Random Rolling Numbers Game in C#
Submitted by donbermoy on Saturday, July 26, 2014 - 10:48.
In this tutorial, we will create a game called Random Rolling Game using C# that is rolled randomly with its number and has a Progress Bar that serves as a timer.
1. Let's start with creating a Windows Form Application in C# for this tutorial by following the following steps in Microsoft Visual Studio: Go to File, click New Project, and choose Windows Application.
2. Design your interface like this:
3. Create this code for your Timer1_Tick to be able to randomly roll the numbers in textbox. Put this code below:
4. In your Button put this code below to start the timer and assigning the Progress Bar of a minimum value.
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
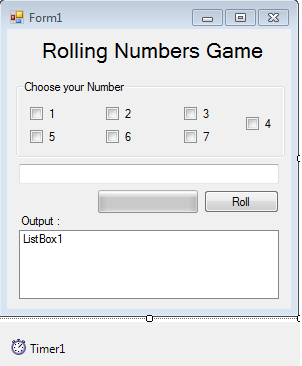
- private void Timer1_Tick(System.Object sender, System.EventArgs e)
- {
- //declaring a random variable to store the numbers on it.
- //it represents a psuedo random generator.
- //declaring an integer variable to store the nonnegative random numbers.
- //starts with the minimum value to the maximum value
- //next represents to return a random number within a specified range.
- int result = System.Convert.ToInt32(num.Next(1, 7));
- //put the result in the textbox.
- TextBox1.Text = result.ToString();
- //progressbar increased by 1.
- ProgressBar1.Value += 1;
- //the condition is, when the progressbar value reached to 100
- //then the timer will stop and the number in the textbox will stop rolling too.
- if (ProgressBar1.Value == 100)
- {
- //filtering the checkbox.
- //if the checkbox1 is checked the message will appear in the listbox.
- {
- if (TextBox1.Text == CheckBox1.Text)
- {
- //add the string message in the listbox.
- ListBox1.Items.Add("The number " + TextBox1.Text + " won!");
- }
- }
- {
- if (TextBox1.Text == CheckBox2.Text)
- {
- ListBox1.Items.Add("The number " + TextBox1.Text + " won!");
- }
- }
- {
- if (TextBox1.Text == CheckBox3.Text)
- {
- ListBox1.Items.Add("The number " + TextBox1.Text + " won!");
- }
- }
- {
- if (TextBox1.Text == CheckBox4.Text)
- {
- ListBox1.Items.Add("The number " + TextBox1.Text + " won!");
- }
- }
- {
- if (TextBox1.Text == CheckBox5.Text)
- {
- ListBox1.Items.Add("The number " + TextBox1.Text + " won!");
- }
- }
- {
- if (TextBox1.Text == CheckBox6.Text)
- {
- ListBox1.Items.Add("The number " + TextBox1.Text + " won!");
- }
- }
- {
- if (TextBox1.Text == CheckBox7.Text)
- {
- ListBox1.Items.Add("The number " + TextBox1.Text + " won!");
- }
- }
- Timer1.Stop();
- }
- }
- private void btnRoll_Click(System.Object sender, System.EventArgs e)
- {
- //clearing the listbox
- ListBox1.Items.Clear();
- //timer starts
- Timer1.Start();
- //progressbar value returns to 0 everytime you click the button
- ProgressBar1.Value = 0;
- }
Output:
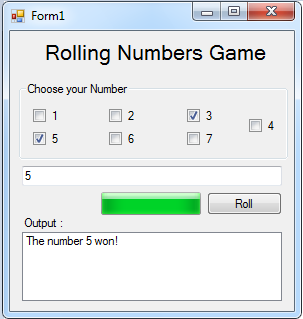
Add new comment
- 202 views