Part I: Creating and Reading of MySQL Data Using OOP Approach in PHP
Submitted by GeePee on Friday, April 10, 2015 - 22:33.
This tutorial I will show you on how to perform Object Oriented Programming using PHP and how easy to manage our source code and deal with objects and update the code easily. Before we move on to writing object-oriented code in PHP, it is important to understand the basic concept of classes, objects, properties, and methods.
A Class is like a blueprint of a car. It defines how car created from the blueprint will look and behave, but it still an abstract concept.
An Object is like a real car created from the blueprint. It has a real properties (such as how fast it’s going on), and real behaviors (like “accelerate” and “brake”).
An object properties are closely tied to the object. Although all objects created from a given class have the same properties, one object properties can have different values of other object properties.
A Method is simply a function that is defined inside the class is a method and used as an object. Where they can contain many local variables, and they can return values.
An object is habitually assumed to be an Instance of a class, and the process of creating an object from a class is called Instantiation.
Here’s the final output looks like for this tutorial.
Now let’s begin the tutorial, this course contains this directory with PHP files.
This time we're going to start our coding for config.php, database.php, functions.php, initialize.php and user.php.
The config.php file will handle the settings for server, user, password and the database to be used for example I will used "jokendb". And here’s the code.
Next the function.php.
Next for initialize.php.
And lastly the user.php class.
And for our header.php this is the code.
And we need to add this code for index.php.
This time when the [New Entry] is clicked, it will call the create_user.php.
That's it for now folks you can download the full source code together with images and css file included in this topic. My next will be the continuation of this topic that will tackle the editing and updating of user.
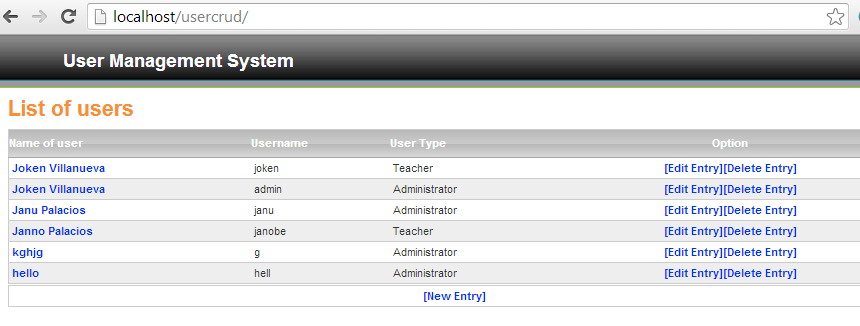
Usercrud images includes config.php database.php functions.php initialize.php user.php layouts Header.php stylesheets Protect.css create_user.php index.phpOur database to be used:
- CREATE TABLE IF NOT EXISTS `users` (
- `user_id` INT(11) NOT NULL AUTO_INCREMENT,
- `users_name` VARCHAR(30) NOT NULL,
- `uname` VARCHAR(30) NOT NULL,
- `u_pass` VARCHAR(60) NOT NULL,
- `utype` VARCHAR(30) NOT NULL,
- PRIMARY KEY (`user_id`)
- ) ENGINE=MyISAM DEFAULT CHARSET=latin1 AUTO_INCREMENT=1234571 ;
For our database.php class.
- <?php
- /**
- * Description: The main class for Database.
