How to Create a Flash Message in PHP using Session Tutorial
In this tutorial, you will learn how to create a Flash Messages in PHP Language using PHP Session. The Flash Message that I am talking about is like the CodeIgniter's Flashdata Function. It allows the developer to store a temporary messages or data that can be access on the next request and after use.
How do Flash Messages Works?
The Flash Messages works something like the CodeIgniter's Flashdata Function where the developer can use it to store the process responses, error responses, messages, and other string data. This data will be stored using the PHP Session and when the data has called, the Flash Message stored in the session will b automatically removed.
Here, we will create a simple PHP application that has multiple buttons that triggers to save a Flash Message. After the successful saving of the flash message, the system will be redirected back to the page referrer and displays the message.
Getting Started
Download XAMPP as your local web server to run our PHP Script. After Installing the virtual server, open the XAMPP's Control Panel and start the Apache Server.
Download Bootstrap v5 for the interface design of the application that we'll be creating. After that move the library directory to the folder where you will store the source code on your end.
Creating The Interface
Below is the script of our Main Page which contains the elements codes for displaying flash messages and other displays. save this file as index.php.
- <?php require_once('function.php') ?>
- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8">
- <meta http-equiv="X-UA-Compatible" content="IE=edge">
- <meta name="viewport" content="width=device-width, initial-scale=1.0">
- <title>Flash Messages</title>
- <link rel="stylesheet" href="./Font-Awesome-master/css/all.min.css">
- <link rel="stylesheet" href="./css/bootstrap.min.css">
- <script src="./js/bootstrap.min.js"></script>
- <style>
- :root {
- --bs-success-rgb: 71, 222, 152 !important;
- }
- html,
- body {
- height: 100%;
- width: 100%;
- font-family: Apple Chancery, cursive;
- }
- .btn-info.text-light:hover,
- .btn-info.text-light:focus {
- background: #000;
- }
- </style>
- </head>
- <body class="bg-light">
- <nav class="navbar navbar-expand-lg navbar-dark bg-dark bg-gradient" id="topNavBar">
- <div class="container">
- <a class="navbar-brand" href="https://sourcecodester.com">
- Sourcecodester
- </a>
- <div>
- <b class="text-light">Flash Messages</b>
- </div>
- </div>
- </nav>
- <div class="container py-5" id="page-container">
- <h4 class="text-center"><b>Flash Messages Like CodeIgniter's Flashdata Functions.</b></h4>
- <!-- Displaying Flash Data if exist -->
- <!-- Looping All Flash messages -->
- <?php foreach($_SESSION['_flashdata'] as $key => $val): ?>
- <div class="my-2 px-3 py2 alert alert-<?= $key ?>">
- <div class="d-flex align-items-center">
- <div class="col-11">
- <!-- Displaying the Flash Message -->
- <p><?= get_flashdata($key) ?></p>
- </div>
- <div class="col-1 text-end">
- <button class="btn-close" type="button" onclick="this.closest('.alert').remove()"></button>
- </div>
- </div>
- </div>
- <?php endforeach; ?>
- <!-- Looping All Flash messages -->
- <?php endif; ?>
- <!-- Displaying Flash Data if exist -->
- <div class="col-lg-12 pt-5">
- <div class="row">
- <div class="col-md-3 px-2">
- <a href="set_messages.php?msg=info" class="btn btn-lg rounded-pill btn-pill btn-info">Show an Info Message</a>
- </div>
- <div class="col-md-3 px-2">
- <a href="set_messages.php?msg=success" class="btn btn-lg rounded-pill btn-pill btn-success">Show a Success Message</a>
- </div>
- <div class="col-md-3 px-2">
- <a href="set_messages.php?msg=error" class="btn btn-lg rounded-pill btn-pill btn-danger">Show an Error Message</a>
- </div>
- <div class="col-md-3 px-2">
- <a href="set_messages.php?msg=multiple" class="btn btn-lg rounded-pill btn-pill btn-default border">Show a Multiple Message</a>
- </div>
- </div>
- </div>
- </div>
- </body>
- </html>
Creating The Main Function
Next, we will be creating the PHP file that contains the function of storing the flash messages, getting the data, and deleting the flash message. Save this file as function.php.
- <?php
- if(session_status() === PHP_SESSION_NONE)
- // Sets a flash message data function
- function set_flashdata($key = "", $value = ""){
- $_SESSION['_flashdata'][$key] = $value;
- return true;
- }
- return false;
- }
- // Getting the flashdata
- function get_flashdata($key=""){
- $data = $_SESSION['_flashdata'][$key];
- return $data;
- }else{
- echo "<script> alert(' Flash Message \'{$key}\' is not defined. ')</script>";
- }
- }
Creating the Process File
The script below is the code is the sample PHP File that stores the flash messages and redirects to the Main Page. Save this file as set_messages.php.
