PHP - Simple Display Data On Current Date
Submitted by razormist on Sunday, May 12, 2019 - 06:55.
In this tutorial we will create a Simple Display Data On Current Date using PHP. This code can display a data base on the given date when the user submit the form data. The code use MySQLi SELECT query to retrieve the data in a current day when you add WHERE clause in which it contrast the date value. This a user-friendly program feel free to modify and use it to your system.
We will be using PHP as a scripting language that interpret in the webserver such as xamp, wamp, etc. It is widely use by modern website application to handle and protect user confidential information.
There you have it we successfully created Simple Display Data On Current Date using PHP. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
Getting Started:
First you have to download & install XAMPP or any local server that run PHP scripts. Here's the link for XAMPP server https://www.apachefriends.org/index.html. And, this is the link for the bootstrap that i used for the layout design https://getbootstrap.com/.Creating Database
Open your database web server then create a database name in it db_display, after that click Import then locate the database file inside the folder of the application then click ok.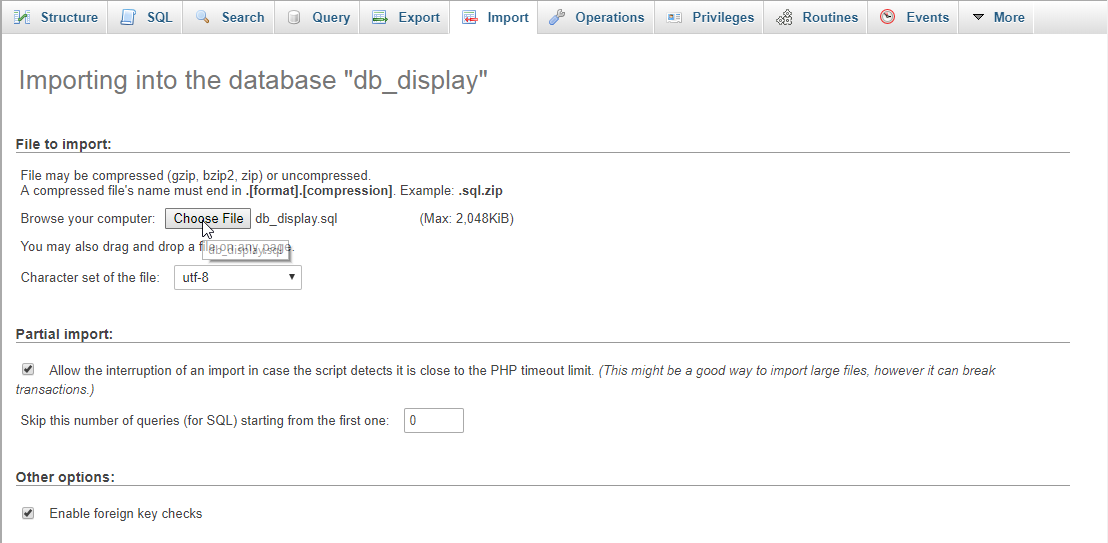
Creating the database connection
Open your any kind of text editor(notepad++, etc..). Then just copy/paste the code below then name it conn.php.- <?php
- if(!$conn){
- }
- ?>
Creating The Interface
This is where we will create a simple form for our application. To create the forms simply copy and write it into your text editor, then save it as index.php.- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css"/>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="continer-fluid">
- <a class="navbar-brand" href="https://sourcecodester.com">Sourcecodester</a>
- </div>
- </nav>
- <div class="col-md-3"></div>
- <div class="col-md-6 well">
- <h3 class="text-primary">PHP - Simple Display Data On Current Date</h3>
- <hr style="border-top:1px dotted #ccc;"/>
- <form method="POST" action="insert.php">
- <div class="form-inline">
- <input type="text" name="product_name" class="form-control" placeholder="Product Name" required="required"/>
- <input type="date" name="date_purchase" class="form-control" placeholder="Date Purchase" required="required"/>
- <button class="btn btn-primary" name="insert">Insert<//button>
- </div>
- </form>
- <br />
- <div class="col-md-3"></div>
- <div class="col-md-6">
- <form method="POST" action="">
- <button class="btn btn-success" name="all">Display All</button>
- <button class="btn btn-warning" name="current">Display Current</button>
- </form>
- <br /><br />
- <table class="table table-bordered">
- <thead class="alert-info">
- <tr>
- <th>Product Name</th>
- <th>Date Purchase</th>
- </tr>
- </thead>
- <?php include'filter.php'?>
- </table>
- </div>
- </div>
- </body>
- </html>
Creating the PHP Query
This code contains the query of the application. This code will store the data inputs to the database server. To do this just copy and write these block of codes below inside the text editor, then save it as insert.php.- <?php
- require_once'conn.php';
- $product_name=$_POST['product_name'];
- $date_purchase=$_POST['date_purchase'];
- mysqli_query($conn, "INSERT INTO `product` VALUES('', '$product_name', '$date_purchase')") or die(mysqli_error());
- }
- ?>
Creating the Main Function
This code contains the main function of the application. This code will display the data on the current date. To make this just copy and write these block of codes below inside the text editor, then save it as filter.php.- <?php
- ?>
- <tbody>
- <?php
- require'conn.php';
- ?>
- <tr>
- <td><?php echo $fetch['product_name']?></td>
- <td><?php echo $fetch['date_purchase']?></td>
- </tr>
- <?php
- }
- ?>
- </tbody>
- <?php
- ?>
- <tbody>
- <?php
- require'conn.php';
- $query=mysqli_query($conn, "SELECT * FROM `product` WHERE `date_purchase`='$date'") or die(mysqli_error());
- ?>
- <tr>
- <td><?php echo $fetch['product_name']?></td>
- <td><?php echo $fetch['date_purchase']?></td>
- </tr>
- <?php
- }
- ?>
- </tbody>
- <?php
- }else{
- ?>
- <tbody>
- <?php
- require'conn.php';
- ?>
- <tr>
- <td><?php echo $fetch['product_name']?></td>
- <td><?php echo $fetch['date_purchase']?></td>
- </tr>
- <?php
- }
- ?>
- </tbody>
- <?php
- }
- ?>