How to Navigate Records Based on DataGridView Using C#
Submitted by janobe on Wednesday, December 12, 2018 - 21:29.
This time, let’s learn how to navigate records based on datagridview using C#. This tutorial will show to you that you can put limitation on your displayed records in the datagridview. Sometimes, it’s stressful to scroll down if you are looking for a record that contains a lot of data. Well, this tutorial is a big help for you because you don’t have to scroll down anymore. You can now easily find the record that you’re looking for in just a click. Simply click the next and previous button to see other displayed records.
Note: The database file is included inside the folder.
The complete source code is included. You can download it and run it on your computer
For any questions about this article. You can contact me @
Email – [email protected]
Mobile No. – 09305235027 – TNT
Or feel free to comment below.
Creating Database
Create a database named "dbsubjects". Create a table in the database that you have created.
Add the data in the table.
- (8, 'English Plus', 'English Plus', 3, 'Nones'),
- (14, 'NSTP1', 'National Service Training Program 1', 3, 'None'),
- (15, 'PE1', 'Physical Education 1', 2, 'None'),
- (16, 'HUMAN', 'Humanities', 3, 'None'),
- (17, 'COMART2', 'Communication Arts 2', 3, 'COMART1'),
- (18, 'COPRO-2', 'Computer Programming 2', 4, 'COPRO1'),
- (19, 'DATSTRUC', 'Data Structures', 4, 'COPRO1'),
- (20, 'DISTRUC', 'Discrete Structure', 3, 'ALGEBRA'),
- (21, 'NSTP2', 'National Service Training Program 2', 3, 'None'),
- (22, 'INTROART', 'Introduction to Arts', 3, 'None'),
- (23, 'PE2', 'Physical Education 2', 2, 'PE1'),
- (24, 'TRIGO', 'Trigonometry', 3, 'ALGEBRA'),
- (25, 'COMART3', 'Communication Arts 3', 3, 'COMART2'),
- (26, 'COPRO-3', 'Computer Programming 3', 4, 'COPRO2'),
- (27, 'LOGIC', 'Logic Design and Switching', 3, 'DISTRUC'),
- (28, 'PHILGOV', 'Philippine Government', 3, 'None'),
- (29, 'PHYSICS1', 'Physics 1', 4, 'None'),
- (30, 'STAT', 'Statistics', 3, 'ALGEBRA'),
- (31, 'SOCSTUD', 'Social Studies', 3, 'None'),
- (32, 'PE3', 'Physical Education 3', 2, 'PE1'),
- (33, 'COMART4', 'Communication Arts 4', 3, 'COMART3'),
- (34, 'ASSEM', 'Assembly Language', 4, 'LOGIC'),
- (35, 'PHILO', 'Philosophy', 3, 'None'),
- (36, 'SADSIGN', 'System Analysis and Design', 3, 'COPRO1'),
- (37, 'PHYSICS2', 'Physics 2', 4, 'Physics 1'),
- (38, 'DBSYS', 'Theory Database Systems', 3, 'DATSTRUC'),
- (39, 'PE4', 'Physical Education 4', 2, 'PE1'),
- (40, 'Eng 111', 'CommunicationArts 1', 3, 'none'),
- (41, 'Fil 111', 'Kom sa Akad. Fil', 3, 'NONE'),
- (42, 'Math 1', 'Basic math ', 3, 'NONE'),
- (43, 'SCE 111', 'Earth Science', 3, 'NONE '),
- (44, 'Lit 111', 'Philippines Literature ', 3, 'NONE'),
