Small and Capital Letters Count in PHP
Submitted by azalea zenith on Friday, October 14, 2016 - 15:05.
In this tutorial, we are going to create a simple program that can count small and capital letters in the webpage and it's called Small and Capital Letters Count in PHP. If you are looking for this kind of program then you are at the right place. This simple program is written using PHP and HTML. This is a very short PHP query that can count the number of Small and Capital letters in a given word by the user enters in the TextBox. This simple source code is very simple to use yet easy to understand. Let's create, so you can have this simple program and try it to your computer.
Live Demo below:
How to Create it
First, we are going to create our simple markup and it contains the TextBox and the button to count the small and capital letters given by the user. Source code look like this:
We create a simple query where the small and capital letters separate. The purpose of this query, our program will know the format of small and capital letters and to have an easy count when the button hit by the user.
Using the PHP query below, getting the information from the user enters in the TextBox, so we can get the desired word or phrase from the user then this simple query will get it and count the small and capital letters given from the user.
After executing the source code above, in the source code below, used to display the result count of small and capital letters. Look the simple markup below with PHP source code to display the result.
This would be the output
If you have a question regarding this simple program, kindly leave a comment below. Enjoy coding. Thank you.
The source code will look like this in the photo below.
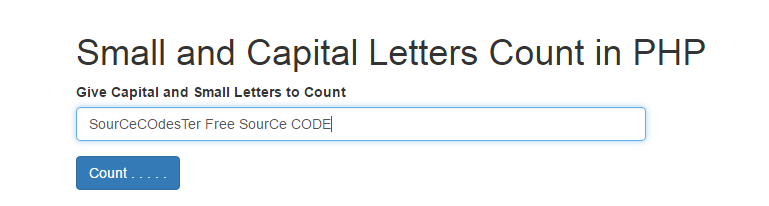
- <?php
- function count_Capital_Letter($string_letter)
- {
- }
- function count_Small_Letter($string_letter)
- {
- }
- ?>
- <?php
- {
- $string_word = $_POST['string_word'];
- $result1 = count_Capital_Letter($string_word);
- $result2 = count_Small_Letter($string_word);
- ?>
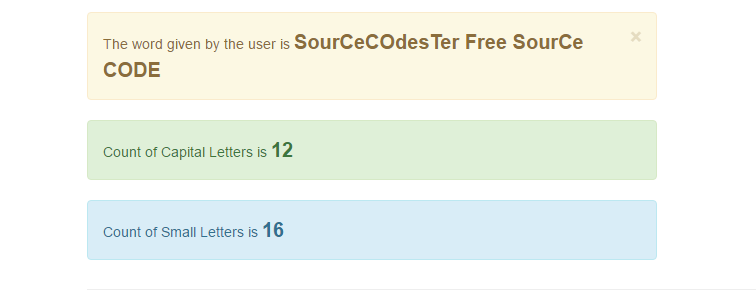
Here's the full source code
Study the source code and try this simple program to your computer. Enjoy coding. Thank you.- <div style="width:50%;">
- <form method="post">
- <div>
- <label>Give Capital and Small Letters to Count</label>
- <input type="text" name="string_word" placeholder="Give Capital and Small Letters to Count . . . . ." autofocus="autofocus" />
- </div>
- <button type="submit" name="count_letter">Count . . . . .</button>
- </form>
- <?php
- function count_Capital_Letter($string_letter)
- {
- }
- function count_Small_Letter($string_letter)
- {
- }
- {
- $string_word = $_POST['string_word'];
- $result1 = count_Capital_Letter($string_word);
- $result2 = count_Small_Letter($string_word);
- ?>
- <br />
- <div>
- <button type="button"><span>×</span></button>
- The word given by the user is <strong style="font-size:20px;"><?php
- echo $string_word; ?></strong>
- </div>
- <div>Count of Capital Letters is <strong style="font-size:20px;"><?php
- echo $result1; ?></strong></div>
- <div>Count of Small Letters is <strong style="font-size:20px;"><?php
- echo $result2; ?></strong></div>
- <?php
- } ?>
- </div>
Add new comment
- 151 views