How to Create an Image Converter in C#
Submitted by donbermoy on Wednesday, July 9, 2014 - 09:45.
Today in C#, I will teach you how to create a program that will convert an image to its file extension in jpeg, bmp, png, and gif.
Now, let's start this tutorial!
1. Let's start with creating a Windows Form Application for this tutorial by following the following steps in Microsoft Visual Studio 2010: Go to File, click New Project, and choose Windows Application.
2. Next, add one PictureBox named picbox, two buttons named btnBrowse and btnconvert, and one ComboBox named cbformats. Add two dialogs named saveDialog as saveFileDialog, and OFDialog as o You must design your interface like this:
3. Click the Items property of your cbformats and encode PNG, BMP, GIF, and JPEG.
4. In your form_load() put this code below:
.
5. In your btnbrowse put this code below so that it can browse image files.
6. In your btnconvert, put this code below to convert the images with its format.
Sample output:
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
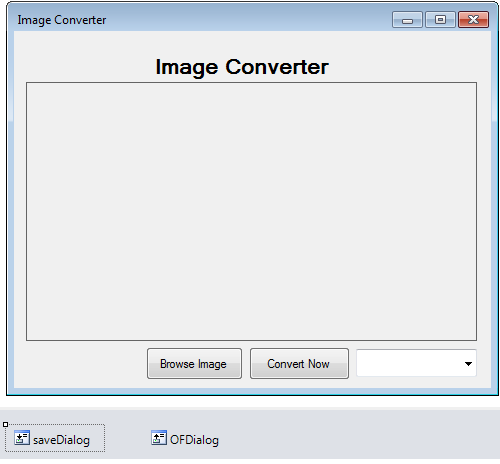
- public void Form1_Load(System.Object sender, System.EventArgs e)
- {
- cbformats.SelectedItem = "PNG";
- }
- public void btnbrowse_Click(System.Object sender, System.EventArgs e)
- {
- try
- {
- // when the Browse Image button is click it will open the OpenfileDialog
- //this line of will check if the dialogresult selected is cancel then
- if (OFDialog.ShowDialog() != System.Windows.Forms.DialogResult.Cancel)
- {
- //the the selected image speciied by the user will be put into out picturebox
- picbox.Image = Image.FromFile(OFDialog.FileName);
- //then the image sizemode is set to strectImage
- picbox.SizeMode = PictureBoxSizeMode.StretchImage;
- //then we assign val in to true because val variable above is declare as boolean
- val = true;
- }
- }
- catch (Exception ex)
- {
- MessageBox.Show(ex.Message);
- }
- }
- public void btnconvert_Click(System.Object sender, System.EventArgs e)
- {
- try
- {
- //it check if the variable val is set to val
- if (val == true)
- {
- //check if what format is selected by user in the combobox
- //the after this the program will convert it to the desired format by the user.
- if ((string) cbformats.SelectedItem == "PNG")
- {
- saveDialog.Filter = "PNG|*.png";
- if (saveDialog.ShowDialog() != System.Windows.Forms.DialogResult.Cancel)
- {
- picbox.Image.Save(saveDialog.FileName, System.Drawing.Imaging.ImageFormat.Png);
- }
- }
- else if ((string) cbformats.SelectedItem == "JPEG")
- {
- saveDialog.Filter = "JPEG|*.jpg";
- if (saveDialog.ShowDialog() != System.Windows.Forms.DialogResult.Cancel)
- {
- picbox.Image.Save(saveDialog.FileName, System.Drawing.Imaging.ImageFormat.Jpeg);
- }
- }
- else if ((string) cbformats.SelectedItem == "BMP")
- {
- saveDialog.Filter = "BMP|*.bmp";
- if (saveDialog.ShowDialog() != System.Windows.Forms.DialogResult.Cancel)
- {
- picbox.Image.Save(saveDialog.FileName, System.Drawing.Imaging.ImageFormat.Bmp);
- }
- }
- else if ((string) cbformats.SelectedItem == "GIF")
- {
- saveDialog.Filter = "GIF|*.gif";
- if (saveDialog.ShowDialog() != System.Windows.Forms.DialogResult.Cancel)
- {
- picbox.Image.Save(saveDialog.FileName, System.Drawing.Imaging.ImageFormat.Gif);
- }
- }
- }
- }
- catch (Exception ex)
- {
- MessageBox.Show(ex.Message);
- }
- }
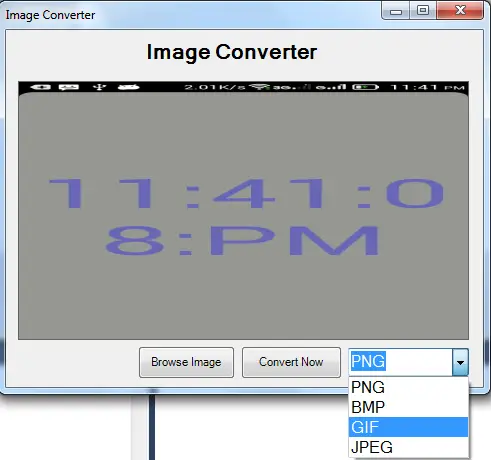
Comments
Add new comment
- Add new comment
- 305 views