How to Copy Contents to another Text File in Python
In this tutorial, we’ll learn how to program "How to Copy Contents to Another Text File in Python." We’ll focus on copying the contents from one text file to another. The objective is to copy the content safely and accurately to a new text file. A sample program will be provided to demonstrate the coding process.
This topic is straightforward to understand. Just follow the instructions, and you’ll be able to do it yourself with ease. The program I’ll show you demonstrates the correct way to copy content from one file to another efficiently. So, let’s start coding!
Getting Started:
First you will have to download & install the Python IDLE's, here's the link for the Integrated Development And Learning Environment for Python https://www.python.org/downloads/.
Creating Main Function
This is the main function of the application. The following code will display a simple GUI in terminal console that will display program. To do this, simply copy and paste these blocks of code into the IDLE text editor.- import shutil
- def append_files_method2(file1_path, file2_path):
- with open(file1_path, 'r') as file1:
- with open(file2_path, 'a') as file2:
- shutil.copyfileobj(file1, file2)
- while True:
- print("\n================= Copy Contents to another Text File =================\n\n")
- file1_path = 'sample1.txt'
- file2_path = 'sample2.txt'
- append_files_method2(file1_path, file2_path)
- print("Copied File Content Successfully!")
- opt = input("\nDo you want to try again?(yes/no): ")
- if opt.lower() == 'yes':
- ret=False
- elif opt.lower() == 'no':
- ret=True
- print("Exiting program....")
- else:
- print("Please enter yes/no:")
- break
- if ret == False:
- continue
This program copies the contents from one text file, sample1.txt, and appends them to another text file, sample2.txt, using Python's shutil.copyfileobj() function. It opens sample1.txt in read mode and sample2.txt in append mode, so new content is added without overwriting existing data. The program repeats this process based on user input until the user decides to exit.
Output:
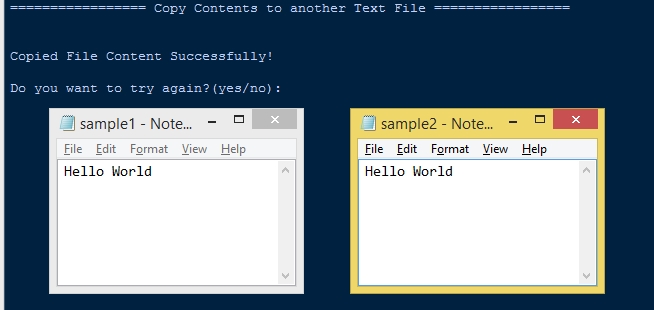
There you have it we successfully created How to Copy Contents to another Text File in Python. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for Python Language