PHP - Form File Upload Using AngularJS
Submitted by razormist on Saturday, September 28, 2019 - 09:49.
In this tutorial we will create a Form File Upload Using AngularJS. This code will upload a file to the database server when user submit the form inputs. The code use angular directives to upload file that will be process and send a POST request that will store the file to the database server using MySQLi INSERT query. This a user-friendly program feel free to modify and use it to your system.
We will be using AngularJS as a framework which has additional custom HTML attributes embedded into it. It can interprets those attributes as directives to bind inputted parts of the page to a model that represent as a standard JavaScript variables.
script.js
Note: Make sure you save this file inside the js directory in order the script works.
There you have it we successfully created a Form File Upload using AngularJS. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!!
Getting Started:
First you have to download & install XAMPP or any local server that run PHP scripts. Here's the link for XAMPP server https://www.apachefriends.org/index.html. And this is the link for the bootstrap that i used for the layout design https://getbootstrap.com/. Lastly, this is the link for the AngularJS https://angularjs.org/.Creating Database
Open your database web server then create a database name in it db_file_angular, after that click Import then locate the database file inside the folder of the application then click ok.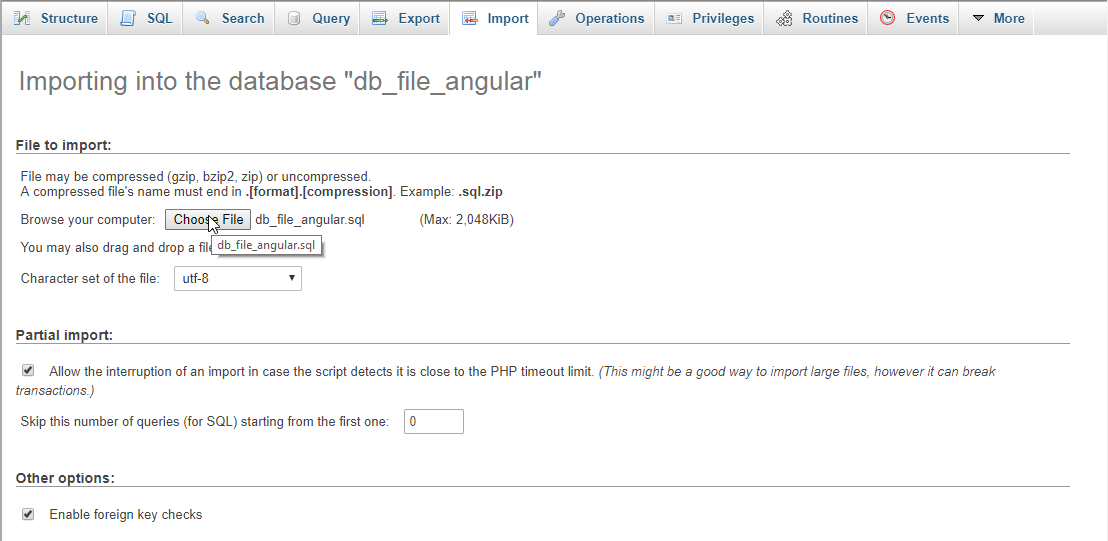
Creating the database connection
Open your any kind of text editor(notepad++, etc..). Then just copy/paste the code below then name it conn.php.- <?php
- if(!$conn){
- }
- ?>
Creating The Interface
This is where we will create a simple form for our application. To create the forms simply copy and write it into you text editor, then save it index.php.- <!DOCTYPE html>
- <html ng-app="myModule" >
- <head>
- <meta charset = "UTF-8" name = "viewport" content = "width=device-width, initial-scale=1"/>
- <link rel = "stylesheet" type = "text/css" href = "css/bootstrap.css"/>
- </head>
- <body ng-controller="myController">
- <nav class = "navbar navbar-default">
- <div class = "container-fluid">
- <a class = "navbar-brand" href = "https://sourcecodester.com">Sourcecodester</a>
- </div>
- </nav>
- <div class = "col-md-3"></div>
- <div class = "col-md-6 well">
- <h3 class = "text-primary">PHP - Form File Upload Using AngularJS</h3>
- <hr style = "border-top:1px dotted #000;"/>
- <div class="col-md-4">
- <form ng-submit="submit()" name="form" role="form">
- <div class = "form-group">
- <input ng-model="file" class="form-controller" type="file" class="form-control" onchange="angular.element(this).scope().uploadedFile(this)">
- </div>
- <center><button class = "btn btn-primary"><span class = "glyphicon glyphicon-upload"></span> Upload</button></center>
- </form>
- </div>
- <div class="col-md-8">
- <table class="table table-bordered">
- <thead class="alert-info">
- <tr>
- <th>File Name</th>
- <th>File Location</th>
- </tr>
- <thead>
- <tbody>
- <?php
- require 'conn.php';
- echo"<tr>
- <td>".$fetch['filename']."</td>
- <td>".$fetch['location']."</td>
- </tr>";
- }
- ?>
- </tbody>
- </table>
- </div>
- </div>
- </body>
- <script src="js/angular.js"></script>
- <script src = "js/script.js"></script>
- </html>
Creating the Main Function
This code contains the main function of the application. This code will upload a file to the database server when the button is clicked. To do this just copy and write these block of codes as shown below inside the text editor and save it as shown below. upload.php- <?php
- require_once 'conn.php';
- $file_name = $_FILES['file']['name'];
- $file_temp = $_FILES['file']['tmp_name'];
- $file_size = $_FILES['file']['size'];
- $location = "uploads/".$file;
- if($file_size < 5242880){
- echo "Successfully uploaded!";
- }else{
- echo "File too large too upload!";
- }
- }else{
- echo "Please upload file first!";
- }
- ?>
- var app = angular.module('myModule',[]);
- app.controller('myController', function($scope, $http) {
- $scope.form = [];
- $scope.files = [];
- $scope.uploadedFile = function(element) {
- $scope.currentFile = element.files[0];
- var reader = new FileReader();
- reader.onload = function(event) {
- $scope.$apply(function($scope) {
- $scope.files = element.files;
- });
- }
- reader.readAsDataURL(element.files[0]);
- }
- $scope.submit = function() {
- $scope.form.file = $scope.files[0];
- $http({
- method : 'POST',
- url : 'upload.php',
- processData: false,
- transformRequest: function (data) {
- var formData = new FormData();
- formData.append("file", $scope.form.file);
- return formData;
- },
- data : $scope.form,
- headers: {
- 'Content-Type': undefined
- }
- }).then(function(data){
- alert(data.data);
- window.location="index.php";
- });
- };
- });
Add new comment
- 233 views