How to Get Least Frequent Character in String using Python
In this tutorial, we will program 'How to Get the Least Frequent Character in a String using Python'. We will learn how to display the least frequent character(s) in a string. The objective is to automatically identify and display the least frequent character(s) in a given string. I will provide a sample program to demonstrate the actual coding process in this tutorial.
This topic is very easy to understand. Just follow the instructions I provide, and you can do it yourself with ease. The program I will show you covers the basics of programming to display the least frequent character in a string. I will do my best to provide you with a simple method for identifying and displaying the least frequent character. So, let's start with the coding.
Getting Started:
First you will have to download & install the Python IDLE's, here's the link for the Integrated Development And Learning Environment for Python https://www.python.org/downloads/.
Creating Main Function
This is the main function of the application. The following code will display a simple GUI in terminal console that will display program. To do this, simply copy and paste these blocks of code into the IDLE text editor.- from collections import Counter
- test_str = "sourcecodester"
- ret = False
- while True:
- print("\n================== Get Least Frequent Character in String ==================\n\n")
- print ("Original String: " + test_str)
- res = Counter(test_str)
- res = min(res, key = res.get)
- print ("The least characters in a string is : " + str(res))
- opt = input("\nDo you want to try again?(yes/no): ")
- if opt.lower() == 'yes':
- ret=False
- elif opt.lower() == 'no':
- ret=True
- print("Exiting program....")
- else:
- print("Please enter yes/no:")
- break
- if ret == False:
- continue
This script finds and displays the least frequent character in the string "sourcecodester". It repeatedly prompts the user to rerun the process or exit the program. Each time, it prints the least frequent character, allowing the user to choose whether to continue or exit based on their input.
Output:
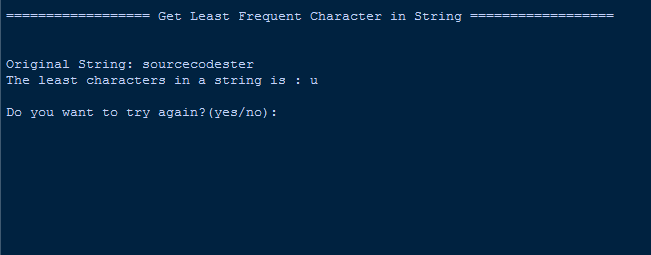
There you have it we successfully created How to Get Least Frequent Character in String using Python. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for Python Language
Add new comment
- 78 views