Decode String into Integer in Java
Submitted by donbermoy on Wednesday, December 24, 2014 - 21:53.
This tutorial will teach you how to create a program that will decode a string into integer in java.
So, now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of decodeNumberSystem.java.
2. We will initialize variables in our Main, variable named decimal,octal,and hexa as String. We have initialized this variable to make this an Integer.
3. To decode a string, we will use the decode method of the integer data type and put inside the String variables that we have declare above.
Here's the full code of this tutorial:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
A decode method of the integer data type decodes a String. It accepts decimal, hexadecimal, and octal numbers.
4. Lastly, display the decoded integer value of the inputted string.
Output:
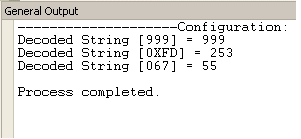
- public class decodeNumberSystem {
- }
- }
Add new comment
- 28 views