Today in C#, i will teach you to get a time interval in C#. In here, we will use the
TimeSpan properties for getting the time interval. It segregates the hours, minutes, seconds, millisecond and even the tick of the clock.
So, now let's start this tutorial!
1. Let's start with creating a Windows Form Application for this tutorial by following the following steps in Microsoft Visual Studio 2010: Go to File, click New Project, and choose Windows Application and name it as
TimeInterval.
2. Next, add two DateTimePicker named
dtpstrTime for the starting time and
dtpEndTime for the end time. Insert 5 textboxes named
txthours for displaying hours,
txtminutes for displaying minutes,
txtseconds for displaying seconds,
txtmillesecond for displaying millisecond, and
txtticks for displaying ticks. Add also a Button named
btnGo. You must design your interface like this:
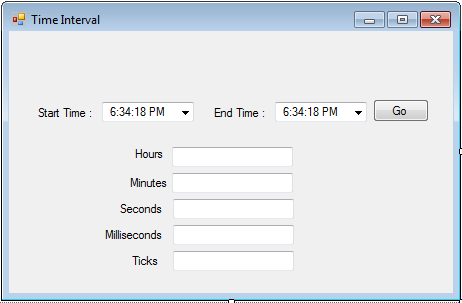
3. Create a sub procedure named
TimeInterval for separating the time intervals of the corresponding fields, put this code below:
private void TimeInterval(TimeSpan ts)
{
//use the properties of the timespan and it demonstrate timespan.hours
//, timespan.milliseconds , timespan.minutes , timespan.seconds
// and timespan.ticks
try
{
txthours.Text = ts.Hours.ToString();
txtmillisecond.Text = ts.Milliseconds.ToString();
txtminutes.Text = ts.Minutes.ToString();
txtseconds.Text = ts.Seconds.ToString();
txtticks.Text = ts.Ticks.ToString();
}
catch (Exception ex)
{
MessageBox.Show(ex.Message, this.Text);
}
}
4. For btnGo, put this code below to get the time interval.
public void btngo_Click(System.Object sender, System.EventArgs e)
{
try
{
//create a variable of a timespan
TimeSpan time_span
= new TimeSpan
();
//create the variable of a datetime
DateTime strt_Date = default(DateTime);
DateTime end_Date = default(DateTime);
//set and convert the time from the datetimepicker
strt_Date = DateTime.Parse(dtpstrTime.Text);
end_Date = DateTime.Parse(dtpEndtime.Text);
//subtract the starting time and the end time
time_span = end_Date.Subtract(strt_Date).Duration();
//perform the sub procedure that you have
//created in displaying the time interval
TimeInterval(time_span);
}
catch (Exception Ex)
{
MessageBox.Show(Ex.Message, this.Text);
}
}
5. Now, put this code in your Form_Load.
public void Form1_Load(System.Object sender, System.EventArgs e)
{
//set the value of the end date higher than the start date
dtpEndtime.Value = DateAndTime.DateAdd(DateInterval.Hour, 9, dtpEndtime.Value);
dtpEndtime.Value = DateAndTime.DateAdd(DateInterval.Minute, 30, dtpEndtime.Value);
dtpEndtime.Value = DateAndTime.DateAdd(DateInterval.Second, 30, dtpEndtime.Value);
}
Output:
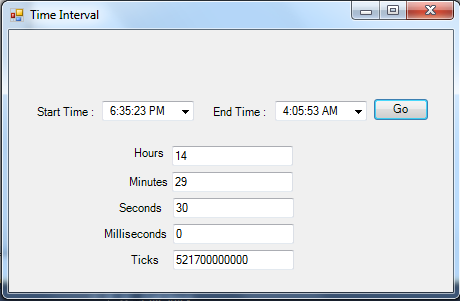
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:
[email protected]
Add and Follow me on Facebook:
https://www.facebook.com/donzzsky
Visit and like my page on Facebook at:
https://www.facebook.com/BermzISware