Laravel CRUD Series: Fetching Data using AJAX
Submitted by nurhodelta_17 on Friday, November 10, 2017 - 18:22.
Getting Started
First, I'm going to create a new project named mysite and add this to my localhost with the name mysite.dev. If you have no idea how to do this, please refer to my tutorial Installing Laravel.Creating and Connecting our Database
In your phpMyAdmin, create a new database. In my case, I've create a database named mysite. In yourproject, in my case mysite, open .env file. Edit the ff lines with you database, username and password:
DB_DATABASE=mysite
DB_USERNAME=root
DB_PASSWORD=
Creating our Controller and Model
Next, we're going to create our controller and model. To do this, open your command prompt, navigate to the project that you have created and type the ff codes: For Controller type: php artisan make:controller MemberController This code will create our controller in the form of MemberController.php file located in youproject/app/Http/Controller. Open this file and change it with the following codes:- <?php
- namespace App\Http\Controllers;
- use Illuminate\Http\Request;
- use App\Member;
- class MemberController extends Controller
- {
- public function index(){
- return view('show');
- }
- public function getMembers(){
- $members = Member::all();
- }
- }
- <?php
- namespace App;
- use Illuminate\Database\Eloquent\Model;
- class Member extends Model
- {
- protected $table = 'members';
- }
- <?php
- use Illuminate\Support\Facades\Schema;
- use Illuminate\Database\Schema\Blueprint;
- use Illuminate\Database\Migrations\Migration;
- class CreateMembersTable extends Migration
- {
- /**
- * Run the migrations.
- *
- * @return void
- */
- public function up()
- {
- Schema::create('members', function (Blueprint $table) {
- $table->increments('id');
- $table->string('firstname');
- $table->string('lastname');
- $table->timestamps();
- });
- }
- /**
- * Reverse the migrations.
- *
- * @return void
- */
- public function down()
- {
- Schema::dropIfExists('members');
- }
- }
Making our Database Migration
