How to Randomize your Content Order using jQuery in JavaScript
How to Randomize your Content Order using jQuery in JavaScript
Introduction
In this tutorial we will create a How to Randomize your Content Order using jQuery in JavaScript. This tutorial purpose is to teach you how to display your content in random order. This will thoroughly take the basic usage of jQuery . I will provide a sample program to show the actual coding of this tutorial.
This tutorial is simple and easy to understand just follow the instruction I provided and you can do it without a problem. This program can be use to any system or application that can apply jQuery plugin. I will give my best to provide you the easiest way of creating this program Random Order Content. So let's do the coding.
Before we get started:
First you have to download the jQuery plugin.
Here is the link for the jQuery that i used in this tutorial https://jquery.com/.
Lastly, this is the link for the template that i used for the layout design https://getbootstrap.com/.
Creating The Interface
This is where we will create a simple interface for our application. This code will only display the content of an article. To create this simply copy and write it into your text editor, then save it as index.html.- <!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8" name="viewport" content="width=device-width, initial-scale=1" />
- <link rel="stylesheet" type="text/css" href="css/bootstrap.css" />
- </head>
- <body onload="displayRandom();">
- <nav class="navbar navbar-default">
- <div class="container-fluid">
- </div>
- </nav>
- <div class="col-md-6 well">
- <hr style="border-top:1px dotted #ccc;"/>
- <div class="content alert alert-info">
- </div>
- <div class="content alert alert-info">
- </div>
- <div class="content alert alert-info">
- </div>
- <div class="content alert alert-info">
- </div>
- </div>
- </body>
- </html>
Creating JavaScript Function
This is where the main function of the application is. This code will randomize the order of your content in the html page. To do this just copy and write these block of codes inside the text editor and save it as script.js.- function displayRandom(){
- let contents = $(".content");
- for(var i = 0; i < contents.length; i++){
- let target = Math.floor(Math.random() * contents.length -1) + 1;
- let target2 = Math.floor(Math.random() * contents.length -1) +1;
- contents.eq(target).before(contents.eq(target2));
- }
- }
In the given code we first call the basic jQuery ready event to signal that the DOM of the page is now ready to be use. In this code we just simply created only one method called displayRandom(). This function will randomize the order of your content in the page. This code uses loops to read through the contents and then use Math() function to randomize the index position.
Output:
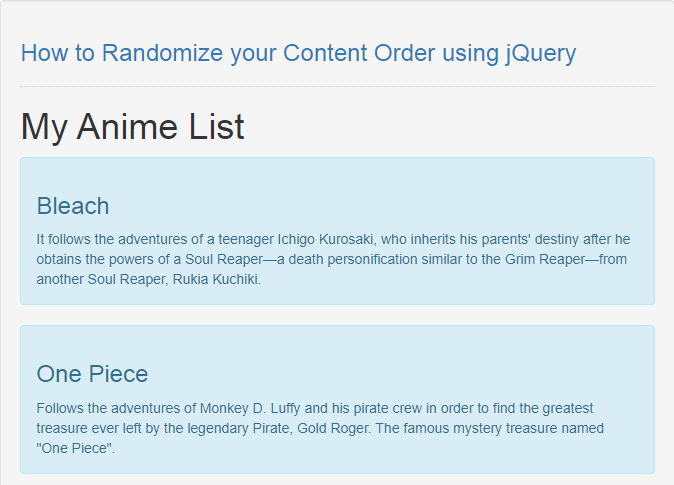
The How to Randomize your Content Order using jQuery in JavaScript source code that I provide can be download below. Please kindly click the download button.
There you have it we successfully created How to Randomize your Content Order using jQuery in JavaScript. I hope that this simple tutorial help you to what you are looking for. For more updates and tutorials just kindly visit this site. Enjoy Coding!
More Tutorials for JavaScript Language
Add new comment
- 117 views