- * Author: Joken Villanueva
- * Date Created: May 18, 2013
- * Revised By:
- */
- require_once(LIB_PATH.DS."config.php");
- class Database {
- var $sql_string = '';
- var $error_no = 0;
- var $error_msg = '';
- private $conn;
- public $last_query;
- private $magic_quotes_active;
- private $real_escape_string_exists;
- function __construct() {
- $this->open_connection();
- }
- //openning the connection
- public function open_connection() {
- if(!$this->conn){
- echo "Problem in database connection! Contact administrator!";
- }else{
- if (!$db_select) {
- echo "Problem in selecting database! Contact administrator!";
- }
- }
- }
- function setQuery($sql='') {
- $this->sql_string=$sql;
- }
- function executeQuery() {
- $this->confirm_query($result);
- return $result;
- }
- private function confirm_query($result) {
- if(!$result){
- return false;
- }
- return $result;
- }
- function loadResultList( $key='' ) {
- $cur = $this->executeQuery();
- if ($key) {
- $array[$row->$key] = $row;
- } else {
- $array[] = $row;
- }
- }
- return $array;
- }
- function loadSingleResult() {
- $cur = $this->executeQuery();
- $data = $row;
- }
- return $data;
- }
- function getFieldsOnOneTable( $tbl_name ) {
- $this->setQuery("DESC ".$tbl_name);
- $rows = $this->loadResultList();
- $f[] = $rows[$x]->Field;
- }
- return $f;
- }
- public function fetch_array($result) {
- }
- //gets the number or rows
- public function num_rows($result_set) {
- }
- public function insert_id() {
- // get the last id inserted over the current db connection
- }
- public function affected_rows() {
- }
- public function escape_value( $value ) {
- if( $this->real_escape_string_exists ) { // PHP v4.3.0 or higher
- // undo any magic quote effects so mysql_real_escape_string can do the work
- } else { // before PHP v4.3.0
- // if magic quotes aren't already on then add slashes manually
- // if magic quotes are active, then the slashes already exist
- }
- return $value;
- }
- //closing of connection
- public function close_connection() {
- }
- }
- }
- $mydb = new Database();
- ?>
- <?php
- function strip_zeros_from_date($marked_string="") {
- //first remove the marked zeros
- return $cleaned_string;
- }
- function redirect_to($location = NULL) {
- if($location != NULL){
- exit;
- }
- }
- function output_message($message="") {
- return "<p class=\"message\">{$message}</p>";
- }else{
- return "";
- }
- }
- //this function will autoload our classess available
- function __autoload($class_name) {
- $path = LIB_PATH.DS."{$class_name}.php";
- require_once($path);
- }else{
- }
- }
- function include_layout_template_public($template="") {
- include(SITE_ROOT.DS.'layouts'.DS.$template);
- }
- ?>
- <?php
- /**
- * Description: This includes for basic and core configurations.
- * Author: Joken Villanueva
- * Date Created: May 19, 2013
- * Revised By:
- */
- //define the core paths
- //Define them as absolute peths to make sure that require_once works as expected
- //DIRECTORY_SEPARATOR is a PHP Pre-defined constants:
- //(\ for windows, / for Unix)
- // load config file first
- require_once(LIB_PATH.DS."config.php");
- //load basic functions next so that everything after can use them
- require_once(LIB_PATH.DS."functions.php");
- require_once(LIB_PATH.DS."database.php");
- ?>
- <?php
- /**
- * Description: user class.
- * Return list of users, Single user.
- * Author: Joken E. Villanueva
- * Date Created: May 24,2013
- * Date Modified:June 8, 2013
- */
- require_once(LIB_PATH.DS.'database.php');
- class User {
- protected static $tbl_name = "users";
- /*-Comon SQL Queries-*/
- function db_fields(){
- global $mydb;
- return $mydb->getFieldsOnOneTable(self::$tbl_name);
- }
- function allUsers(){
- global $mydb;
- $mydb->setQuery("SELECT * FROM users");
- $cur = $mydb->loadResultList();
- return $cur;
- }
- function userFilter($id=0,$uname=''){
- global $mydb;
- $mydb->setQuery("SELECT * FROM ".self::$tbl_name." Where user_id= {$id} OR uname= {$uname}");
- $cur = $mydb->loadResultList();
- return $cur;
- }
- function singleUser($id=0){
- global $mydb;
- $mydb->setQuery("SELECT * FROM ".self::$tbl_name." WHERE user_id={$id} LIMIT 1");
- $row = $mydb->loadSingleResult();
- return $row;
- }
- static function Authenticate($uname="", $h_upass=""){
- global $mydb;
- $mydb->setQuery("SELECT * FROM `users` WHERE `uname`='". $uname ."' and `u_pass`='". $h_upass ."'");
- $rows = $mydb->loadSingleResult();
- $_SESSION['user_id'] = $rows->user_id;
- $_SESSION['username'] = $rows->users_name;
- $_SESSION['usersname'] = $rows->uname;
- $_SESSION['userpass'] = $rows->u_pass;
- $_SESSION['usertype'] = $rows->utype;
- return true;
- }
- /*---Instantiation of Object dynamically---*/
- static function instantiate($record) {
- $object = new self;
- foreach($record as $attribute=>$value){
- if($object->has_attribute($attribute)) {
- $object->$attribute = $value;
- }
- }
- return $object;
- }
- /*--Cleaning the raw data before submitting to Database--*/
- private function has_attribute($attribute) {
- // We don't care about the value, we just want to know if the key exists
- // Will return true or false
- }
- protected function attributes() {
- // return an array of attribute names and their values
- global $mydb;
- foreach($this->db_fields() as $field) {
- if(property_exists($this, $field)) {
- $attributes[$field] = $this->$field;
- }
- }
- return $attributes;
- }
- protected function sanitized_attributes() {
- global $mydb;
- // sanitize the values before submitting
- // Note: does not alter the actual value of each attribute
- foreach($this->attributes() as $key => $value){
- $clean_attributes[$key] = $mydb->escape_value($value);
- }
- return $clean_attributes;
- }
- /*--Create,Update and Delete methods--*/
- public function save() {
- // A new record won't have an id yet.