- (45, 'Rdg 1', 'Dev. Reading 1', 3, 'NONE'),
- (46, 'Psych 116', 'General Psychology', 3, 'NONE'),
- (47, 'PE 111', ' Physical Fitness', 2, 'NONE'),
- (48, 'NSTP 111', 'National Service training program 1 ', 3, 'NONE'),
- (49, 'Eng 211', 'speech & oral communication', 3, 'Eng 121'),
- (50, 'Fil 211', 'Masining na pagpapahayag', 3, 'Fil 121'),
- (51, 'Lit 121 ', 'World literature', 3, 'Lit 111'),
- (52, 'SocSci 132', 'Rizal & other heroes ', 3, 'NONE'),
- (53, 'Ed 211', 'Child & adolescent Psychology', 3, 'Psyh 116'),
- (54, 'Ed 212', 'Education Technology 1', 3, 'NONE'),
- (55, 'FS 1', 'Observation of learners Dev\'t & the school enviroment ', 3, 'NONE'),
- (56, 'Eng 212', 'The Teaching Of speaking ', 3, 'NONE '),
- (57, 'Eng 213', 'The teaching of lsting & Rdg.', 2, 'NONE'),
- (58, 'PE 211', 'Team sports', 2, 'PE 121 '),
- (59, 'Stat 115', 'Intro. to Statistics ', 3, 'NONE'),
- (60, 'Ed 311', 'The teaching profession ', 3, 'NONE'),
- (61, 'Ed 312', 'Assessment of Teaching 2 ', 3, 'Ed 221'),
- (62, 'Ed 313', 'Principles of teaching', 3, 'Ed 224'),
- (63, 'FS 3', 'Micro-Teaching &the use of technology', 1, 'FS 2'),
- (64, 'Eng 311', 'Arfo- Asian Literature ', 3, 'NONE '),
- (65, 'Eng 312', 'Introduction to Stylistics ', 3, 'NONE'),
- (66, 'Eng 313', 'Translating & Editing of text ', 3, 'NONE'),
- (67, 'Eng 314', 'Teaching Literature', 3, 'NONE'),
- (68, 'Ed 314', ' Teaching Multigrade Class', 1, 'NONE'),
- (69, 'Ed 412', 'Use of popular Media ', 1, 'NONE'),
- (70, 'FS 5', 'Assestment of student Learning ', 1, 'FS 4'),
- (71, 'FS 6 ', 'Becoming a Teacher', 1, 'FS 5'),
- (72, 'Hum116', 'Art , Man & Society', 3, 'Hum 111'),
- (73, 'Eng 411', 'language & literature assestment', 3, 'NONE'),
- (74, 'Eng 412', 'lang. Curr. for sec. sch.', 3, 'NONE'),
- (75, 'Eng 413', 'Literary Criticism ', 3, 'NONE'),
- (76, 'Eng 414', 'Language Research ', 3, 'NONE'),
- (77, 'Eng 415', 'Dramatic & Stagecrafts', 3, 'NONE '),
- (78, 'CS 113', 'Basic Software Package w/ Internet Application', 3, 'None'),
- (79, 'Eng 111', 'Communication Arts', 3, 'None'),
- (80, 'Fil 111', 'Kom. sa Akad. Fil', 3, 'None'),
- (81, 'Math 1', 'Basic Math', 3, 'None'),
- (82, 'SCE 111', 'Earth Science', 3, 'None'),
- (83, 'Read 1', 'Dev. Reading 1', 3, 'None'),
- (84, 'Psych 116', 'General Psycology', 3, 'None'),
- (85, 'PE 111', 'Physical Fitness', 2, 'None'),
- (86, 'NSTP 111', 'Nat\'l Service Trng. Prog. 1', 3, 'None'),
- (87, 'Eng 211', 'Speech and Oral Communication', 3, 'Eng 121'),
- (88, 'Fil 211', 'Masining na Pagpapahayag', 3, 'Fil 121'),
- (89, 'Lit 121', 'World Literature', 3, 'Lit 111'),
- (90, 'SocSci 132', 'Rizal and Other Heroes', 3, 'None'),
- (91, 'Ed 211', 'Child Psycology', 3, 'Psych 116'),
- (92, 'Ed 212', 'Educational Technology 1', 3, 'None'),
