In this tutorial, I’m going to show you how create an application on how to send email messages using Visual Basic.Net. To start with this application, create a new project called “sendEmail”. Then design the form that looks like as shown below.
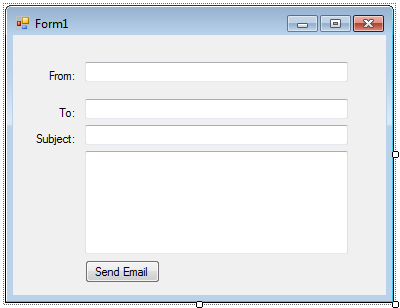
Next, double click the form and add the following code above the public class Form1.
The added code above is a namespace that contains classes used to send electronic mail to a Simple Mail Transfer Protocol(SMTP) server for delivery. After this, double click the button and add the following code.
Dim mail As New MailMessage
'this area for our Sender
mail.From = New MailAddress(txtfrom.Text)
'this area for our Recipient
mail.To.Add(txtto.Text)
'this would the email subject
mail.Subject = txtsubj.Text
'this for the body of our email
mail.Body = txtbody.Text
The code above has a MailMessage class, this class represents the content of a mail message. Under these codes, we will be dealing with another class called SmtpClient that will used to transmit email to SMTP host that you designate for email delivery. And here’s the following codes.
Dim smtp As New SmtpClient("smtp.gmail.com")
'set the EnableSsl to true
smtp.EnableSsl = True
'this area is for your login username and password
smtp.Credentials = New System.Net.NetworkCredential("yourUsername", "yourPassword")
'Gets the port used for SMTP Transactions
smtp.Port = "587"
'finally it sends the message to SMTP server for delivery
smtp.Send(mail)
At this time, we can test our application by pressing the “F5” or Start button. Then it should look like as shown below.
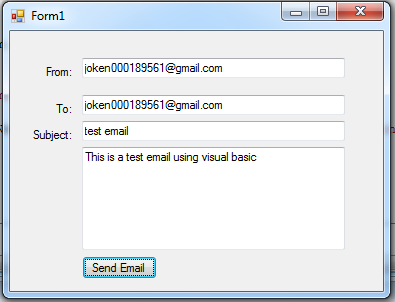
Then after you fill up the following input fields. You can then click the “Send Email” button. Then if you check your Google mail, this will look like as shown below.
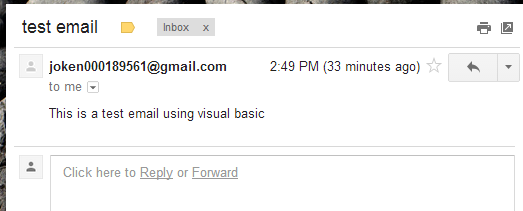
And here's all the code used in this application:
Imports System.Net.Mail
Public Class Form1
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
Dim mail As New MailMessage
'this area for our Sender
mail.From = New MailAddress(txtfrom.Text)
'this area for our Recipient
mail.To.Add(txtto.Text)
'this would the email subject
mail.Subject = txtsubj.Text
'this for the body of our email
mail.Body = txtbody.Text
Dim smtp As New SmtpClient("smtp.gmail.com")
'set the EnableSsl to true
smtp.EnableSsl = True
'this area is for your login username and password
smtp.Credentials = New System.Net.NetworkCredential("yourUsername", "yourPassword")
'Gets the port used for SMTP Transactions
smtp.Port = "587"
'finally it sends the message to SMTP server for delivery
smtp.Send(mail)
End Sub
End Class