Number To Words in Java
Submitted by donbermoy on Monday, December 29, 2014 - 12:05.
Language
This tutorial will teach you how to create a program that will convert a number and will become words in java.
So, now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of NumberToWords.java.
2. Import the io library as we will need a user input.
3. Now, we will declare our global variables. We will have variables for the inputting number, ones,tens, elevens, hundreds, thousands, and the zero. We will have only a maximum input of 3000.
We will create the BufferedReader class with variable in, and n as intger that will make as our input.
4. We will code first for inputting of the zero. We will just use the if and switch statement here. Have this code below:
5. Now, this code will be for the hundred and thousand when inputting 3 to 4 digits. We will use the '%' for the modulus as we have to get the zeroes and the remainder. Have this code below:
6. For the eleven to nineteen, we will filter such code with the if condition followed by the switch statement.
7. Then have this code for the ones and tens.
8. Remember that our conversion will have only its maximum number of 3000, so we must filter it. Have this code:
Output:
Here's the full code of this tutorial:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
- import java.io.*;
- static int n,thou,hun,tens,ones,zero,elevens;
- if (n==0){
- switch (n){
- }
- if ((n>=100) && (n<=3000)){
- thou = n/1000;
- n = n%1000;
- hun = n/100;
- n = n%100;
- switch(thou){
- }
- switch (hun) {
- }
- }
- if ((n>10) && (n<20)){
- tens = n / 10;
- ones = n;
- elevens = n % 10;
- switch (elevens){
- }
- }
- else {
- tens = n/10;
- n = n%10;
- ones = n;
- switch (tens) {
- }
- switch (ones){
- }
- }
- if (n>3000){
- }
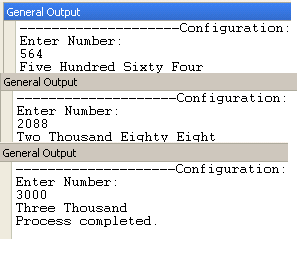
- import java.io.*;
- public class NumberToWords {
- static int n,thou,hun,tens,ones,zero,elevens;
- if (n==0){
- switch (n){
- }
- }
- if ((n>=100) && (n<=3000)){
- thou = n/1000;
- n = n%1000;
- hun = n/100;
- n = n%100;
- switch(thou){
- }
- switch (hun) {
- }
- }
- if ((n>10) && (n<20)){
- tens = n / 10;
- ones = n;
- elevens = n % 10;
- switch (elevens){
- }
- }
- if (n>3000){
- }
- else {
- tens = n/10;
- n = n%10;
- ones = n;
- switch (tens) {
- }
- switch (ones){
- }
- }
- }
- }
Note: Due to the size or complexity of this submission, the author has submitted it as a .zip file to shorten your download time. After downloading it, you will need a program like Winzip to decompress it.
Virus note: All files are scanned once-a-day by SourceCodester.com for viruses, but new viruses come out every day, so no prevention program can catch 100% of them.
FOR YOUR OWN SAFETY, PLEASE:
1. Re-scan downloaded files using your personal virus checker before using it.
2. NEVER, EVER run compiled files (.exe's, .ocx's, .dll's etc.)--only run source code.
Comments
Add new comment
- Add new comment
- 7072 views