Populate/Load Database Records into a Combobox using Java
Submitted by donbermoy on Wednesday, April 30, 2014 - 18:44.
Today, I will teach you how to load database records into a combobox using Java.
1. First create an access database file named sample.mdb same with the table and records below.
2. Create now a java program with a filename of PopulateComboBox.java.
3. Import the following libraries below.
4. Initialize the following variables below. Most importantly we will have a combobox inside our form.
6. Now, create a constructor named PopulateComboBox that is the same name with the filename. This will add the controls inside the panels and some important functions in the program.
7. Create a method named add_Cat_combo(JComboBox cmb). This will load the data in the database to the combobox and will connect into the sample.mdb as our database.
8. Lastly, create your Main to set the size and location of the form.
Output:
Here's the full code of this tutorial:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware

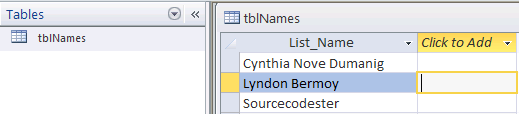
- import java.awt.*;
- import java.awt.event.*;
- import java.io.*;
- import java.sql.*;
- import javax.swing.*;
- import java.util.*;
5. Create a class j2 that will serve as our main panel.
- {
- public j2()
- {
- }
- {
- super.paint(g);
- g.drawString("Populate Combobox",60,70);
- }
- }
- public PopulateComboBox()
- {
- super("Populate Combobox");
- Names.setEditable(false);
- add_Cat_combo(Names);
- panel1.add(Names);
- panel1.setOpaque(true);
- getContentPane().add(new j2(),"NORTH");
- getContentPane().add(panel1,"CENTER");
- getContentPane().add(panel2,"CENTER");
- setDefaultCloseOperation(DISPOSE_ON_CLOSE);
- };
- {
- try{
- Class.forName("sun.jdbc.odbc.JdbcOdbcDriver");
- e.printStackTrace();
- }
- e.printStackTrace();
- }
- try{
- while (rs.next())
- {
- cmb.addItem(rs.getString("List_Name"));
- }
- stmt.close();
- }
- {
- }
- }
- PopulateComboBox p1= new PopulateComboBox();
- p1.setSize(650,630);
- p1.setLocation(300,50);
- p1.setVisible(true);
- p1.setResizable(false);
- }
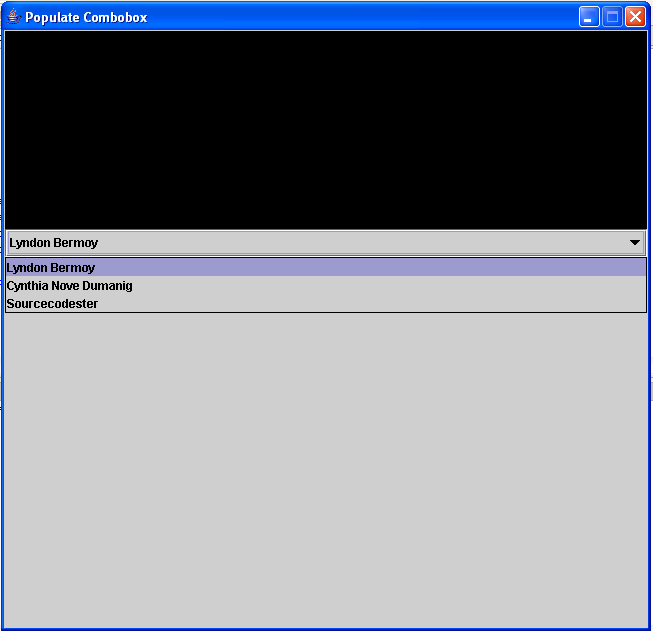
- import java.awt.*;
- import java.awt.event.*;
- import java.io.*;
- import java.sql.*;
- import javax.swing.*;
- import java.util.*;
- Connection cn;
- {
- public j2()
- {
- }
- {
- super.paint(g);
- g.drawString("Populate Combobox",60,70);
- }
- }
- public PopulateComboBox()
- {
- super("Populate Combobox");
- Names.setEditable(false);
- add_Cat_combo(Names);
- panel1.add(Names);
- panel1.setOpaque(true);
- getContentPane().add(new j2(),"NORTH");
- getContentPane().add(panel1,"CENTER");
- getContentPane().add(panel2,"CENTER");
- setDefaultCloseOperation(DISPOSE_ON_CLOSE);
- }
- {
- try{
- Class.forName("sun.jdbc.odbc.JdbcOdbcDriver");
- e.printStackTrace();
- }
- e.printStackTrace();
- }
- try{
- while (rs.next())
- {
- cmb.addItem(rs.getString("List_Name"));
- }
- stmt.close();
- }
- {
- }
- }
- PopulateComboBox p1= new PopulateComboBox();
- p1.setSize(650,630);
- p1.setLocation(300,50);
- p1.setVisible(true);
- p1.setResizable(false);
- }
- }
Add new comment
- 1009 views