How to Check if an Inputted Year is a Leap Year using Java GUI
Submitted by donbermoy on Sunday, May 25, 2014 - 21:02.
In this tutorial, i will teach you how to check if an inputted year as leap year or not. Hence we already know that leap year has 366 days instead of the normal 365 days. Leap years occur every 4 years. And this fact is our formula in finding a leap year using Java GUI.
So, now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of leapYear.java.
2. Import javax.swing package. Hence we will use a GUI (Graphical User Interface) here like the inputting the year. Import also java.util package to access the Gregorian Calendar class.
3. Initialize your variables in your Main, variable numInput as string and make it as an inputdialogbox. Variable yr as integer that will parse the inputted value of numInput for inputting the year.
4. Now, let's obtain an instance of GregorianCalendar variable of calendar. This will trigger to access the package of java.util in the calendar class of it.
5. To find the Leap year, we will create an If-Else statement. In our if statement we will use the isLeapYear method of our Gregorian Calendar class that we have instantiated earlier. Otherwise, in Else statment, it will prompt not a leap year.
Output:
Here's the full source code:
Hope this helps! :)
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- int yr;
- String numInput;
- if (calendar.isLeapYear(yr))
- {
- }
- else{
- }
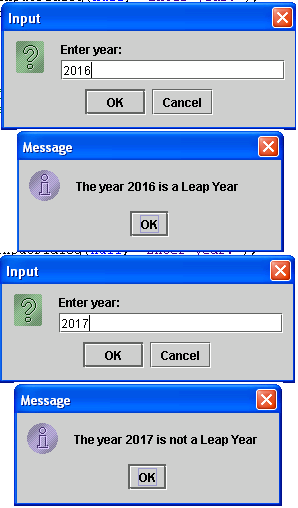
- import javax.swing.*;
- import java.util.*;
- public class leapYear{
- int yr;
- String numInput;
- if (calendar.isLeapYear(yr))
- {
- }
- else{
- }
- }
- }
Add new comment
- 585 views