How to make a Calculator in C#
Submitted by janobe on Tuesday, July 19, 2016 - 16:11.
In this tutorial, I will show you how to create a calculator using C#.net. This is a simple calculator with functions that works accurately. It also contains procedures that are very easy to follow and can be done in a short period of time.
Step 1. Open Microsoft Visual Studio 2008 and create new Windows Form Application for C#. After that, do the form just like this.
Step 2. Go to the Solution Explorer, double-click the “View Code” to fire the code editor.
Step 3. In the code editor, declare the variables that are needed.
Step 4. Set the following codes for the function of the (0-9 ,clear and .) buttons .
Step 5. Set the following codes for the function of the (x,/,+,-) MDAS calculation.
Download the complete source code and run it on your computer.
For all students who need a programmer for your thesis system or anyone who needs a source code in any programming languages. You can contact me @ :
Email – [email protected]
Mobile No. – 09305235027 – tnt
Let's begin:
Step 1. Open Microsoft Visual Studio 2008 and create new Windows Form Application for C#. After that, do the form just like this.
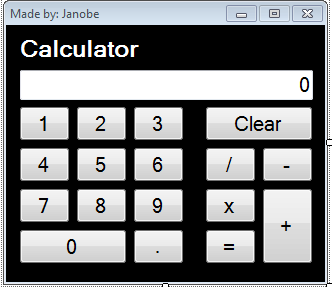
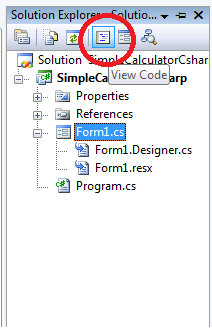
- double total = 0;
- string LOperator;
- private void btn1_Click(object sender, EventArgs e)
- {
- if (txtView.Text == "0")
- {
- txtView.Clear();
- txtView.Text += btn1.Text;
- }
- else
- {
- txtView.Text += btn1.Text;
- }
- }
- private void btn2_Click(object sender, EventArgs e)
- {
- if (txtView.Text == "0")
- {
- txtView.Clear();
- txtView.Text += btn2.Text;
- }
- else
- {
- txtView.Text += btn2.Text;
- }
- }
- private void btn3_Click(object sender, EventArgs e)
- {
- if (txtView.Text == "0")
- {
- txtView.Clear();
- txtView.Text += btn3.Text;
- }
- else
- {
- txtView.Text += btn3.Text;
- }
- }
- private void btn0_Click(object sender, EventArgs e)
- {
- if (txtView.Text == "0")
- {
- txtView.Clear();
- txtView.Text += btn0.Text;
- }
- else
- {
- txtView.Text += btn0.Text;
- }
- }
- private void btn8_Click(object sender, EventArgs e)
- {
- if (txtView.Text == "0")
- {
- txtView.Clear();
- txtView.Text += btn8.Text;
- }
- else
- {
- txtView.Text += btn8.Text;
- }
- }
- private void btn7_Click(object sender, EventArgs e)
- {
- if (txtView.Text == "0")
- {
- txtView.Clear();
- txtView.Text += btn7.Text;
- }
- else
- {
- txtView.Text += btn7.Text;
- }
- }
- private void btn9_Click(object sender, EventArgs e)
- {
- if (txtView.Text == "0")
- {
- txtView.Clear();
- txtView.Text += btn9.Text;
- }
- else
- {
- txtView.Text += btn9.Text;
- }
- }
- private void btn6_Click(object sender, EventArgs e)
- {
- if (txtView.Text == "0")
- {
- txtView.Clear();
- txtView.Text += btn6.Text;
- }
- else
- {
- txtView.Text += btn6.Text;
- }
- }
- private void btn5_Click(object sender, EventArgs e)
- {
- if (txtView.Text == "0")
- {
- txtView.Clear();
- txtView.Text += btn5.Text;
- }
- else
- {
- txtView.Text += btn5.Text;
- }
- }
- private void btn4_Click(object sender, EventArgs e)
- {
- if (txtView.Text == "0")
- {
- txtView.Clear();
- txtView.Text += btn4.Text;
- }
- else
- {
- txtView.Text += btn4.Text;
- }
- }
- private void btnDote_Click(object sender, EventArgs e)
- {
- if (!txtView.Text.Contains("."))
- {
- txtView.Text += btnDote.Text;
- }
- }
- private void btnClear_Click(object sender, EventArgs e)
- {
- txtView.Text = "0";
- total = 0;
- }
- private void btnPlus_Click(object sender, EventArgs e)
- {
- total += double.Parse(txtView.Text);
- LOperator = "plus";
- txtView.Clear();
- }
- private void btnminus_Click(object sender, EventArgs e)
- {
- total += double.Parse(txtView.Text);
- LOperator = "minus";
- txtView.Clear();
- }
- private void btnMultiply_Click(object sender, EventArgs e)
- {
- total += double.Parse(txtView.Text);
- LOperator = "multiply";
- txtView.Clear();
- }
- private void btnDivide_Click(object sender, EventArgs e)
- {
- total += double.Parse(txtView.Text);
- LOperator = "divide";
- txtView.Clear();
- }
- private void btnEqual_Click(object sender, EventArgs e)
- {
- switch (LOperator){
- case "plus":
- total = total + double.Parse(txtView.Text);
- txtView.Text = total.ToString();
- total = 0;
- LOperator = "";
- break ;
- case "minus":
- total = total - double.Parse(txtView.Text);
- txtView.Text = total.ToString();
- total = 0;
- LOperator = "";
- break;
- case "multiply":
- total = total * double.Parse(txtView.Text);
- txtView.Text = total.ToString();
- total = 0;
- LOperator = "";
- break;
- case "divide":
- total = total / double.Parse(txtView.Text);
- txtView.Text = total.ToString();
- total = 0;
- LOperator = "";
- break;
- default :
- txtView.Text = total.ToString();
- break;
- }
- }