Built-in Arrow Button in Java
Submitted by donbermoy on Thursday, November 27, 2014 - 18:26.
This is a tutorial in which we will going to create a program that will have a built-in arrow button in Java.
So, now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of arrowButton.java.
2. Import the following packages:
We will use the BasicArrowButton class of the javax.swing.plaf package to have the arrow buttons.
3. Initialize your variable in your Main, variable frame for JFrame only.
4. To add the built-in arrow to the frame, we will use the getContentPane.add method then instantiate the BasicArrowButton class and the position of the arrows that you want.
As you have seen the code above, we have created four buttons with different position, the North, South, West, and East position, respectively.
5. Now, have the frame set to a FlowLayout as the layout manager. Lastly, set visibility, close operation of the frame, and pack the frame. Have this code below:
Output:
Here's the full code of this tutorial:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- import java.awt.*; //used to access the FlowLayout class
- import javax.swing.*; //used to access the JFrame class
- import javax.swing.plaf.basic.*; //used to access the BasicArrowButton class
- frame.pack();
- frame.setVisible(true);
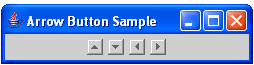
- import java.awt.*; //used to access the FlowLayout class
- import javax.swing.*; //used to access the JFrame class
- import javax.swing.plaf.basic.*; //used to access the BasicArrowButton class
- public class arrowButton{
- frame.pack();
- frame.setVisible(true);
- }
- }
Add new comment
- 114 views