- <?php require_once('function.php') ?>
- <?php
- echo "<script> alert('No message to set.'); location.href='./'</script>";
- }
- $msg = $_GET['msg'];
- switch ($msg){
- case 'info';
- set_flashdata('info',"<i class='fa fa-info-circle'></i> This is a Sample Info Message.");
- break;
- case 'success';
- set_flashdata('success',"<i class='fa fa-check'></i> This is a Sample Success Message.");
- break;
- case 'error';
- set_flashdata('danger',"<i class='fa fa-exclamation-triangle'></i> This is a Sample Error Message.");
- break;
- case 'multiple';
- set_flashdata('info',"<i class='fa fa-info-circle'></i> This is a Sample Info Message.");
- set_flashdata('success',"<i class='fa fa-check'></i> This is a Sample Success Message.");
- set_flashdata('danger',"<i class='fa fa-exclamation-triangle'></i> This is a Sample Error Message.");
- break;
- default:
- set_flashdata('danger',"Undefined Flash Message.");
- break;
- }
And here's the sample snapshot of the application.
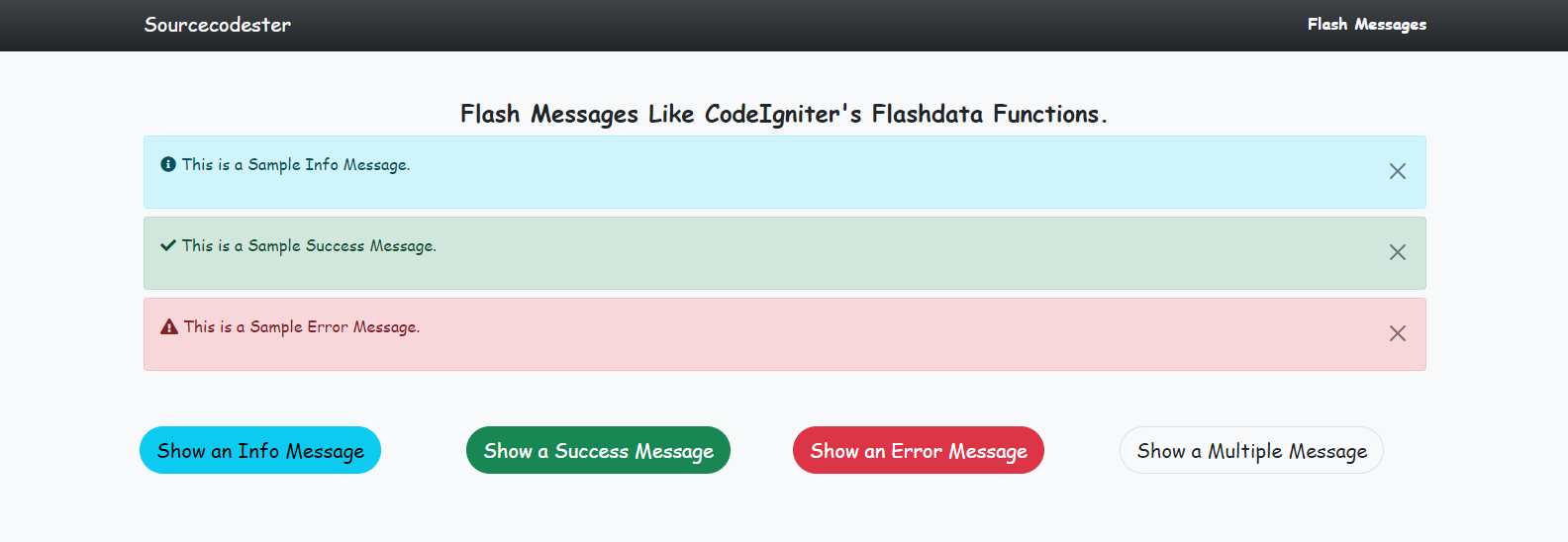
DEMO VIDEO
That's it! You can now test the application in your browser and see if we have met our goal on this tutorial. If ever you have encountered any errors, please review your source code by differentiating it from the source code I provided above. You can also test the working source code I created for this tutorial. You can download the source code zip file below this article.
That is the end of this tutorial. I hope you'll find this Flash Messages in PHP Tutorial useful for your current and future projects.
Happy Coding :)
Add new comment
- 3635 views