TUTORIAL NO 10
3 Types of FILE IO part1
In this tutorial you will learn:
File Reading using
1. Standard Input Output
2. New Input Output
3. New Input Output2
4. Anonymous classes
Today I am going to teach you how to read files in java using all the three types of methods available in java. The standard input output was the oldest after that new input output was used and now new input output is being used. To keep things simple we will simply read our program source code from the current directory using the three FILE IOs which will be selected by pressing 3 different buttons.
In this tutorial we will be working in JAVA SWING. The first thing that we are going to do is setting up a JFrame and adding the required panels and Components.
Basic step:
Download and install ECLIPSE and set up a JAVA PROJECT. Then create a new class and name it FileIO. Then follow the steps
1.IMPORT STATEMENTS
First of all write these import statements in your .java file
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.io.*;
import java.nio.*;
import java.nio.file.*;
import java.nio.channels.*;
import java.util.EnumSet;
We require event import for mouse click , awt and swing imports for visual design, io import for standard input output, nio import for new input output and new input output 2. For this tutorial we will only be making two classes JPanel class and frame class. We will add the panel in the frame class
2.
SETTING UP THE PANEL CLASS
class Filepanel
extends JPanel {
long readingTime = 0, beforeReadingTime = 0, afterReadingTime = 0;
String path
;// path of the code
String text
= ""; // appending it into a string and then appending all the
char b[]; // for reading characters in file
First of all we will make the objects for adding text fields , labels and the text area for making the GUI as shown in the picture above. After that we need a scroll pane to add the scroll bars in the text area when necessary. Then we need to make a variable to store the file name and file reference for pointing towards the file and defining the path. Other than that we need character array for a reading the characters in the files.
3. WRITING THE CONTSTUCTOR
Filepanel() {
add(up, "North");
up.add(jl);
up.add(tf);
up.add(rjl);
up.add(timetf);
up.add(jb1);
up.add(jb2);
up.add(jb3);
add(sp); // adding scroll panel
file_name = tf.getText();
if (me.getSource() == jb1)
sio();
}
});
//if (me.getSource() == jb2)
//nio();
}
});
//if (me.getSource() == jb3)
//nio2();
}
});
}// end constructor
In the constructor what we are doing is making a JPanel for adding in the north position and adding the labels and text fields and labels in that panel according to the GUI of the application. Then we are adding mouse listeners to the buttons so that they call the functions accordingly and read the a file by using the functionality of that function whether new or standard io.
void computeReadTime() {
readingTime = afterReadingTime - beforeReadingTime;
timetf.setText("" + readingTime + "ns");
}
long getTime() {
}
In this function we are simply calculating the time it took to read the file. For that we just need to subtract the after reading time from just before reading time (Remember: These variables will have stored values when we will call this function). Then we simply set the text field to that calculated time. After that we simply write another function in which simply returns the system time in nano seconds. We will use these functions in another function.
4. WRITING THE FUNCTION:
void sio() {
myfile
= new File(file_name
);
t_area.setText(" ");
text = "";
if (myfile.exists()) { // if file is found
beforeReadingTime = getTime();
path = myfile.getPath();
b = new char[(int) myfile.length()];
try {
reader.read(b);
afterReadingTime = getTime();
for (int i = 0; i < b.length; i++) {
text += b[i]; }
computeReadTime();
t_area.setText(text);
}
}
else
{
text ="";
t_area.setText(text);
tf.setText("File Not Found.. !!");
}
} // end sio
In this function first of all we clear any previous text written in the text area and then proceed. First of all we check whether the code file exists in the current directory or not if it does the first thing that we do is calculate the time just before the software is about to start reading. Then we set our character array size to the file size to read all the characters the file contains. Then we simply create a file reader reference to read the contents of the file and store in the character array. Then we will append all the characters in the text area to show the complete file contents. After that we simply calculate the time because we have read everything and written everything and we need to calculate the overall time it took to read from the file to writing in the text area. In the else condition we simply set the text to empty and write “file not found” in the text field. After that
// next tutorial
}
// next tutorial
}
}// end Filepanel
Now we will make the two functions for nio and nio2 which we will work on in the next tutorial.
5. WRITING THE MAIN PUBLIC CLASS
public class FileIO
extends JFrame {
static Filepanel fp = new Filepanel();
FileIO() {
setDefaultCloseOperation(FileIO.EXIT_ON_CLOSE);
setSize(1000, 700);
add(fp);
setVisible(true);
}
public static void main
(String args
[]) {
new FileIO();
}// end main
}// end file class
In the main frame class we first make a reference of the panel class to add in our frame class. In the constructor we declared the size of the frame and set it to visible and added the panel in the frame.In the main function we simply created an object of our frame class and our app is 40% complete.
OUPUT:
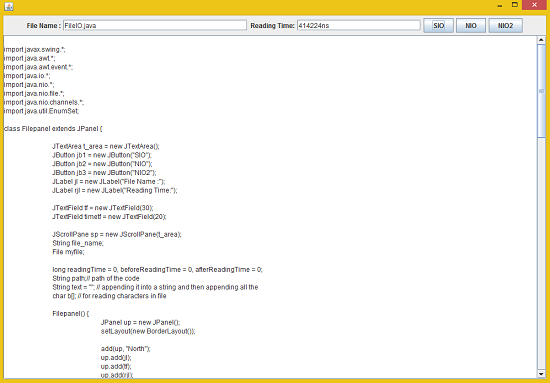