How to Check if a String Ends with an Alphanumeric Character in Python
In this tutorial, we’ll learn how to program “How to Check if a String Ends with an Alphanumeric Character in Python.” The objective is to safely check whether a string ends with an alphanumeric character. This tutorial will guide you through the proper method for determining if a string ends with an alphanumeric character. So, let’s get started!
This topic is straightforward to understand. Just follow the instructions I provide, and you’ll complete it with ease. The program I’ll demonstrate will illustrate the correct way to check if a string ends with an alphanumeric character. So, let’s dive into the coding process!
Getting Started:
First you will have to download & install the Python IDLE's, here's the link for the Integrated Development And Learning Environment for Python https://www.python.org/downloads/.
Creating Main Function
This is the main function of the application. The following code will display a simple GUI in terminal console that will display program. To do this, simply copy and paste these blocks of code into the IDLE text editor.- import re
- regex = '[a-zA-Z0-9]$'
- def check_string(string):
- if(re.search(regex, string)):
- print("String Accepted!")
- else:
- print("String not Accepted!")
- if __name__ == '__main__' :
- while True:
- print("\n============ Check if a String Ends with an Alphanumeric Character ============\n\n")
- my_string = input("Enter a string: ")
- check_string(my_string)
- opt = input("\nDo you want to try again?(yes/no): ")
- if opt.lower() == 'yes':
- ret=False
- elif opt.lower() == 'no':
- ret=True
- print("Exiting program....")
- else:
- print("Please enter yes/no:")
- break
- if ret == False:
- continue
This script repeatedly prompts the user for input and checks whether the entered string ends with a letter or number, printing 'String Accepted!' if it does and 'String not Accepted!' otherwise.
Output:
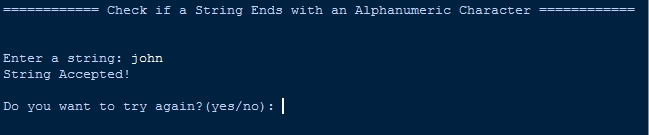
There you have it we successfully created How to Check if a String Ends with an Alphanumeric Character in Python. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for Python Language