In creating a modular program using Visual Basic.Net we always used Procedures and Functions. A procedure and function is a piece of code in larger program. They perform a specific task. Reducing duplication of code, Decomposing complex problems into simpler pieces, Improving of code, Reuse of code, and Information hiding are the common advantage of using procedures and functions.
Procedures
A procedure does not return any value and the statements is enclosed inside the Sub and End Sub.
Example:
Public Class testsub
Private Sub Button3_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button3.Click
Dim email As String
'Get the value from textbox named txteamil
'and stored to string variable email
email = Txtemail.Text
'call the sub procedure named checkemel
Call checkemel(email)
End Sub
'this is our Procedure
Sub checkemel(ByVal email As String)
'test if the user dont e something on the textbox
If email = "" Then
'if true then it will ask the user to write the email address
MsgBox("Please Provide correct email address!")
Else
'else it simply display the value inputed by the user
MsgBox("Your email address is!" & email
)
End If
End Sub
End Class
Output
Functions
A function does something returns a value and using Visual Basic the statements inside Function, End Function.
Example:
Public Class testFunctions
Private Sub Button6_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button6.Click
Dim first As Integer
Dim second As Integer
Dim result As Integer
'assign values to specified variables
'calls the function to perform addition
result = AddTwoNumbers(first, second)
If result = 0 Then
Else
MsgBox("The answer is " & result
)
End If
End Sub
Function AddTwoNumbers(ByVal firsts As Integer, ByVal seconds As Integer) As Integer
Dim answer As Integer
answer = firsts + seconds
'AddTwoNumbers = answer
Return answer
End Function
End Class
Output
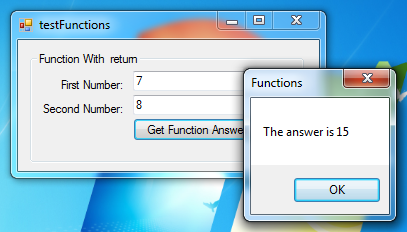