- (93, 'FS 1', 'Observation of Learning Dev\'t and the School Environment', 1, 'None'),
- (94, 'Eng 212', 'The Teaching of Speaking', 3, 'Eng 121'),
- (95, 'Math 3', 'Elem. Theory of Numbers', 3, 'Math 2'),
- (96, 'PE 211', 'Team Sports', 2, 'PE 121'),
- (97, 'JEEP Start 1', ' ', 3, 'None'),
- (98, 'Stat 115', 'Intro. To Statistics', 3, 'None'),
- (99, 'Ed 311', 'The Teaching Profession', 3, 'Ed 221'),
- (100, 'Ed 312', 'Assessment of Student Lrng. 1', 3, 'Ed 221'),
- (101, 'Ed 313', 'Priciples of Teaching 2', 3, 'Ed 224'),
- (102, 'FS 3', 'Micro-Teaching and the use of Technology', 3, 'FS 2'),
- (103, 'SocSci 311', 'Basic Geography', 3, 'None'),
- (104, 'Chem 1', 'Basic Chemistry', 3, 'None'),
- (105, 'Fil 311', 'Pagpapahalagang Pampanitikan', 3, 'Fil 221'),
- (106, 'Math 5', 'Elementary Algebra', 3, 'None'),
- (107, 'Ed 314', 'Tchg. Multigrade Class', 1, 'Ed 221, Ed 222'),
- (108, 'Ed 412', 'Use of Popular Media', 1, 'None'),
- (109, 'FS 5', 'Assmt. of Study Learning', 1, 'FS 4'),
- (110, 'FS 6', 'Becoming a Teacher', 1, 'FS 5'),
- (111, 'Hum 116', 'Art, Man and Society', 3, 'Hum 111'),
- (112, 'Math 6', 'Mthds and Strat in Teaching Math', 3, 'Math 5'),
- (113, 'SocSci 411', 'Geog. and Nat Res of Phil', 3, 'SocSci311'),
- (114, 'HELE 111', 'Home Economic and Livinhood Eductaion', 3, 'None'),
- (115, 'FS PT', 'Practice Teaching(Whole Day)', 6, 'FS 6'),
- (116, 'Ed 320', 'Methods of Research', 3, 'Stat 115'),
- (117, 'Ed 321', 'Social Dimension of Educ.', 3, 'Ed 312'),
- (118, 'Ed 322', 'Assessment of Study Lrng.', 3, 'Ed 312'),
- (119, 'Ed 323', 'Environment Educ.', 1, 'None'),
- (120, 'FS 4', 'Team Teaching: Exploring the Curr.', 1, 'FS 3'),
- (121, 'Lit 321', 'Children\'s Literature', 3, 'None'),
- (122, 'Eng 321', 'Remedial Inst\'n in Eng', 3, 'None'),
- (123, 'Hum 111', 'Intro. to Humanities', 3, 'None'),
- (124, 'Econ 110', 'Prin. of Econ/AgRfm Tax and Coop', 3, 'None'),
- (125, 'Phys 1', 'Fundamental of Physics', 3, 'None'),
- (126, 'Edd 221', 'Facilitating Learning', 3, 'Ed 211'),
- (127, 'Ed 222', 'Educational Technology 2', 3, 'Ed 212'),
- (128, 'Ed 223', 'Curriculum Development', 3, 'None'),
- (129, 'FS 2', 'Classroom Mgt. Skill', 1, 'FS 1'),
- (130, 'Eng 221', 'Creative Writing', 3, 'Eng 121'),
- (131, 'Fil 221', 'Kontem Panitikang Fil', 3, 'None'),
- (132, 'Math 4', 'Plans and Solid Geometry', 3, 'Math 3'),
- (133, 'PE 221', 'Recreational Activities', 2, 'PE 211'),
- (134, 'JEEP Start 1', ' ', 3, 'None'),
- (135, 'Eng 121', 'Writing in the Disc.', 3, 'Eng 111'),
- (136, 'Fil 121', 'Pagbasa at Pagsulat', 3, 'Fil 111'),
- (137, 'Math 2', 'Contemporary Math', 3, 'Math 1'),
- (138, 'SCE 121', 'Survey of BioSci', 3, 'None'),
- (139, 'SCE 122', 'Astronomy', 3, 'None'),
- (140, 'Lit 111', 'Philippine Literature', 3, 'None'),
