Creating a StopWatch in VB.NET
Submitted by donbermoy on Tuesday, April 1, 2014 - 23:20.
Stopwatch is a timepiece that can be started or stopped for exact timing as of a race or any activity. In this article, we will going to create a program that has the capabilities and control like a stopwatch.
Now, let's start this tutorial!
1. Let's start with creating a Windows Form Application for this tutorial by following the following steps in Microsoft Visual Studio: Go to File, click New Project, and choose Windows Application.
2. Next, add four Buttons named Button1 and labeled it as "Start” for starting the time, Button 2 and labeled it as "Stop” for stopping the time, Button3 and labeled it as "Mark” for marking the time, and Button4 and labeled it as "Reset” for resetting the time back to 0. Insert a timer named Timer1 for creating the time, Label named Label1 for displaying the time, and ListView named ListView1 for displaying the marked time. You must design your interface like this:
3. Now put this code for your code module.
Download the source code below and try it! :)
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
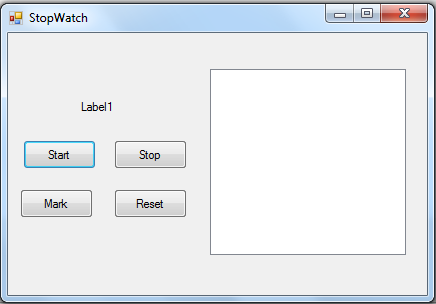
- Public Class Form1
- Dim StopWatch As New Diagnostics.Stopwatch
- Private Sub Timer1_Tick(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Timer1.Tick
- Dim elapsed As TimeSpan = Me.StopWatch.Elapsed
- Label1.Text = String.Format("{0:00}:{1:00}:{2:00}:{3:00}", Math.Floor(elapsed.TotalHours), elapsed.Minutes, elapsed.Seconds, elapsed.Milliseconds)
- End Sub
- Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
- Timer1.Start()
- Me.StopWatch.Start()
- End Sub
- Private Sub Button2_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button2.Click
- Timer1.Stop()
- Me.StopWatch.Stop()
- End Sub
- Private Sub Button4_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button4.Click
- Me.StopWatch.Reset()
- Label1.Text = "00:00:00:000"
- ListBox1.Items.Clear()
- End Sub
- Private Sub Button3_Click_1(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button3.Click
- ListBox1.Items.Add(Label1.Text)
- End Sub
- End Class
Explanation:
We have first initialized variable StopWatch As New Diagnostics.Stopwatch. Stopwatch Class provides a set of methods and properties that you can use to accurately measure elapsed time. Our Button1 is for starting the stopwatch to tick its time. We have initialized variable elapsed As TimeSpan which will be equal to the elapsed time of variable StopWatch. A TimeSpan object represents a time interval (duration of time or elapsed time) that is measured as a positive or negative number of days, hours, minutes, seconds, and fractions of a second. The TimeSpan structure can also be used to represent the time of day, but only if the time is unrelated to a particular date. We used Label1 as the timer that has the format like the real stopwatch. We have the format "{0:00}:{1:00}:{2:00}:{3:00}" for time. Math.Floor Method here returns the largest integer less than or equal to the specified double-precision floating-point number. It does have elapsed time for hours, minutes, seconds, and milliseconds. Our Button2 is used for stopping the time. We stop the timer and the stopwatch variable. Button3 is used for marking the time, meaning saving it to the ListBox. Lastly, Button4 is used for resetting the time back to 0.Output:
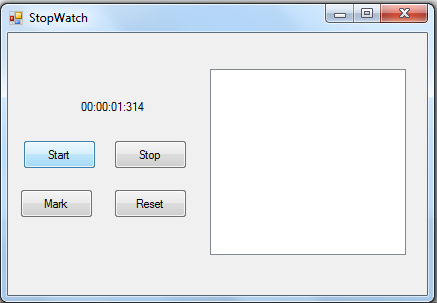
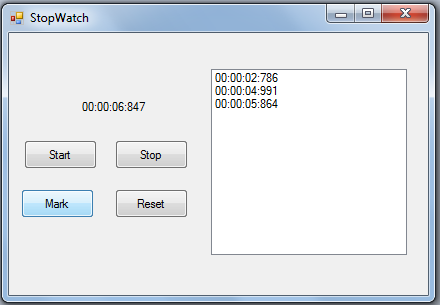
Engr. Lyndon R. Bermoy
IT Instructor/System Developer/Android Developer
Mobile: 09079373999
Telephone: 826-9296
E-mail:[email protected]
Visit and like my page on Facebook at: Bermz ISware Solutions
Subscribe at my YouTube Channel at: SerBermz
Add new comment
- 6686 views