- }
- public function create() {
- global $mydb;
- // Don't forget your SQL syntax and good habits:
- // - INSERT INTO table (key, key) VALUES ('value', 'value')
- // - single-quotes around all values
- // - escape all values to prevent SQL injection
- $attributes = $this->sanitized_attributes();
- $sql = "INSERT INTO ".self::$tbl_name." (";
- $sql .= ") VALUES ('";
- $sql .= "')";
- echo $mydb->setQuery($sql);
- if($mydb->executeQuery()) {
- $this->id = $mydb->insert_id();
- return true;
- } else {
- return false;
- }
- }
- public function update($id=0) {
- global $mydb;
- $attributes = $this->sanitized_attributes();
- foreach($attributes as $key => $value) {
- $attribute_pairs[] = "{$key}='{$value}'";
- }
- $sql = "UPDATE ".self::$tbl_name." SET ";
- $sql .= " WHERE user_id=". $id;
- $mydb->setQuery($sql);
- if(!$mydb->executeQuery()) return false;
- }
- public function delete($id=0) {
- global $mydb;
- $sql = "DELETE FROM ".self::$tbl_name;
- $sql .= " WHERE user_id=". $id;
- $sql .= " LIMIT 1 ";
- $mydb->setQuery($sql);
- if(!$mydb->executeQuery()) return false;
- }
- }
- ?>
- <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
- <html xmlns="http://www.w3.org/1999/xhtml">
- <head>
- <meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
- <title>User Information Management System</title>
- <script type="text/javascript" src="dropdown/dropdown.js"></script>
- </head>
- <body>
- <div id="titlebar_m">
- <div id="titlebar_l">
- <div id="titlebar_r">
- <table border="0" cellpadding="0" cellspacing="0" width="100%" id="titlebar_frame">
- <tr>
- <td width="50%" align="left" id="system-title">User Management System</td>
- <td align="right">
- <table border="0" cellpadding="0" cellspacing="0" 'id="welcome-and-logout"'>
- <tr>
- <td id="welcome-user"></strong></td>
- <td width="30"> </td>
- <td>
- </td>
- </tr>
- </table>
- </td>
- </tr>
- </table>
- </div>
- </div>
- </div>
- <link rel="stylesheet" type="text/css" href="javascripts/tools/calendar/css/calendar-win2k-cold-1.css" />
- <script type="text/javascript" language="javascript" src="javascripts/application.js"></script>
- <script type="text/javascript" language="javascript" src="javascripts/tools/calendar/calendar.js"></script>
- <script type="text/javascript" language="javascript" src="javascripts/tools/calendar/lang/calendar-en.js"></script>
- <script type="text/javascript" language="javascript" src="javascripts/tools/calendar/calendar-setup.js"></script>
- <script type="text/javascript" language="javascript" src="javascripts/tools/monthpicker/monthpicker.js"></script>
- <?php
- /**
- * Description: Listing of user.
