How to Rotate Array Position in Console Terminal using Python
In this tutorial, we will program 'How to Rotate Array Positions in a Console Terminal using Python.' We will learn how to rotate the array positions by shifting the numbers. The objective is to safely rotate the array positions in an incrementing order. I will provide a sample program to demonstrate the actual coding process in this tutorial.
This topic is very easy to understand. Just follow the instructions I provide, and you will be able to do it yourself with ease. The program I will show you covers the basics of programming for rotating array positions. I will do my best to provide you with a simple method for incrementing the order of array positions. So, let's start coding
Getting Started:
First you will have to download & install the Python IDLE's, here's the link for the Integrated Development And Learning Environment for Python https://www.python.org/downloads/.
Creating Main Function
This is the main function of the application. The following code will display a simple GUI in terminal console that will display program. To do this, simply copy and paste these blocks of code into the IDLE text editor.- def reverse(start, end, arr):
- no_of_reverse = end-start+1
- count = 0
- while((no_of_reverse)//2 != count):
- arr[start+count], arr[end-count] = arr[end-count], arr[start+count]
- count += 1
- return arr
- def left_rotate_array(arr, size, d):
- start = 0
- end = size-1
- arr = reverse(start, end, arr)
- start = 0
- end = size-d-1
- arr = reverse(start, end, arr)
- start = size-d
- end = size-1
- arr = reverse(start, end, arr)
- return arr
- ret = False
- while True:
- print("\n================= Rotate Array Position =================\n\n")
- arr = [1, 2, 3, 4, 5, 6, 7, 8, 9]
- size = 9
- n = int(input("Enter a number 1-9: "))
- print('Original array:', arr)
- if(n <= size):
- print('Rotated array: ', left_rotate_array(arr, size, n))
- else:
- n = n % size
- print('Rotated array: ', left_rotate_array(arr, size, n))
- opt = input("\nDo you want to try again?(yes/no): ")
- if opt.lower() == 'yes':
- ret=False
- elif opt.lower() == 'no':
- ret=True
- print("Exiting program....")
- else:
- print("Please enter yes/no:")
- break
- if ret == False:
- continue
This Python script rotates an array to the left by a specified number of positions using a reverse function. It repeatedly prompts the user for a number to rotate the array and displays the rotated result. The loop continues until the user decides to exit the program.
Output:
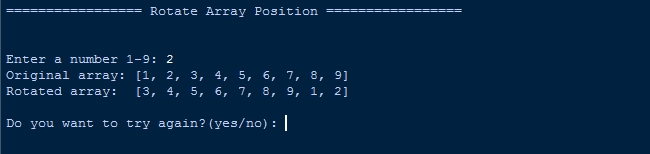
There you have it we successfully created How to Rotate Array Position in Console Terminal using Python. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for Python Language
Add new comment
- 46 views