How to Delete JSON Element from an Array in JavaScript
How to Delete JSON Element from an Array in JavaScript
Introduction
In this tutorial we will create a How to Delete JSON Element from an Array in JavaScript. This tutorial purpose is to teach you how to delete an array from JSON object. This will thoroughly show you the simple way deleting an array . I will provide a sample program to show the actual coding of this tutorial.
This tutorial is simple and easy to understand just follow the instruction I provided and you can do it without a problem. This program can be use to any system or application that array for display data elements. I will give my best to provide you the easiest way of creating this program Delete an array from JSON object. So let's do the coding.
Before we get started:
Here is the link for the template that i used for the layout design https://getbootstrap.com/.
Creating The Interface
This is where we will create a simple interface for our application. This code will only display the html form and table. To create this simply copy and write it into your text editor, then save it as index.html.- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1"/>
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css"/>
- </head>
- <body>
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- </div>
- </nav>
- <div class="col-md-6 well">
- <hr style="border-top:1px dotted #ccc;"/>
- <div class="col-md-8">
- <table class="table table-bordered">
- <thead class="alert-info">
- <tr>
- </tr>
- </thead>
- </table>
- </div>
- <div class="col-md-4">
- <div class="form-group">
- <input type="text" id="firstname" class="form-control"/>
- </div>
- <div class="form-group">
- <input type="text" id="lastname" class="form-control"/>
- </div>
- <div class="form-group">
- <input type="text" id="address" class="form-control"/>
- </div>
- <div class="form-group">
- </div>
- </div>
- </div>
- </body>
- </html>
Creating JavaScript Function
This is where the main function of the application is. This code will allow you to delete an array from JSON object. To do this just copy and write these block of codes inside the text editor and save it as script.js.- var data = [];
- function displayData(){
- var table = "" ;
- for(var i in data){
- table += "<tr>";
- table += "<td>"
- + data[i].firstname +"</td>"
- + "<td>" + data[i].lastname +"</td>"
- + "<td>" + data[i].address +"</td>"
- + "<td><button class='btn btn-danger btn-sm' onclick='deleteData("+i+")'>Delete</button></td>";
- table += "</tr>";
- }
- document.getElementById("result").innerHTML = table;
- }
- function saveData(){
- let firstname = document.getElementById("firstname");
- let lastname = document.getElementById("lastname");
- let address = document.getElementById("address");
- if(firstname.value == "" || lastname.value == "" || address.value == ""){
- alert("Please complete the required field first!");
- }else{
- var member = {
- 'firstname': firstname.value,
- 'lastname': lastname.value,
- 'address': address.value,
- };
- data.push(member);
- displayData();
- firstname.value = "";
- lastname.value = "";
- address.value = "";
- }
- }
- function deleteData(index){
- delete data[index];
- alert("You have deleted a row");
- displayData();
- }
In the code above we just create one method to delete an array from the JSON object called deleteData(). This function will dynamically delete the array data in the table when you clicked the delete button.
Output:
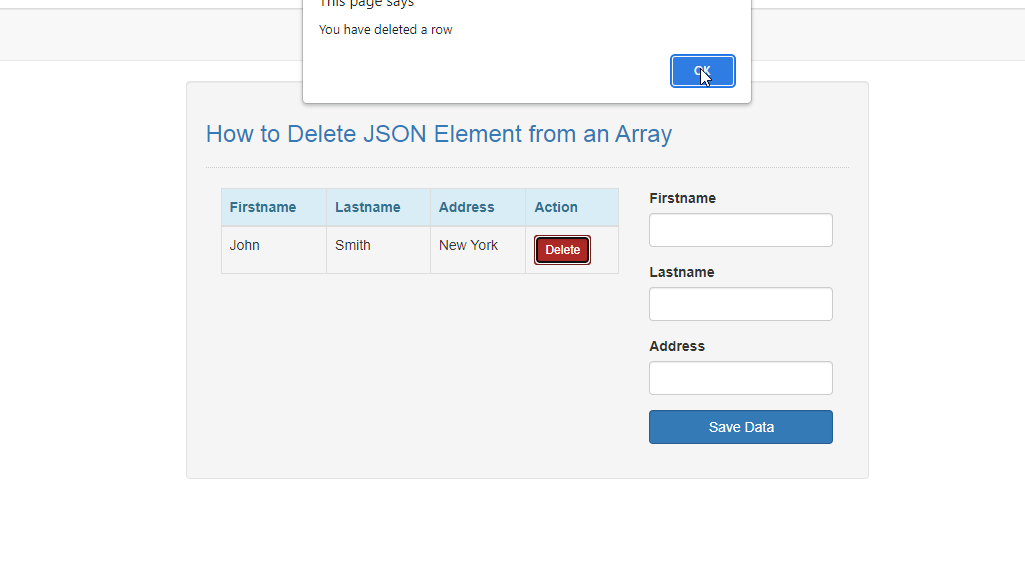
The How to Delete JSON Element from an Array in JavaScript source code that I provide can be download below. Please kindly click the download button.
There you have it we successfully created How to Delete JSON Element from an Array in JavaScript. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for JavaScript Language
Add new comment
- 461 views