How to have a Formatted Date in Java
Submitted by donbermoy on Tuesday, December 2, 2014 - 22:33.
This tutorial is about how to have a formatted date in java. We will use the DateFormatter and some text packages library of java here.
So, now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of defaultFormatterFactory.java.
2. Import the following package library.
3. Initialize your variable in your Main, variable frame for JFrame, and a Jlabel named label..
To create a formatted date to display we will use the DateFormat,SimpleDateFormat, and DateFormatter class.
The DateFormat class formats and parses dates or time and will use the SimpleDateFormat class to allow formatting of date to text, parsing text to date, and normalization.
Now, we will use the DefaultFormatterFactory class to accommodate if a more specific formatter could not be found. The DateFormatter variables will be put inside the DefaultFormatterFactory class. Have this code below:
4. Now, create JFormattedTextField component named input to have the formatted date be in the DefaultFormatterFactory be in the JFormattedTextField. Create also a JPanel to put their the JFormattedTextField.
Limit the characters of the JFormattedTextField using the setColumn method.
Add the label and the formatted textfield in the panel using the add method.
5. Lastly, add the panel to the frame, add a textfield to the frame, pack the frame, set its visibility to true, and have its close operation.
Output:
Here's the full code of this tutorial:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- import java.text.*; // use to access DateFormat and SimpleDateFormat class
- import javax.swing.*; //used to access JFrame,JLabel,JPanel, JTextField, and JFormattedTextField class
- import javax.swing.text.*; //used to access DateFormatter, and DefaultFormatterFactory class
- DateFormatter displayFormatter = new DateFormatter(displayFormat);
- DateFormatter editFormatter = new DateFormatter(editFormat);'
- DefaultFormatterFactory factory = new DefaultFormatterFactory(displayFormatter,
- displayFormatter, editFormatter);
- txtInput.setColumns(30);
- panel.add(label);
- panel.add(txtInput);
- frame.getContentPane().add(panel,"North");
- frame.pack();
- frame.setVisible(true);
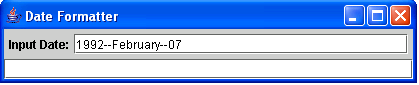
- import java.text.*; // use to access DateFormat and SimpleDateFormat class
- import javax.swing.*; //used to access JFrame,JLabel,JPanel, JTextField, and JFormattedTextField class
- import javax.swing.text.*; //used to access DateFormatter, and DefaultFormatterFactory class
- public class defaultFormatterFactory {
- DateFormatter displayFormatter = new DateFormatter(displayFormat);
- DateFormatter editFormatter = new DateFormatter(editFormat);
- DefaultFormatterFactory factory = new DefaultFormatterFactory(displayFormatter,
- displayFormatter, editFormatter);
- JFormattedTextField input = new JFormattedTextField(factory);
- input.setColumns(30);
- panel.add(label);
- panel.add(input);
- frame.getContentPane().add(panel,"North");
- frame.pack();
- frame.setVisible(true);
- }
- }
Add new comment
- 21 views