- * Author: Joken E. Villanueva
- * Date Created: May 24,2013
- * Date Modified:June 6, 2013
- */
- require_once("includes/initialize.php");
- include_layout_template_public('header.php');
- ?>
- <div id="module-name">List of users
- </div>
- <div id="content">
- <Table class="app_listing" style="width:100%;">
- <tr>
- <th align="left">Name of user</th>
- <th align="left">Username</th>
- <th align="left">User Type</th>
- <th align="center">Option</th>
- </tr>
- <?php
- //it declare $user as new User class
- //$user->allUser it simply calls the allUser() function that will return all user found in the database table
- $user = new User();
- $cur = $user->allUsers();
- //this loadObject() is a function where we loop all the return user by $user->allUsers() function.
- loadObject();
- function loadObject(){
- global $cur;
- foreach ($cur as $object){
- echo (@$odd == true) ? ' <tr class="odd_row" > ' : ' <tr class="even_row"> ';
- @$odd = !$odd;
- echo ' <td> ';
- echo '<a href="#.php'" class="app_listitem_key">'.$object->users_name.'</a>';
- echo ' <td> ';
- echo $object->uname;
- echo ' <td> ';
- echo $object->utype;
- echo ' <td align="center"> ';
- echo '<a href="#.php" class="app_listitem_key">[Edit Entry]</a>';
- echo '<a href="#.php'" class="app_listitem_key">[Delete Entry]</a>';
- }
- }
- ?>
- </table>
- <table class="app_listing" style="width:100%;">
- <tr align="center">
- <td>
- <a href="create_user.php" class="app_listitem_key">[New Entry]</a>
- </td>
- </tr>
- </table>
- <?php
- /**
- * Description: Create user.
- * Author: Joken E. Villanueva
- * Date Created: May 24,2013
- * Date Modified:June 6, 2013
- */
- require_once("includes/initialize.php");
- include_layout_template_public('header.php');
- ?>
- <div id="module-name">New User
- </div>
- <?php
- //it check if submit button is set
- //form has been submitted1
- if($name == ''){
- echo 'Name is not Valid!';
- exit;
- }elseif($uname == ''){
- echo 'Username is not Valid!';
- exit;
- }elseif($upass == ''){
- echo 'Password is not Valid!';
- exit;
- }else{
- //instantiation of objects
- $user = new User();
- $user->users_name = $name;
- $user->uname = $uname;
- $user->utype = $utype;
- //it save the data and if successfully saved it redirected back into index.php
- $istrue = $user->create();
- if($istrue){?>
- <script type="text/javascript">
- alert("New user has successfully added!");
- window.location = "index.php";
- </script>
- <?php
- }else{
- echo "Inserting Failed!";
- }
- }
- }else{
- $name = "";
- $uname = "";
- $upass = "";
- $utype = "";
- }
- ?>
- <div id="content">
- <form method="post" action="create_user.php">
- <table class="app_listing" width="50%">
- <tr>
- <th > <div class="app_title" align="left"> User Details</div></th>
- </tr>
- <tr class="form">
- <td class="form">
- <table class="app_form">
- <tr>
- <td class="label" width="120">Name :: </td>
- <td >
- <input type="text" name="user_name" id="user_name" class="txtbox" />
- </td>
- </tr>
- <tr>
- <td class="label">Username :: </td>
- <td>
- <input type="text" name="uname" id="uname" class="txtbox" />
- </td>
- </tr>
- <tr>
- <td class="label">Password :: </td>
- <td>
- <input type="password" name="upass" id="upass" class="txtbox" />
- </td>
- </tr>
- <tr>
- <td class="label">User Type :: </td>
- <td>
- <select name="utype" id="utype" >
- <option value="Administrator">Administrator</option>
- <option value="Registrar">Registrar</option>
- <option value="Teacher">Teacher</option>
- <option value="Student">Student</option>
- </select>
- </td>
- </tr>
- <tr>
- <td class="label"></td>
- <td>
- <input type="submit" name="submit" value="Save" class="app_button">
- </td>
- </tr>
- </table>
- </tr>
- </table>
- </form>
Add new comment
- 141 views