = oImage
- End Sub
- Private Sub test5()
- Dim oImage As New Bitmap(500, 360)
- Dim oGraphics As Graphics = Graphics.FromImage(oImage)
- Dim imageFile1 As Image = Image.FromFile("c:/test.jpg")
- ' Create parallelogram for drawing image.
- Dim ulCorner1 As New Point(500, 14)
- Dim urCorner1 As New Point(360, 104)
- Dim llCorner1 As New Point(500, 257)
- Dim destPara1 As Point() = {ulCorner1, urCorner1, llCorner1}
- ' Create rectangle for source image.
- Dim srcRect As New Rectangle(50, 50, 800, 533)
- Dim units As GraphicsUnit = GraphicsUnit.Pixel
- oGraphics.DrawImage(imageFile1, destPara1, srcRect, units)
- Me.PictureBox7.Image = oImage
- End Sub
- Private Sub test6()
- Dim oImage As New Bitmap(500, 360)
- Dim oGraphics As Graphics = Graphics.FromImage(oImage)
- Dim imageFile1 As Image = Image.FromFile("c:/test.jpg")
- ' Create parallelogram for drawing image.
- Dim ulCorner1 As New Point(590, 32)
- Dim urCorner1 As New Point(360, 104)
- Dim llCorner1 As New Point(590, 270)
- Dim destPara1 As Point() = {ulCorner1, urCorner1, llCorner1}
- ' Create rectangle for source image.
- Dim srcRect As New Rectangle(50, 50, 800, 533)
- Dim units As GraphicsUnit = GraphicsUnit.Pixel
- oGraphics.DrawImage(imageFile1, destPara1, srcRect, units)
- Me.PictureBox8.Image = oImage
- End Sub
- Private Sub test7()
- Dim oImage As New Bitmap(800, 360)
- Dim oGraphics As Graphics = Graphics.FromImage(oImage)
- Dim imageFile1 As Image = Image.FromFile("c:/test.jpg")
- ' Create parallelogram for drawing image.
- Dim ulCorner1 As New Point(650, 104)
- Dim urCorner1 As New Point(360, 104)
- Dim llCorner1 As New Point(650, 340)
- Dim destPara1 As Point() = {ulCorner1, urCorner1, llCorner1}
- ' Create rectangle for source image.
- Dim srcRect As New Rectangle(50, 50, 800, 533)
- Dim units As GraphicsUnit = GraphicsUnit.Pixel
- oGraphics.DrawImage(imageFile1, destPara1, srcRect, units)
- Me.PictureBox9.Image = oImage
- Me.PictureBox10.Image = oImage
- End Sub
- Private Sub Button2_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button2.Click
- '-------Button2 events-----------------------------
- 'set counter low for upward count in timer1 _tick event
- count = 0
- PictureBox10.Visible = False
- Timer1.Enabled = True
- End Sub
- Private Sub Button3_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button3.Click
- '-------Button3 events-----------------------------
- 'set counter high for backward count in timer2 _tick event
- 'sets timer2 count1 to 9
- count1 = 10
- '---------Get picturebox2 out of the way as it overlaps onto picturebox10's side
- PictureBox2.Visible = False
- PictureBox10.Visible = True
- Timer2.Enabled = True
- End Sub
- Private Sub Form1_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Load
- '--------Set timer intervals----------------------
- Timer1.Interval = 35
- Timer2.Interval = 35
- '--------Load bitmap into pictureboxes 2-10-------
- test()
- test1()
- test2()
- test3()
- test4() 'Can you get this section to work from within a function????
- test5() 'a variable would be nice to store the image in so we could
- test6() 'call a different image for each flip.
- test7()
- '-------------------------------------------------
- End Sub
Output:
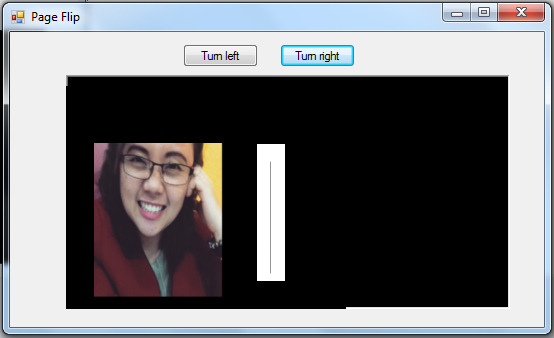
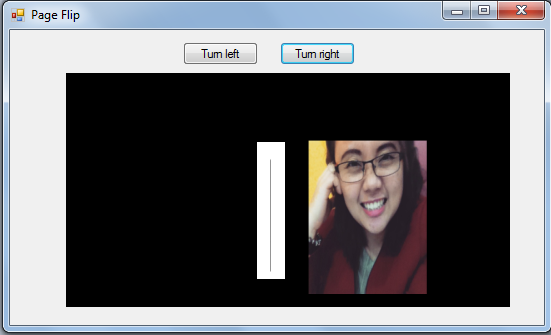
Add new comment
- 299 views