Automatic Page Scroll Load Data
Submitted by rinvizle on Tuesday, August 2, 2016 - 16:09.
In this tutorial we will show you how to create a Automatic Page Scroll Load Data in PHP. Automatic Page Scroll Load Data will help us and show an automatic process to load more data from the database in PHP. Creating a Automatic Page Scroll Load Data compose of a script using jQuery, Ajax, and PHP. And it will be very user friendly because of the data that would be loaded from the MySQL database automatically while you scrolling the page down. I have a example script of code.
Database Configuration - dbConfig.php is a configuration of file, in this file we will create a database connection and select the data in MySQL database.
PHP and HTML Form
Hope that you learn in this project and enjoy coding. Don't forget to LIKE & SHARE this website.
Sample Code
Data.php - Using the data.php file, we’ll fetch the data from the MySQL database based on the last data ID and generate the data list HTML. Ajax success method gets this HTML and renders the HTML content to the respective DIV element.- <?php
- include('dbConfig.php');
- $lastID = $_POST['id'];
- $showLimit = 1;
- $queryAll = $db->query("SELECT COUNT(*) as num_rows FROM data WHERE id < ".$lastID." ORDER BY id DESC");
- $rowAll = $queryAll->fetch_assoc();
- $allNumRows = $rowAll['num_rows'];
- $query = $db->query("SELECT * FROM data WHERE id < ".$lastID." ORDER BY id DESC LIMIT ".$showLimit);
- if($query->num_rows > 0){
- while($row = $query->fetch_assoc()){
- $postID = $row["id"]; ?>
- <div class="list-item"><a href="javascript:void(0);"><h2><?php echo $row["title"]; ?></h2></a></div>
- <?php } ?>
- <?php if($allNumRows > $showLimit){ ?>
- <div class="load-more" lastID="<?php echo $postID; ?>" style="display: none;">
- <img src="assets/loading.gif"/>
- </div>
- <?php }else{ ?>
- <div class="load-more" lastID="0">
- No More Post To Load...
- </div>
- <?php }
- }
- }
- ?>
- <?php
- $dbhost = 'localhost';
- $dbusername = 'root';
- $dbpassword = '';
- $dbname = 'loaddata';
- $db = new mysqli($dbhost, $dbusername, $dbpassword, $dbname);
- die("Error! Unable To Connect The Database: " . $db->connect_error);
- }
- ?>
- <!DOCTYPE html>
- <html lang="en">
- <head>
- <title>Automatic Page Scroll Load Data</title>
- <link type="text/css" rel="stylesheet" href="assets/css/style.css">
- <link type="text/css" rel="stylesheet" href="bootstrap/css/bootstrap.min.css">
- <script src="assets/js/jquery.min.js"></script>
- <script type="text/javascript">
- $(document).ready(function(){
- $(window).scroll(function(){
- var lastID = $('.load-more').attr('lastID');
- if ($(window).scrollTop() == $(document).height() - $(window).height() && lastID != 0){
- $.ajax({
- type:'POST',
- url:'data.php',
- data:'id='+lastID,
- beforeSend:function(html){
- $('.load-more').show();
- },
- success:function(html){
- $('.load-more').remove();
- $('#postList').append(html);
- }
- });
- }
- });
- });
- </script>
- </head>
- <body>
- <?php
- include('dbConfig.php');
- ?>
- <div class="container">
- <div class="row">
- <div class="col-lg-12">
- <h1 align="center">Automatic Page Scroll Load Data</h1>
- <div class="post-list" id="postList">
- <?php
- $query = $db->query("SELECT * FROM data ORDER BY id DESC LIMIT 7");
- if($query->num_rows > 0){
- while($row = $query->fetch_assoc()){
- $postID = $row["id"];
- ?>
- <div class="list-item" href="javascript:void(0);"><h2><?php echo $row['title']; ?></h2></div>
- <?php } ?>
- <div class="load-more" lastID="<?php echo $postID; ?>" style="display: none;">
- <img src="assets/loading.gif"/>
- </div>
- <?php } ?>
- </div>
- </div>
- </div>
- </div>
- </body>
- </html>
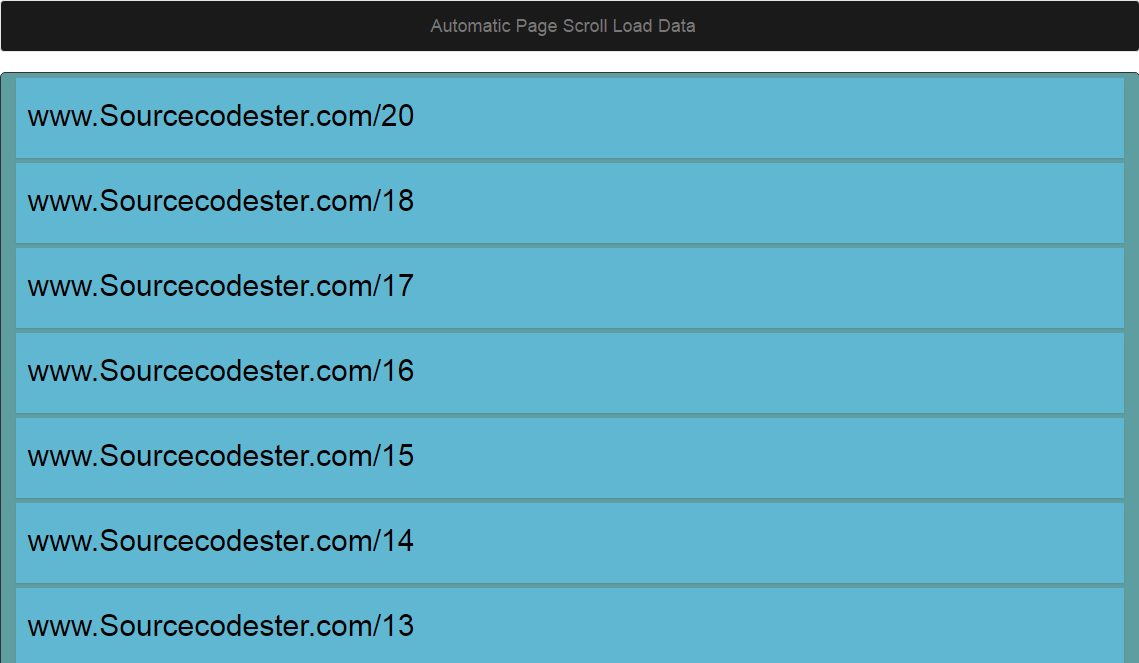
Add new comment
- 387 views