Text to Binary Conversion using C#
Submitted by donbermoy on Friday, May 30, 2014 - 17:46.
In this tutorial, I will teach you how to create a program in C# that converts an inputted text to a binary. Because we all know that computers store all characters as numbers stored as binary data. Binary code uses the digits of 0 and 1 (binary numbers) to represent computer instructions or text.
So, now let's start this tutorial!
1. Let's start with creating a Windows Form Application in C# for this tutorial by following the following steps in Microsoft Visual Studio: Go to File, click New Project, and choose Windows Application and name your program ConvertTextToBinary.
2. Next, add two TextBox named TextBox1 to input a text and TextBox2 for displaying the binary conversion of the text you have inputted. Add also a button named Button1. Design your interface like this one below:
3. Put this code to your Button1_Click. This will display the conversion of the text to binary.
Initialize Temp as String to nothing, and txtBuilder to instantiate into a StringBuilder. System.Text.StringBuilder represents a string-like object whose value is a mutable sequence of characters. The value is said to be mutable because it can be modified once it has been created by appending, removing, replacing, or inserting characters.
Create a for each loop with a variable Character as byte to get the equivalent bytes of the TextBox1 using System.Text.ASCIIEncoding.ASCII.GetBytes. The txtBuilder variable will be appended after the conversion of the Character Bytes to String. The padleft method below returns a new string of a specified length in which the beginning of the current string is padded with spaces or with a specified Unicode character. And this padleft right -a ligns the characters. We have also appended a space for us to separate each character as they have different binary equivalents.
After the For Each Loop, the Temp variable will have a value of the TextBuilder with its specified length. Next, all the conversion process holds the Temp Variable and it will be equal and displayed in TextBox2.
Full source code:
Output:
Download the source code below and try it! :)
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
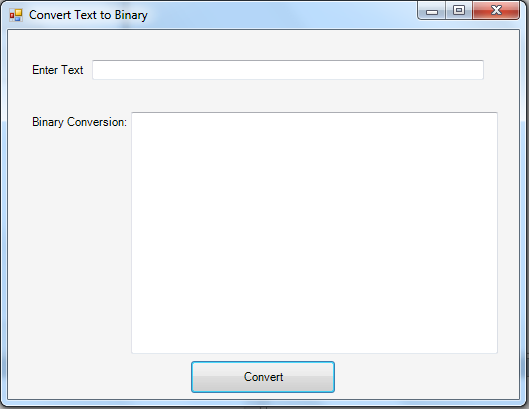
- string Temp = "";
- foreach (byte Character in System.Text.ASCIIEncoding.ASCII.GetBytes(TextBox1.Text))
- {
- txtBuilder.Append(Convert.ToString(Character, 2).PadLeft(8, '0'));
- txtBuilder.Append(" ");
- }
- Temp = txtBuilder.ToString().Substring(0, txtBuilder.ToString().Length - 0);
- TextBox2.Text = Temp;
- using System.Diagnostics;
- using System;
- using System.Windows.Forms;
- using System.Collections;
- using System.Drawing;
- using System.Data;
- using System.Collections.Generic;
- namespace ConvertTextToBinary
- {
- public partial class Form1
- {
- public Form1()
- {
- InitializeComponent();
- }
- public void Button1_Click(System.Object sender, System.EventArgs e)
- {
- string Temp = "";
- foreach (byte Character in System.Text.ASCIIEncoding.ASCII.GetBytes(TextBox1.Text))
- {
- txtBuilder.Append(Convert.ToString(Character, 2).PadLeft(8, '0'));
- txtBuilder.Append(" ");
- }
- Temp = txtBuilder.ToString().Substring(0, txtBuilder.ToString().Length - 0);
- TextBox2.Text = Temp;
- }
- }
- }
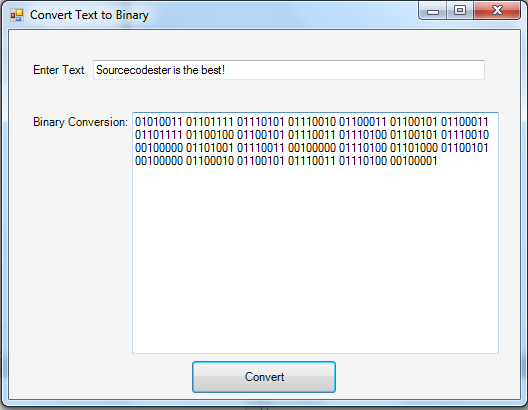
Add new comment
- 652 views