- (141, 'Read 2', 'Development Rdg. 2', 3, 'Read 1'),
- (142, 'PE 121', 'Rhythmic Activities', 2, 'PE 111'),
- (143, 'NSTP 121', 'Nat\'l Service Trang. Prog. 2', 3, 'NSTP 111'),
- (144, 'Eng 411', 'Lang. and Lit, Assessmet', 3, 'None'),
- (145, 'Eng 121', 'Writing in the disc.', 3, 'Eng 111'),
- (146, 'Eng 121', 'Writing in Disc.', 3, 'Eng 111'),
- (147, 'Fil 121', 'Pagbasa at Pagsulat', 3, 'Fil 111'),
- (148, 'Math 2', 'Contemporary Math', 3, 'Math 1'),
- (149, 'Sce 121', 'Survey of BioSci', 3, 'None'),
- (150, 'Econ 110', 'Prin. Econ/AgRfrm Tax Coop', 3, 'None'),
- (151, 'CS 113', 'Basic Software Pkge. w/ Internet Application', 3, 'None'),
- (152, 'Socio 115', 'Culture, Soc. and Fam w/ ARH', 3, 'None'),
- (153, 'PE 121', 'Rhythmic Activities', 2, 'PE 111'),
- (154, 'NSTP 121', 'Nat\'l. Service Trng. Prog. 2', 3, 'NSTP 111'),
- (155, 'Ed 221', 'Facilitating Learning', 3, 'Ed 211'),
- (156, 'Ed 222', 'Educational Technology 2', 3, 'Ed 212'),
- (157, 'Ed 223', 'Curriculum Development', 3, 'None'),
- (158, 'Ed 224', 'Principles of Teaching 1', 3, 'None'),
- (159, 'FS 2', 'Classroom Mgt. Skills', 1, 'FS 1'),
- (160, 'Eng 221', 'Intro. to Linguistics', 3, 'None'),
- (161, 'Eng 222', 'Campus Journalism', 3, 'None'),
- (162, 'Eng 223', 'Creative Writing', 3, 'None'),
- (163, 'Eng 224', 'Eng and Americal Lit.', 3, 'None'),
- (164, 'PE 221', 'Recreational Activities', 2, 'PE 211'),
- (165, 'Ed 320', 'Methods of Research', 3, 'Stat 115'),
- (166, 'Ed 322', 'Assessment of Stud. Lrng 2', 3, 'Ed 312'),
- (167, 'FS 4', 'Team Tchng: Exploring the Curr.', 1, 'FS 3'),
- (168, 'Ed 321', 'Social Dimension of Educ. ', 3, 'Ed 311'),
- (169, 'Ed 323', 'Environment Educ.', 1, 'None'),
- (170, 'Eng 321', 'Remedial Instr\'n in Eng', 3, 'None'),
- (171, 'Eng 323', 'Structure of English', 3, 'None'),
- (172, 'Eng 324', 'Mythology and Folklore', 3, '3'),
- (173, 'Eng 325', 'Eng for Specific Purpose', 3, 'None'),
- (174, 'Eng 322', 'Prep. and Evaluation of Instructional Materials', 3, 'None'),
- (175, 'FS PT', 'Practice Teaching(Whole Day)', 6, 'FS 5, FS 6'),
- (176, 'Eng 111', 'Communication skills', 3, 'None'),
- (177, 'Fil 111', 'Komunikasyon sa akademikong Pilipino', 3, 'None'),
- (178, 'Math 111', 'College algebra ', 3, 'None'),
- (179, 'CS 111', 'Basic software Package & Internet Application ', 3, 'None '),
- (180, 'Acctg 201', 'Fundamentals of Accounting 1', 3, 'None'),
- (181, 'PE 111', 'physical Fitness', 2, 'None '),
- (182, 'NSTP 111', 'national Service trng. Prog', 3, 'None'),
- (183, 'Hum 111', 'Art Man and Society', 3, 'None '),
- (184, 'Econ 111', 'Intro. To Economics w/ LRT', 3, 'None'),
- (185, 'Psycho 111', 'General Psychology', 3, 'None'),
- (186, 'Math 117', 'Calculus for Business', 3, 'math 111'),
- (187, 'Acctg 211', 'Financial Acctg & Reporting ', 3, 'Acctg 202'),
