Stock Inventory System: Creating the Stock Master
Submitted by joken on Monday, November 11, 2013 - 21:14.
In this tutorial, I’m going to show you how to create a stock master. This stock master will cater all the data entry for the new acquired stocks or Item(s). And the loading, updating and deleting of a specific stocks or Items. To start in this lesson, first we need to open our project called “Stockinven”. Then, were going to add new form and we will name it as “Stock Master”. To do this, go to solution explorer right click the project name, then point the mouse pointer to “Add” then choose “Windows Form”. And it looks like as shown below:
After creating the form for Stock Master, you need to set the Name Property of all then design it, and the stock master will looks like as shown below.
To test this application, we need to set first the Stock Master to be the first form to load when we run the program. To do this, here are the following steps:
1. On the menu bar, click the Project.
2. And select Stockinven Properties.
3. Then the properties of stockinven will show.
4. Next, click the ApplicationTabbed.
5. Then on the Startup form.
6. Choose Stock Master.
7. Then press “F5” to test your program.
At this time, we will be going to manually populate the following combobox.
For color, here are the items:
Then, were we going to copy some for database connectivity from form1. And we will paste the declaration of variables below the public class and the connection string inside the Stock_Master_load sub. And the code we now look like as shown below:
This time, double click the save button and add the following code:
The code below will do the insertion of data inputted bby the user and store it into the database.
After adding the code above, press “F5” to test the application. then fill up all the fields provided, then click the save button. If successfully save, a message box will appear. And the output now will look like as shown below:
And my next lesson will focus on loading, updating and deleting of an item.
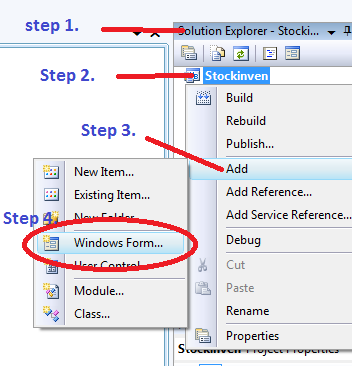
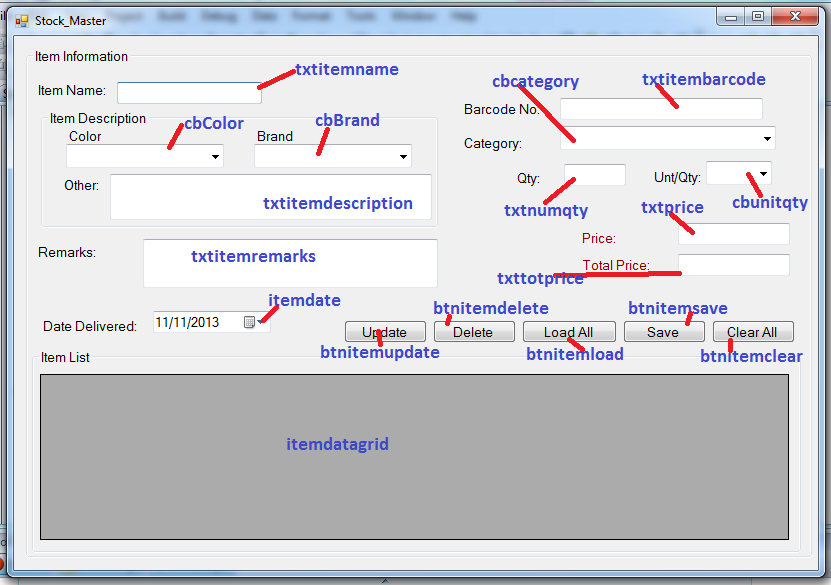
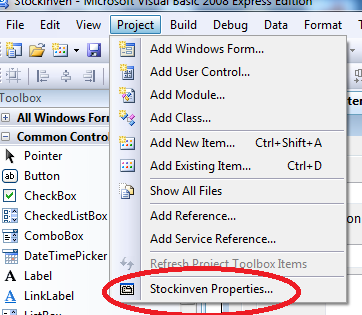
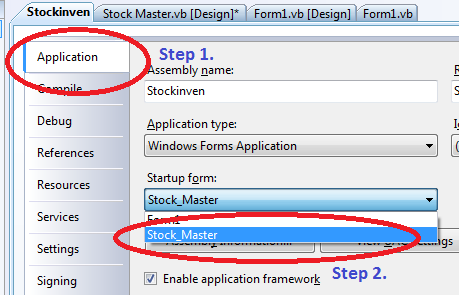
Black,White,Red,Blue,Green,Violet,Brown,Purple
For bbrand here are the items:
Toshiba,Samsung,Dell,Sony,Polytron,Acer,Labtech,Red Fox
For Category, Here are the following items:
Computer parts, School supplies, Consumables, Books
And for Unit and quantity:
Set, Kilograms, Grams, Yard, Piece, Box
Next, let’s start adding code to our application. First Double click the txtprice and add the following code:
This code will activate when you input some value to the txtprice. And it will be computted if the input is numeric else it will be reset to nothing.
