JComboBox Component in Java
Submitted by donbermoy on Friday, November 14, 2014 - 08:08.
This is a tutorial in which we will going to create a program that has the JComboBox component/control using Java.
So, now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of jComboBoxComponent.java.
2. Import the following packages:
3. The jComboBoxComponent classname must extends the JFrame to have the container of the components used. Have also the following variables for the JComboBox below:
Add the JComboBox to the frame using the add method and have it with the flowlayout layout of the frame.
Then pack the frame so that the component/control will be seen directly to the frame. Also set its visibility to true and have it the close operation to exit.
Output:
Here's the full code of this tutorial:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- import java.awt.*; //used to access the flowlayout
- import javax.swing.*; //used to access the JFrame and JComboBox class
We have created a String array of days to be added to the combobox.
4. Now, create a constructor to add the JComboBox to the frame as well as its layout and visibility.
Another way of adding items to the ComboBox is to have the addItem method. As you can see the String array of days variable above has only Monday to Friday. To add another item, have this code below:
- cbo.addItem("Saturday"); // add the value of Saturday to the combobox
- cbo.addItem("Sunday"); // add the value of Sunday to the combobox
- getContentPane().add(cbo); //add the combobox to JFrame
- pack(); //pack the frame
- setVisible(true); //set visibility to true
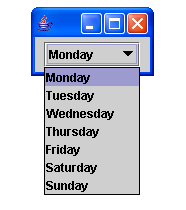
- import java.awt.*; //used to access the flowlayout
- import javax.swing.*; //used to access the JFrame and JComboBox class
- String days[] = { "Monday", "Tuesday", "Wednesday", "Thursday", "Friday"}; //string array of days to be added to combobox
- public jComboBoxComponent() { //constructor
- cbo.addItem("Saturday"); // add the value of Saturday to the combobox
- cbo.addItem("Sunday"); // add the value of Sunday to the combobox
- getContentPane().add(cbo); //add the combobox to JFrame
- pack(); //pack the frame
- setVisible(true); //set visibility to true
- }
- new jComboBoxComponent(); //call the constructor
- }
- }
Add new comment
- 174 views