- (188, 'Hist 111', 'Phil History w/ politics and gov\'t', 3, 'None'),
- (189, 'Eng 113', 'Speech and oral com.', 3, 'Eng 112'),
- (190, 'PE 113', 'Team Sports ', 2, 'PE 111'),
- (191, 'Eng 114', 'Technical writng ', 3, 'Eng 113'),
- (192, 'Mgt 211', 'Human Behavior in Organization ', 3, 'Mgt 111'),
- (193, 'Cost 211', 'Cost Acctg & Cost Mgt. 1', 3, 'Acctg 212'),
- (194, 'Econ 114', 'MicroEconomic theory & Prac.', 3, 'Econ 112'),
- (195, 'CS 212', 'Funfd. of info. sys. Dev\'t', 3, 'CS 211'),
- (196, 'Law 211', 'law on Obiligation & contracts', 3, 'None'),
- (197, 'Pol Sci 211', 'Good Governance & Social Resp', 3, 'Hist 111'),
- (198, 'Math 211', 'Quantitative Techniques in Business', 3, 'Stat 113'),
- (199, 'Econ 114', 'Management Economics ', 3, 'Econ 113'),
- (200, 'Entrep 212', 'Phil. business enviroment', 3, 'Econ 113'),
- (201, 'Law 213', 'Law on sales, labor & other Comi. laws', 3, 'Law 212'),
- (202, 'Net Sci 112', 'Physical Science ', 3, 'Net sci 113'),
- (203, 'Entrep 213', 'Entrepreneurial Business Mgt', 3, 'Entrep 211'),
- (204, 'Soc Sci 111', 'Life & Works of Rizal', 3, 'None'),
- (205, 'Bre 212', 'Business Research 11', 3, 'bre 111'),
- (206, 'Eng1 112', 'Writing in the Dicipline ', 3, 'Eng 111'),
- (207, 'Fil 112', 'Pagbasa at Pagsulat tungo sa pananaliksik', 3, 'Fil 111'),
- (208, 'Math 113', 'Mathematics of investment', 3, 'Math 111'),
- (209, 'CS 211', 'fund. Programming & database theory & appli.', 3, 'CS 111'),
- (210, 'Mgt 111', 'Principles of mgt. & ORaganization ', 3, 'None '),
- (211, 'Acctg 202', 'Fundimentals of Accounting 11', 3, 'Acctg 201'),
- (212, 'PE 112', 'Rhythmic Activities ', 2, 'PE 111'),
- (213, 'NSTP 112', 'Nat.Service trng Prog. 2', 3, 'NSTP 111'),
- (214, 'NSTP 112', 'Nat.Service trng Prog. 2', 3, 'NSTP 111'),
- (215, 'Mktg', 'Principles of Marketing', 3, 'Mgt111'),
- (216, 'Econ112', 'Microeconomics Theory & Practice', 3, 'Econ111'),
- (217, 'Philos111', 'Philosophy, Values Ed. & works Ethics', 3, 'None'),
- (218, 'Stat 113', 'Business Stat w/ Comp. App.', 3, 'Math 113'),
- (219, 'Acctg 212', 'Financial Accounting and Report II', 6, 'Acctg 211'),
- (220, 'Lit 111', 'Philippine Literature', 3, 'Eng 112'),
- (221, 'Nat Sci', 'Siological Science', 3, 'none'),
- (222, 'PE 114', 'Recreational Activities', 2, 'PE 111'),
- (223, 'Engl 114', 'Technical Writing', 3, 'Engl 113'),
- (224, 'Cost 211', 'Cost Acctg & Cost Mgt 1', 3, 'Acctg 212'),
- (225, 'Mgt 211', 'Human Behavioral in Organization', 3, 'Mgt 111'),
- (226, 'Econ 114', 'Macroeconomics Theory & PracticeT', 3, 'Econ 112'),
- (227, 'CS 112', 'Fund. Of Info. Sys. & Sys. Devt.', 3, 'CS 211'),
- (228, 'Law 211', 'Law of Obligation & Contracts', 3, 'None'),
- (229, 'Pol Sci 211', 'Good Governance & Social Resp.', 3, 'Hist 111'),
