Calculator using Dropdown in PHP
Submitted by azalea zenith on Tuesday, October 11, 2016 - 12:07.
In this tutorial, we are going to create Calculator using Dropdown in PHP. I create this simple program in PHP using the drop-down menu to select a mathematical operator such as Addition, Subtraction, Multiplication, and Division.
Check the live demo, click the button below.
First, we have to create the markup for this program. This source code contains two TextBox where the user enters the desired numeric value and one combo box where they can select in what mathematical operator they have to use.
To execute the calculator, we need to construct the PHP query where the operators can run after hit the calculate button on the web page. Let's create one by one.
For Addition
For Subtraction
For Multiplication
For Division
For the complete source code.
Using the source code below, you can use a mathematical operator such as Addition, Subtraction, Multiplication, and Division.
- <form method="post" class="form-inline">
- <div>
- <input type="text" name="first_value" value="<?php echo $first_value; ?>" placeholder="First Value . . . . ." autofocus="autofocus" />
- </div>
- <div>
- <select size="1" name="operations">
- </select>
- </div>
- <div>
- <input type="text" name="second_value" value="<?php echo $second_value; ?>" placeholder="Second Value . . . . ." autofocus="autofocus" />
- </div>
- </form>
Sample Output for Addition
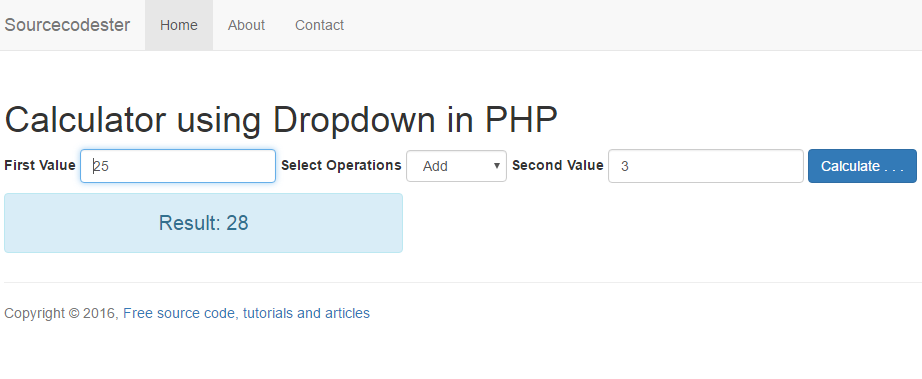
Sample Output for Subtraction
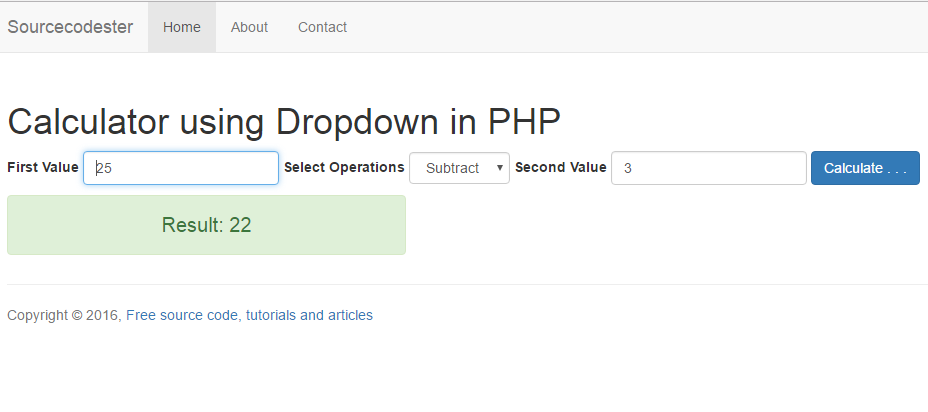
Sample Output for Multiplication
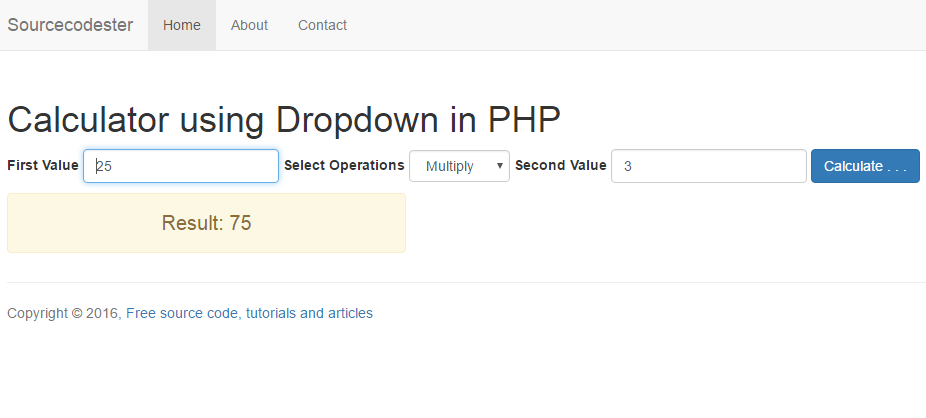
Sample Output for Division
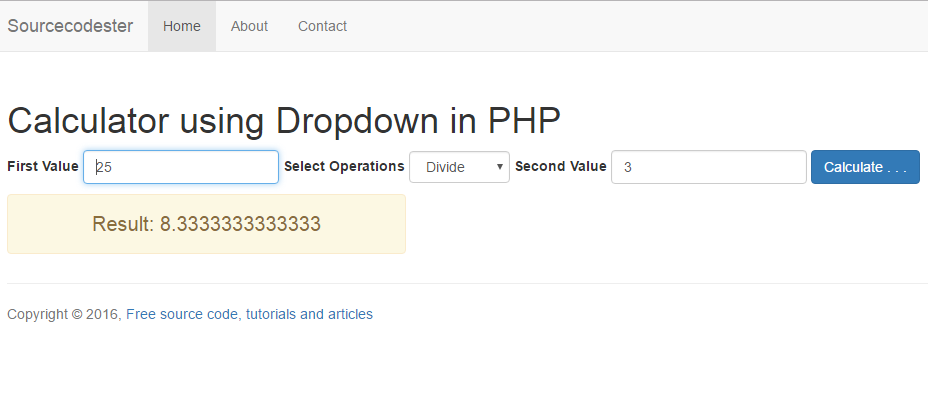
- <div style="width:35%; font-size:20px; text-align:center; margin-top:10px;">
- <?php
- if ($_REQUEST['operations'] == '+')
- { ?>
- <div class="alert alert-info" role="alert">Result: <?php echo $first_value + $second_value; ?></div>
- <?php
- }
- elseif ($_REQUEST['operations'] == '-')
- { ?>
- <div class="alert alert-success" role="alert">Result: <?php echo $first_value - $second_value; ?></div>
- <?php
- }
- elseif ($_REQUEST['operations'] == '*')
- { ?>
- <div class="alert alert-warning" role="alert">Result: <?php echo $first_value * $second_value; ?></div>
- <?php
- }
- elseif ($_REQUEST['operations'] == '/')
- { ?>
- <div class="alert alert-warning" role="alert">Result: <?php echo $first_value / $second_value; ?></div>
- <?php
- } ?>
- </div>
Add new comment
- 2048 views