In command prompt, navigate to your project and type: php artisan migrate. This will create our table. If you have an error like this: [Illuminate\Database\QueryException] SQLSTATE[42000]: Syntax error or access violation: 1071 Specified key was t oo long; max key length is 767 bytes (SQL: alter table `users` add unique ` users_email_unique`(`email`)) You can solve this by opening AppServiceProvider.php located in yourproject/app/Providers. Add this line: use Illuminate\Support\Facades\Schema; In boot add this line: Schema::defaultStringLength(191);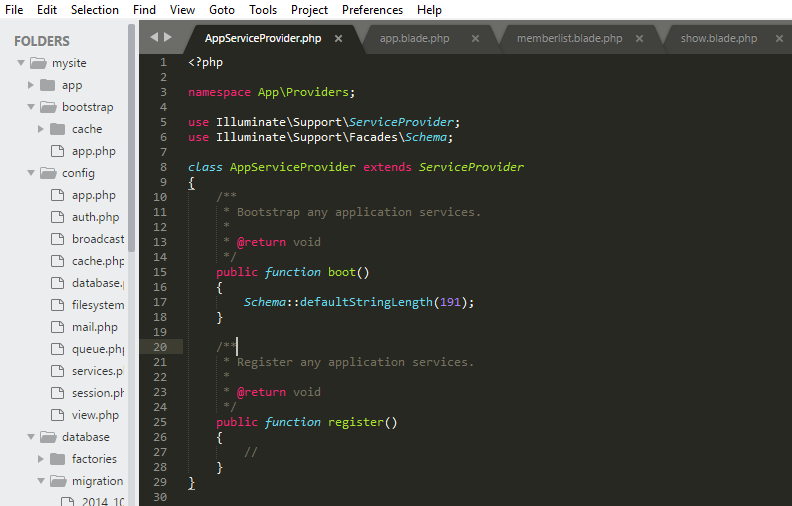
Creating our Routes
In yourproject/routes, open web.php and edit it with the ff codes:
Route::get('/', 'MemberController@index');
Route::get('/show', 'MemberController@getMembers');
Creating our Views
In yourproject/views, create the ff files: app.blade.php- <!DOCTYPE html>
- <html>
- <head>
- <meta charset="utf-8">
- <meta name="csrf-token" content="{{ csrf_token() }}">
- <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css" />
- <link rel="stylesheet" href="/css/app.css">
- <link href="https://maxcdn.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css" rel="stylesheet">
- </head>
- <body>
- <div class="container">
- @yield('content')
- </div>
- @include('modal')
- @yield('script')
- </body>
- </html>
- @extends('app')
- @section('content')
- <div class="row">
- <div class="col-md-10 col-md-offset-1">
- <h2>Members Table
- </h2>
- </div>
- </div>
- <div class="row">
- <div class="col-md-10 col-md-offset-1">
- <table class="table table-bordered table-responsive table-striped">
- <thead>
- </thead>
- <tbody id="memberBody">
- </tbody>
- </table>
- </div>
- </div>
- @endsection
- @section('script')
- <script type="text/javascript">
- $(document).ready(function(){
- showMember();
- $('#add').click(function(){
- $('#addnew').modal('show');
- });
- });
- function showMember(){
- $.get("{{ URL::to('show') }}", function(data){
- $('#memberBody').empty().html(data);
- })
- }
- </script>
- @endsection
Running the Server
In your web browser, type the localhost name of your project, in my case mysite.dev Add sample data in your phpMyAdmin manually for now and you should be able to view our table.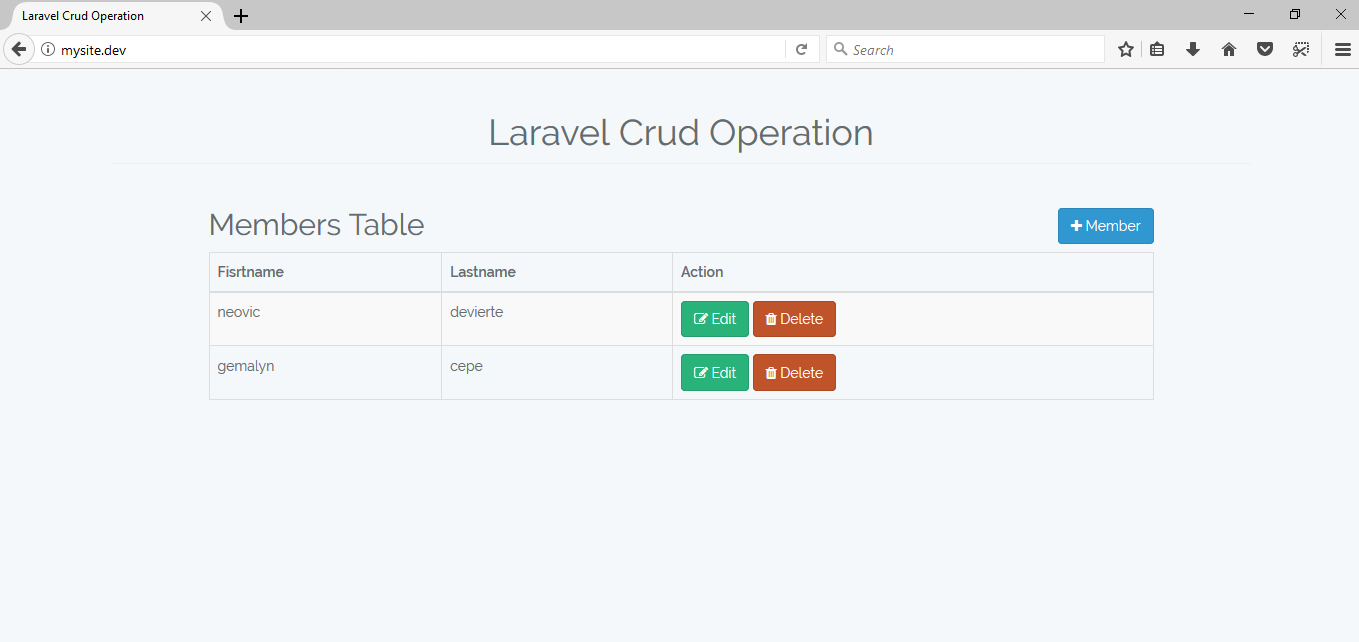
Add new comment
- 511 views