- (230, 'Math 211', 'Quantitative Techniques in Business', 3, 'Hist 111'),
- (231, 'CS 213', 'Accounting Information System', 3, 'CS 212'),
- (232, 'Bre 111', 'Business Research 1', 3, 'None'),
- (233, 'Mgt 212', 'Mgt. Planning & Central Operation', 3, 'Mgt 111'),
- (234, 'Entrep 211', 'Industrial Relations', 3, 'Mgt 211'),
- (235, 'Law 212', 'Law on Business organization', 3, 'Law 211'),
- (236, 'Tax 211', 'Income Taxation', 3, 'Acctg 212'),
- (237, 'Mgt 213', 'Production and opreations Mgt.', 3, 'Stat 113'),
- (238, 'Cost 212', 'Cost Acctg & Cost Mgt. II', 3, 'Cost 211'),
- (239, 'Econ 114', 'Management Economics', 3, 'Econ 113'),
- (240, 'Entrep 212', 'Phil. Business environment', 3, 'Entrep 211'),
- (241, 'Law 213', 'Law on sales, labor and other Coml. Laws', 3, 'Law 212'),
- (242, 'Net Sci 112', 'Physical Science', 3, 'Net Sci 111'),
- (243, 'Entrep 213', 'Entrepreneurial Business Mgt.', 3, 'Entrep 211'),
- (244, 'Soc Sci 111', 'Life and Works of rizal', 3, 'None'),
- (245, 'Bre 212', 'Business Research II', 3, 'Bre 111'),
- (246, 'Socio 111', 'Society and culture with family planning', 3, 'none'),
- (247, 'Entrep 214', 'Sales management', 3, 'Entrep 213'),
- (248, 'Mgt 215', 'Business policies and formulation', 3, 'none'),
- (249, 'Tax 212', 'Business and Transfer Taxes', 3, 'Tax 2111'),
- (250, 'Entrep 215', 'Strategic marketing management', 3, 'Mktg 211'),
- (251, 'OJT 211', 'On job training (200 hours)', 3, 'None'),
- (252, 'Eng 111', 'Communication Arts 1', 3, 'None'),
- (253, 'Fil 111', 'Komunikasyon Sa akademikong Filipino ', 3, 'None'),
- (254, 'Math 111', 'College Algebra', 3, 'None'),
- (255, 'Psycho 111', 'Gen & Criminal Psychology w/ TVET', 3, 'None '),
- (256, 'Crim 211', 'Intro to Criminology', 3, 'None'),
- (257, 'Hist 111', 'Phillippine History', 3, 'None'),
- (258, 'SMGT 111', 'Security Mgt. and Service', 3, 'None '),
- (259, 'PE 111', 'Fundamentals of Matial Arts', 2, 'None'),
- (260, 'NSTP 111', 'Military Science 11', 3, 'None'),
- (261, 'Eng 113', 'Technical Report Writing ', 3, 'Eng 112'),
- (262, 'LEA 213', 'Industrial Sec. Mgt w/ TVET', 3, 'None '),
- (263, 'CLJ 211', 'Criminal Law (Book 1)', 3, 'None'),
- (264, 'Socio 111', 'Society & Culture w/ Pop Ed.', 3, 'None'),
- (265, 'Econ 111', 'Basic Economics w/ TAR', 3, 'None '),
- (266, 'Crimtic 211', 'Personnel Idenfication w/ TVET ', 3, 'None'),
- (267, 'CS 111', 'Basic Software Pkcg Int. Application', 3, 'None'),
- (268, 'FIl 113', 'Masining na pagpapahayag', 3, 'Fil 112'),
- (269, 'PE 211', 'First Aid & water Survival ', 2, 'PE 121'),
- (278, 'CDI 215', 'Court Testimony', 3, 'CLJ 211'),
- (279, 'CDI 217', 'Fire Tech & Arson Investigation ', 3, 'None'),
- (280, 'LEA 217', 'Police Intellegence', 3, 'None'),
- (281, 'LEA 218', 'International Policing ', 3, 'None'),