- 'it check if the value of txtprice is not numeric
- txtprice.Text = ""
- Else
- Dim total As Double
- 'it computes for total price
- 'it passed the value to txttotprice
- End If
- Public Class Stock_Master
- Dim con As New OleDb.OleDbConnection
- Private Sub Stock_Master_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
- con.ConnectionString = "Provider=Microsoft.Jet.OLEDB.4.0;Data Source=" & Application.StartupPath & "\stocksdb.mdb"
- End Sub
- Private Sub txtprice_TextChanged(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles txtprice.TextChanged
- 'it check if the value of txtprice is not numeric
- txtprice.Text = ""
- Else
- Dim total As Double
- 'it computes for total price
- 'it passed the value to txttotprice
- End If
- End Sub
- End Class
- Dim result As Integer
- 'we declare cmd to represent an SQL Statements or a stored procedure
- 'to execute against a data source
- Dim cmd As New OleDb.OleDbCommand
- 'gather all information for item description
- Dim a As String
- a = "Color: " & cbColor.SelectedItem & " " & "Brand: " & cbBrand.SelectedItem & " " & "Other Desc: " & txtitemdescription.Text
- Try
- Dim sql As String
- 'we set up an sql statement intended for inserting a data
- sql = "INSERT INTO tblitemmaster(itemname, itemdescription, " & _
- " itemremarks, itembarcodeno, itemcategory, itemnoqty,itemavailqty, " & _
- " itemqty, itempricce, itemtotalprice, dateencoded) " & _
- " VALUES('" & txtitemname.Text & "'," & _
- "'" & a & "','" & txtitemremarks.Text & "'," & _
- "'" & txtitembarcode.Text & "','" & cbcategory.SelectedValue & "'," & _
- "'" & txtnumqty.Text & "','" & txtnumqty.Text & "','" & cbunitqty.SelectedValue & "'," & _
- 'open the connection
- con.Open()
- With cmd
- .Connection = con
- .CommandText = sql
- 'execute query and return the affected rows
- result = cmd.ExecuteNonQuery
- 'check if result is zero it means that no data has inserted
- If result = 0 Then
- Else
- 'else greater than one it insert the data successfully
- End If
- End With
- Catch ex As Exception
- 'if something went wrong, it will give a message about the error
- End Try
- 'close the connection
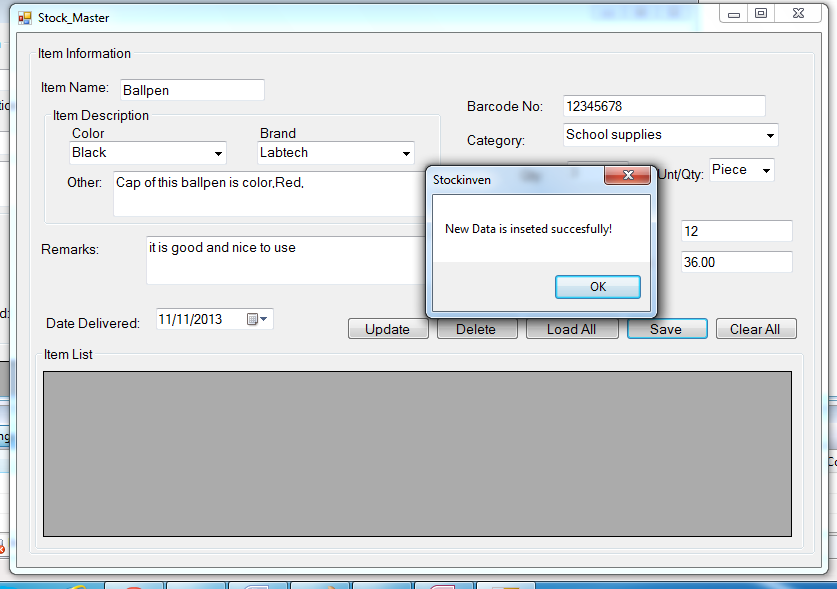