Find Records Between two Dates in C# and MS Access Database
Submitted by janobe on Thursday, July 4, 2019 - 11:50.
In this tutorial, you are going to learn how to find records between two dates using C# and MS Access database. With the use of this method, you will be able to search the records you need in the database between two dates. On the other hand, you can filter the records and display it inside the datagridview. There are times that you might encounter this kind of problem while coding, so I hope this can help you solve it.
Note: Add
Creating Application
Step 1
Open Microsoft Visual Studio 2015 and create a new windows form application for c#.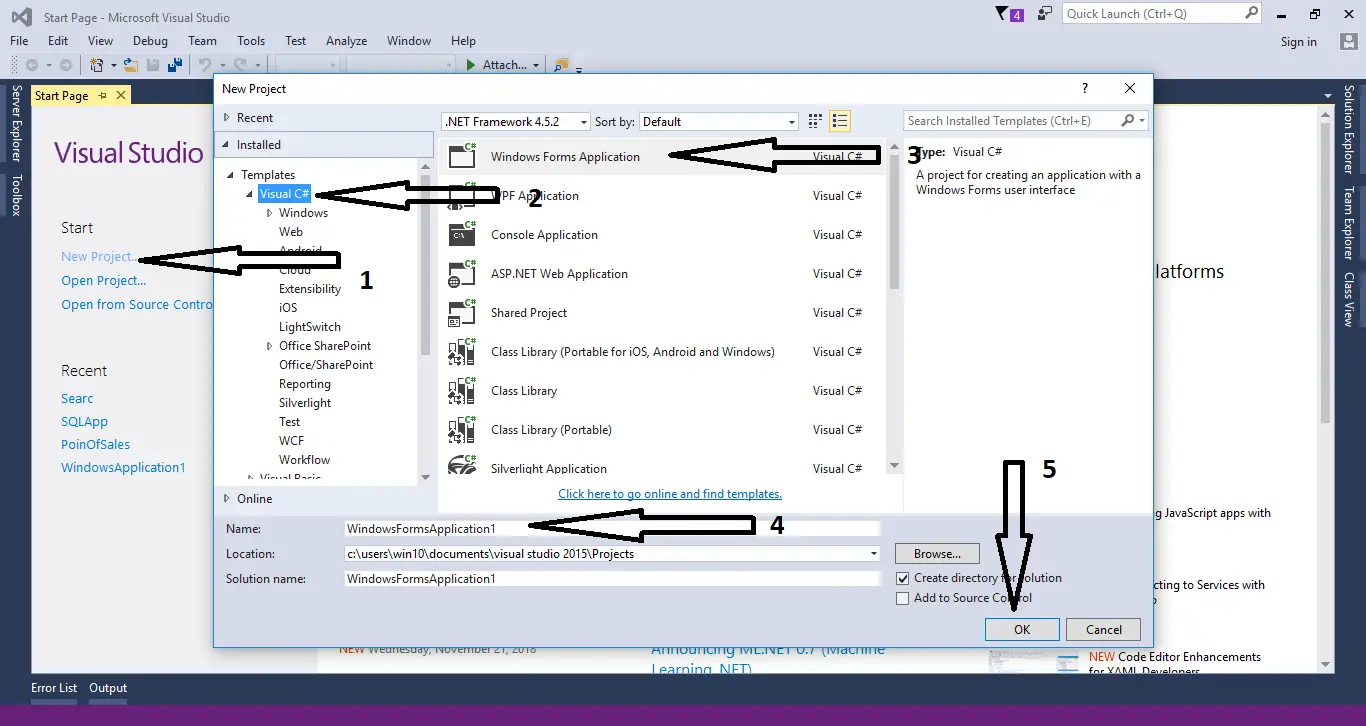
Step 2
Make the Form just like shown below.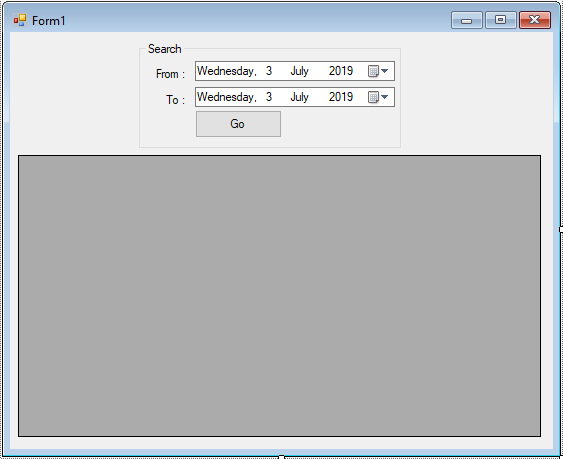
Step 3
Open the code editor and create a connection between c# and ms access database. After that, initialize all the classes that are needed.- OleDbConnection con = new OleDbConnection("Provider=Microsoft.ACE.OLEDB.12.0;Data Source=" + Application.StartupPath + "\\person_db.accdb");
- OleDbCommand cmd;
- OleDbDataAdapter da;
- DataTable dt;
- string sql;
Step 4
Create a method for retrieving data in the database that would be displayed in the datagridview.- private void find_data(string sql, DataGridView dtg)
- {
- try
- {
- con.Open();
- cmd.Connection = con;
- cmd.CommandText = sql;
- da.SelectCommand = cmd;
- da.Fill(dt);
- dtg.DataSource = dt;
- dtg.DefaultCellStyle.WrapMode = DataGridViewTriState.True;
- }
- catch (Exception ex)
- {
- MessageBox.Show(ex.Message);
- }
- finally
- {
- da.Dispose();
- con.Close();
- }
- }
Step 5
Double click the form and call the method that you have created to display the data into the datagridview in the first load of the form.- private void Form1_Load(object sender, EventArgs e)
- {
- sql = "Select Fullname,u_name as Username, u_type as UserRole,DateJoined From tbluser";
- find_data(sql, dataGridView1);
- }
Step 6
Double click the button and do the following codes for searching data between two dates.- private void button1_Click(object sender, EventArgs e)
- {
- sql = "Select Fullname,u_name as Username, u_type as UserRole,DateJoined From tbluser WHERE DateJoined >= #" + dtpFrom.Value.ToString("MM/dd/yyyy") + "# and DateJoined >= #" + dtpFrom.Value.ToString("MM/dd/yyyy") + "#";
- find_data(sql, dataGridView1);
- }
using System.Data.OleDb;
above the namespace to access OleDB libraries.
The complete source code is included. You can download it and run it on your computer.
For more question about this article. You can contact me @
Email – [email protected]
Mobile No. – 09305235027 – TNT
FB Account – https://www.facebook.com/onnaj.soicalap
Or feel free to comment below.v
Add new comment
- 1197 views