- (282, 'PerDev 111', 'Personality Development ', 3, 'None'),
- (283, 'Crim 215', 'Criminology Research & Stat', 3, 'None '),
- (284, 'CLJ 214', 'Criminal Evidence ', 3, 'CLJ 211'),
- (285, 'Review 211', 'Refresher Evidence 1', 3, 'None'),
- (286, 'Eng 112', 'Speech and oral Com.', 3, 'Eng 111'),
- (287, 'Fil 120', 'Pagbasa at pagsulat tungo sa pananaliksik', 3, 'Fil 111'),
- (288, 'Math 121', 'Plane trigonometry', 3, 'Math 111'),
- (289, 'LEA 211', 'PHIL Criminal justice', 3, 'Crim 211'),
- (290, 'LEA 212', 'Police or. and administration', 2, 'none'),
- (291, 'Ethics 111', 'Ethics and values', 3, 'none'),
- (292, 'Pol Sci 111', 'Pol, Sci and Phil. Constitution', 3, 'none'),
- (293, 'Hum 111', 'Logic ', 3, 'none'),
- (294, 'PE 112', 'Disarming technique', 2, 'PE 111'),
- (295, 'NSTP 121', 'Military Science 12', 3, 'MS 11'),
- (296, 'Eng 113', 'Technical report wriring', 3, 'English 112'),
- (297, 'LEA 213', 'Industrial Sec. Mgt with TVET', 3, 'None'),
- (298, 'CLJ 211', 'Criminal Law (Book 1)', 3, 'none'),
- (300, 'Econ 111', 'Basic economics', 2, 'none'),
- (301, 'Crimtic 211', 'Basic Software pckg int App', 2, 'none'),
- (302, 'Fil 113', 'Masining na paglalayag', 3, 'Fil 112'),
- (303, 'PE 211', 'First Aid and water survival', 2, 'PE 211'),
- (304, 'Eng 114', 'Technical report writing 2', 3, 'none'),
- (305, 'Crim 213', 'Juvenile Deliquency and crime prevention', 3, 'none'),
- (306, 'CDI 2111', 'Police patrol operation', 3, 'none'),
- (307, 'Crimtic 212', 'Poloice photography', 3, 'none'),
- (308, 'LEA 215', 'Fund of criminal investigation', 3, 'none'),
- (309, 'SMGT 112', 'Security Mgt Services', 3, 'none'),
- (310, 'PE 114', 'Marmanship and combat shooting', 2, 'none'),
- (311, 'OJT', 'On job training', 2, 'none'),
- (312, 'LEA 214', 'Police Comparative System', 3, 'none'),
- (313, 'LEA 216', 'Police personnel and record mgt.', 3, 'none'),
- (314, 'CLJ 212', 'Criminal law (Book 2)', 3, 'none'),
- (315, 'Chem 111', 'General Chemistry', 3, 'none'),
- (316, 'CDI 212', 'traffic Mgt. and accident investigation', 2, 'none'),
- (317, 'Crimtic 213', 'Forensic ballistic', 2, 'none'),
- (318, 'Crimtic 214', 'Questioned Document', 3, 'none'),
- (319, 'CDI 211', 'Institutional Correction', 3, 'none'),
- (320, 'Crimtic 215', 'Polgraphy ', 3, 'none'),
- (321, 'LEA 219', 'Police Planning', 3, 'none'),
- (322, 'CLJ 213', 'Criminal procedure', 3, 'CLJ 212'),
- (323, 'Chem 211', 'Forensic chem and toxiclology', 3, 'Chem 111'),
- (324, 'CDI 216', 'White collar crime', 3, 'none'),
- (325, 'CDI 214', 'Organized crime', 3, 'none'),
- (326, 'Crimtic 216', 'Legal medicine', 3, 'none'),
- (327, 'CA 212', 'Non Institutional Correction', 3, 'CA 212'),
- (328, 'Prac 1 & 2', 'ON JOB TRAINING', 6, 'none'),
