Text to Binary Conversion in VB.NET
Submitted by donbermoy on Sunday, March 2, 2014 - 11:34.
Computers store all characters as numbers stored as binary data. Binary code uses the digits of 0 and 1 (binary numbers) to represent computer instructions or text. Each instruction or symbol gets a bit string assignment. The strings can correspond to instructions, letters, or symbols. In computing, these codes are used for encoding data.
Here in this tutorial, we will create our own program to create a text to binary conversion. :)
So, now let's start this tutorial!
1. Let's start with creating a Windows Form Application for this tutorial by following the following steps in Microsoft Visual Studio: Go to File, click New Project, and choose Windows Application.
2. Next, add two TextBox named TextBox1 to input a text and TextBox2 for displaying the binary conversion of the text you have inputted. Add also a button named Button1. Design your layout like this one below:
3. Put this code to your Button1_Click. This will display the conversion of the text to binary.
Download the source code below and try it! :)
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
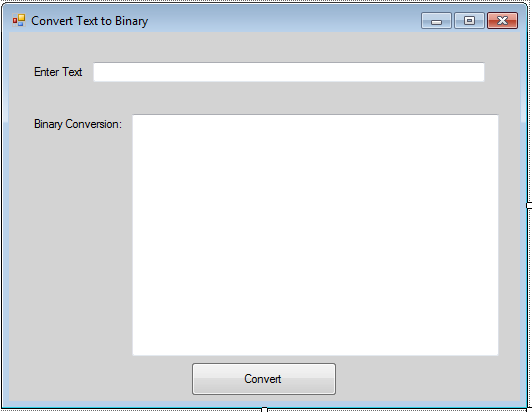
- Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
- Dim Temp As String = ""
- Dim txtBuilder As New System.Text.StringBuilder
- For Each Character As Byte In System.Text.ASCIIEncoding.ASCII.GetBytes(TextBox1.Text)
- txtBuilder.Append(Convert.ToString(Character, 2).PadLeft(8, "0"))
- txtBuilder.Append(" ")
- Next
- Temp = txtBuilder.ToString.Substring(0, txtBuilder.ToString.Length - 0)
- TextBox2.Text = Temp
- End Sub
Explanation:
We initialized Temp as String to nothing, and txtBuilder to instantiate into a StringBuilder. System.Text.StringBuilder represents a string-like object whose value is a mutable sequence of characters. The value is said to be mutable because it can be modified once it has been created by appending, removing, replacing, or inserting characters. Next, we also initialized a variable Character to get the equivalent bytes of the TextBox1. The txtBuilder variable will be appended after the conversion of the Character Bytes to String. The padleft method here returns a new string of a specified length in which the beginning of the current string is padded with spaces or with a specified Unicode character. And this padleft right-aligns the characters. After the For Each Loop, the Temp variable will have a value of the TextBuilder with its specified length. Next, all the conversion process holds the Temp Variable and it will be equal and displayed in TextBox2. Output: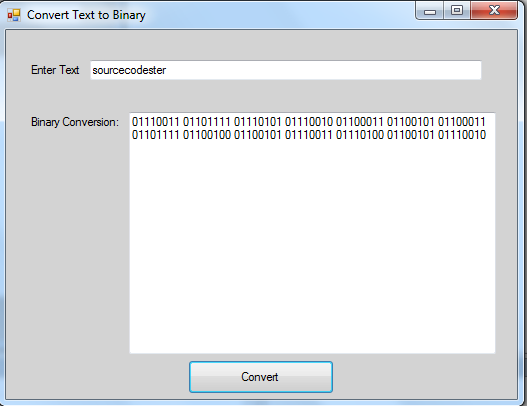
Engr. Lyndon R. Bermoy
IT Instructor/System Developer/Android Developer
Mobile: 09079373999
Telephone: 826-9296
E-mail:[email protected]
Visit and like my page on Facebook at: Bermz ISware Solutions
Subscribe at my YouTube Channel at: SerBermz
Add new comment
- 1502 views