PHP - Submit POST Data to MySQLi Using AngularJS
Submitted by razormist on Friday, May 10, 2019 - 18:36.
In this tutorial we will create a Submit POST Data to MySQLi Using AngularJS. This code will submit the POST data when the user click the submit button. The code use angular directives to submit the process form data in order to send a POST request to the MySQLi server. This a user-friendly program feel free to modify and use it to your system.
We will be using AngularJS as a framework which has additional custom HTML attributes embedded into it. It can interprets those attributes as directives to bind inputted parts of the page to a model that represent as a standard JavaScript variables.
data.php
script.js
Note: Make sure you save this file inside the js directory in order the script works.
There you have it we successfully created a Submit POST Data to MySQLi using AngularJS. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!!
Getting Started:
First you have to download & install XAMPP or any local server that run PHP scripts. Here's the link for XAMPP server https://www.apachefriends.org/index.html. And this is the link for the bootstrap that i used for the layout design https://getbootstrap.com/. Lastly, this is the link for the AngularJS https://angularjs.org/.Creating Database
Open your database web server then create a database name in it db_submit, after that click Import then locate the database file inside the folder of the application then click ok.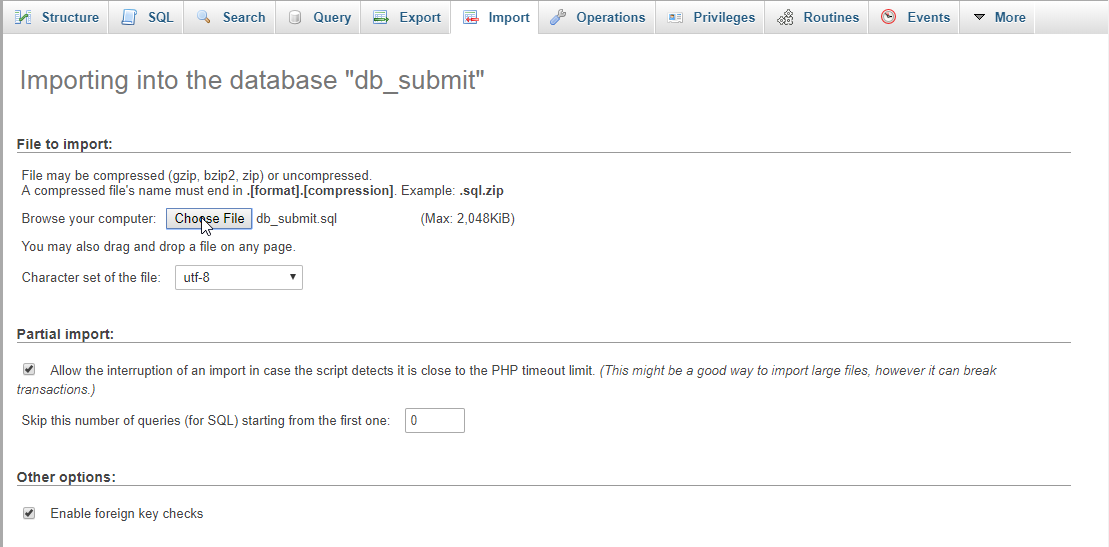
Creating the database connection
Open your any kind of text editor(notepad++, etc..). Then just copy/paste the code below then name it conn.php.- <?php
- if(!$conn){
- }
- ?>
Creating The Interface
This is where we will create a simple form for our application. To create the forms simply copy and write it into you text editor, then save it index.php.- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- </head>
- <body ng-app="myModule" ng-controller="myController">
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- <a class="navbar-brand" href="https://sourcecodester.com" >Sourcecodester</a>
- </div>
- </nav>
- <div class="col-md-3"></div>
- <div class="col-md-6 well">
- <h3 class="text-primary">PHP - Submit POST Data to MySQLi Using AngularJS</h3>
- <hr style="border-top:1px dotted #ccc;">
- <div class="col-md-4">
- <form>
- <div class="form-group">
- <label>Firstname</label>
- <input type="text" class="form-control" ng-model="firstname"/>
- </div>
- <div class="form-group">
- <label>Lastname</label>
- <input type="text" class="form-control" ng-model="lastname"/>
- </div>
- <div class="form-group">
- <label>Address</label>
- <input type="text" class="form-control" ng-model="address"/>
- </div>
- <center><button type = "button" class = "btn btn-primary" ng-click = "saveData()" ><span class = "glyphicon glyphicon-save"></span> Save</button></center>
- </form>
- </div>
- <div class="col-md-8">
- <table class = "table table-bordered">
- <thead class="alert-success">
- <tr>
- <th>Firstname</th>
- <th>Lastname</th>
- <th>Address</th>
- </tr>
- </thead>
- <tbody style="background-color:#fff;">
- <tr ng-repeat = "member in members">
- <td>{{member.firstname}}</td>
- <td>{{member.lastname}}</td>
- <td>{{member.address}}</td>
- </tr>
- </tbody>
- </table>
- </div>
- </div>
- <script src = "js/angular.js"></script>
- <script src = "js/script.js"></script>
- </body>
- </html>
Creating the Main Function
This code contains the main function of the application. This code will submit the POST data when the button is clicked. To do this just copy and write these block of codes as shown below inside the text editor and save it as shown below. save.php- <?php
- require_once'conn.php';
- $firstname = $data->firstname;
- $lastname = $data->lastname;
- $address = $data->address;
- mysqli_query($conn, "INSERT INTO `member` VALUES('', '$firstname', '$lastname', '$address')") or die(mysqli_error());
- ?>
- <?php
- require_once 'conn.php';
- $data[] = $fetch;
- }
- ?>
- var app = angular.module("myModule", [])
- .controller("myController", function($scope, $http, $timeout){
- $http.get('data.php').then(function(response){
- $scope.members = response.data;
- });
- $scope.saveData = function(){
- if($scope.firstname == null || $scope.lastname == null || $scope.address == null){
- alert("Please complete the required field");
- }else{
- $http.post("save.php", {firstname: $scope.firstname, lastname: $scope.lastname, address: $scope.address})
- .then(function(){
- $scope.firstname = "";
- $scope.lastname = "";
- $scope.address = "";
- $scope.getData();
- });
- }
- }
- $scope.getData = function(){
- $http.get('data.php').then(function(response){
- $scope.members = response.data;
- });
- }
- });
Add new comment
- 87 views