- (329, 'Review 211', 'Refreseher Course', 3, 'none'),
- (330, 'FTS 11', 'Field trip seminar', 3, 'none');
Creating Application
Step 1
Open Microsoft Visual Studio 2015 and create a new windows form application in c#.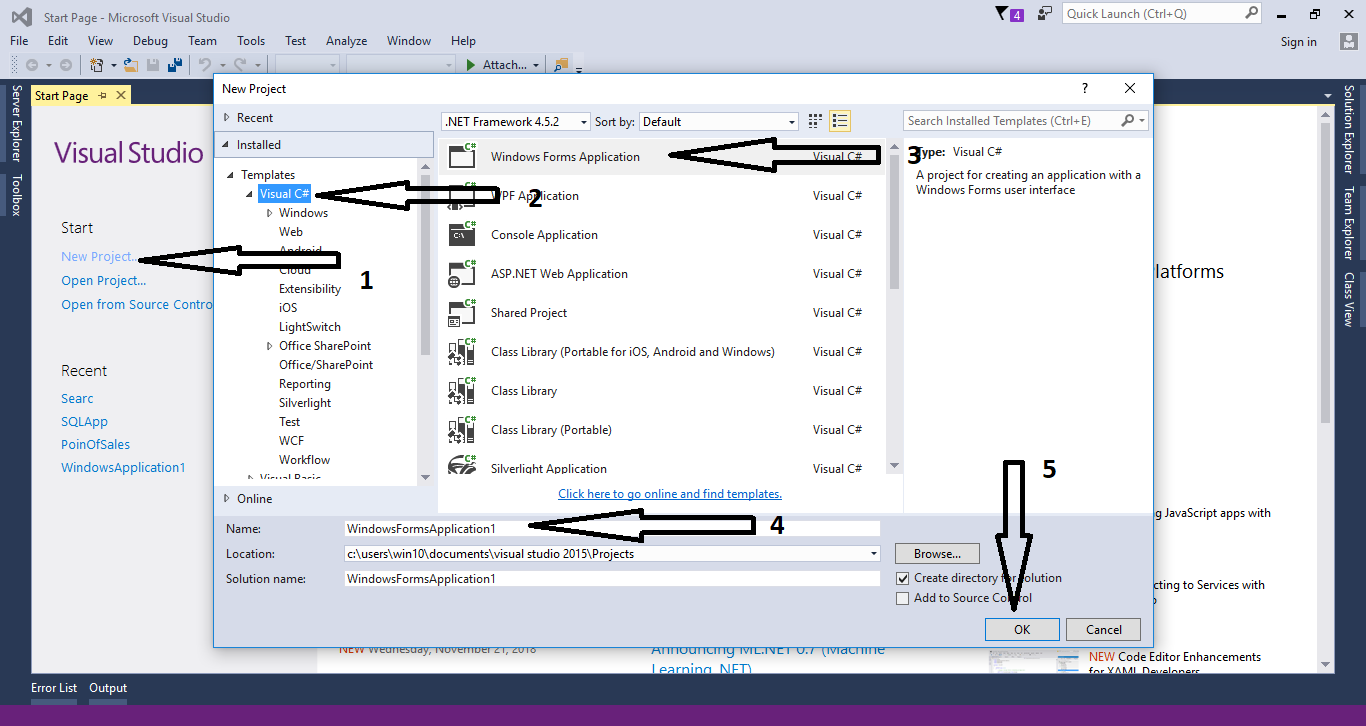
Step 2
Do the form just like shown below.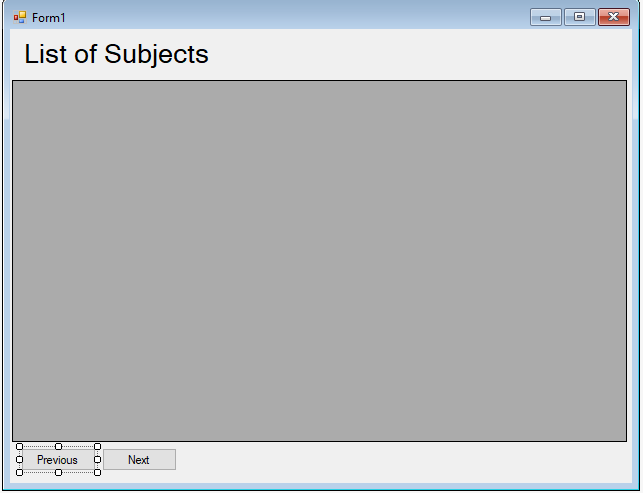
Step 3
Go to the code editor. After that, add a namespace to access MySQL libraries.- using MySql.Data.MySqlClient;
Step 4
Create a connection between C# and MySQL Database. After that, declare all the classes and variables that are needed.- MySqlConnection con = new MySqlConnection("server=localhost;user id=root;password=;database=dbsubjects;sslMode=none");
- MySqlCommand cmd;
- MySqlDataAdapter da;
- DataTable dt;
- String sql;
- int maxrow;
- int inc_value;
- int resultPerPage = 10;
- int startResult;
Step 5
Create a method to retrieve a single result in the MySQL database.- private void loadDataSingle(String sql)
- {
- try
- {
- con.Open();
- cmd.Connection = con;
- cmd.CommandText = sql;
- da.SelectCommand = cmd;
- da.Fill(dt);
- maxrow = dt.Rows.Count - 1;
- }
- catch (Exception ex)
- {
- MessageBox.Show(ex.Message);
- }
- finally
- {
- con.Close();
- da.Dispose();
- }
- }
Step 6
Create a method to retrieve the list of data in the MySQL database.- private void loadDataList(String sql,DataGridView dtg)
- {
- try
- {
- con.Open();
- cmd.Connection = con;
- cmd.CommandText = sql;
- da.SelectCommand = cmd;
- da.Fill(dt);
- dtg.DataSource = dt;
- }catch(Exception ex)
- {
- MessageBox.Show(ex.Message);
- }
- finally
- {
- con.Close();
- da.Dispose();
- }
- }
Step 7
Create a method for the previous function.- private void goto_Previous()
- {
- if (inc_value == 0)
- {
- return;
- }
- else if(inc_value > 0)
- {
- inc_value = inc_value - 1;
- startResult = inc_value * resultPerPage;
- }
- sql = "Select * from subject limit " + startResult + "," + resultPerPage + "";
- loadDataList(sql, dtg_list);
- }
Step 8
Create a method for the next function.- private void goto_Next()
- {
- if (inc_value != maxrow - 2)
- {
- inc_value = inc_value + 1;
- startResult = inc_value * resultPerPage;
- }
- else
- {
- if (inc_value == maxrow)
- {
- return;
- }
- }
- sql = "Select * from subject limit " + startResult + "," + resultPerPage + "";
- loadDataList(sql, dtg_list);
- }
Step 9
Double click the form and do the following codes for getting the total number rows of the table and limit the data to be displayed in the datagridview.- sql = "Select * from subject";
- loadDataSingle(sql);
- sql = "Select * from subject limit 10";
- loadDataList(sql, dtg_list);
Step 10
Double click the “Previous” button and call the method for the previous.- goto_Previous();
Step 11
Double click the “Next” button and call the method for the next.- goto_Next();