Android Excel File Reader Application using Basic4Android - Tutorial Part 1
Submitted by donbermoy on Thursday, January 16, 2014 - 00:58.
Today, i will introduce a powered application in Android that views an Excel File. Some of the Android Phones today is not reliable to open and read an Excel File in their Android Phones. But, reading this tutorial makes you realize that you can program the Android to open an Excel File.
The first thing that you will consider is to copy an Excel File to the File Folder of your Basic4Android Application. You need to create an excel file like this one below and named it "book1.xls".
- The code above is the initialization of reading the table in an Excel file. The table1 here is the variable in my table. Note: Don't Forget to add the Library of Excel in the Library of Basic4Android.
- Given the code above is the same with Form_Load in Visual Basic. In our activity it have two Menu Item, the load table and saving table.
The
- I the code above had not found the book1.xls then it will prompt the user "Unable to open file". Otherwise, it will load the table of book1.xls.
Here is the partial code in our Main Program in Basic4Android:
Tomorrow I will post the complete code for this Excel File Reader Application in Android considering on how to have a formatting in Excel like TableSettings, SetColumnsWidths, ScrollChanged, AddRow, AddColumns, RemoveRow, HideRows, and many more functions to create an Excel File Application.
Best regards,
Engr. Lyndon R. Bermoy
IT Instructor/System Developer/Mobile Developer
09126450702
[email protected]
Follow and add me in my Facebook Account: www.facebook.com/donzzsky
Visit my page on Facebook at: https://www.facebook.com/BermzISware
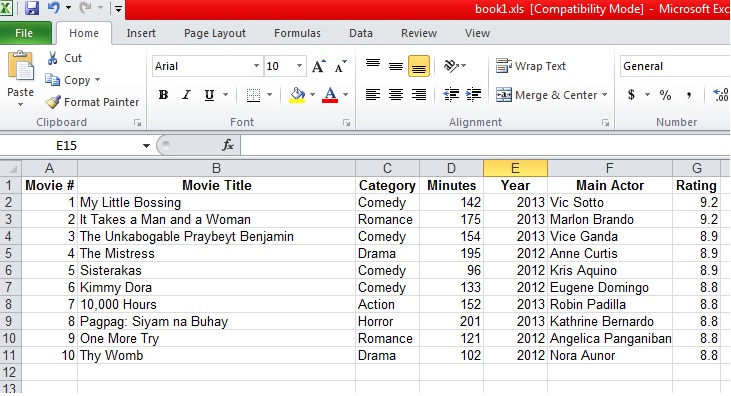
- Sub Globals
- Dim table1 As Table
- End Sub
- Sub Activity_Create(FirstTime As Boolean)
- Activity.Title = "Excel Example - Lyndon Bermoy"
- Activity.AddMenuItem("Load Table", "LoadTable")
- Activity.AddMenuItem("Save Table", "SaveTable")
- LoadTable(File.DirAssets, "Book1.xls")
- End Sub
LoadTable(File.DirAssets, "Book1.xls")
syntax is getting the information of our table created in Excel.
Next, we should have a procedure of clicking our load table in the excel. Write this code:
- Sub LoadTable_Click
- If File.Exists(File.DirRootExternal, "book1.xls") = False Then
- ToastMessageShow("Unable to open file.",True)
- Else
- LoadTable(File.DirRootExternal, "book1.xls")
- End If
- End Sub
- Sub Process_Globals
- End Sub
- Sub Globals
- Dim table1 As Table
- End Sub
- Sub Activity_Create(FirstTime As Boolean)
- Activity.Title = "Excel Example - Lyndon Bermoy"
- Activity.AddMenuItem("Load Table", "LoadTable")
- Activity.AddMenuItem("Save Table", "SaveTable")
- LoadTable(File.DirAssets, "Book1.xls")
- End Sub
- Sub LoadTable_Click
- If File.Exists(File.DirRootExternal, "book1.xls") = False Then
- ToastMessageShow("Unable to open file.", True)
- Else
- LoadTable(File.DirRootExternal, "book1.xls")
- End If
- End Sub
- Sub LoadTable(Dir As String, FileName As String)
- Dim workbook1 As ReadableWorkbook
- Dim moviesSheet As ReadableSheet
- workbook1.Initialize(Dir, FileName)
- moviesSheet = workbook1.GetSheet(0)
- If table1.IsInitialized Then
- Activity.RemoveAllViews 'remove the current table
- End If
- table1.Initialize(Me, "Table1", moviesSheet.ColumnsCount)
- table1.AddToActivity(Activity, 0, 0, 100%x, 100%y)
- For row = 0 To moviesSheet.RowsCount - 1
- Dim values(moviesSheet.ColumnsCount) As String
- For i = 0 To values.Length -1
- values(i) = moviesSheet.GetCellValue(i, row)
- Next
- If row = 0 Then
- table1.SetHeader(values)
- Else
- table1.AddRow(values)
- End If
- Next
- End Sub
- Sub SaveTable_Click
- 'first we create a writable workbook.
- 'the target File should be a NEW File.
- Dim newWorkbook As WritableWorkbook
- newWorkbook.Initialize(File.DirRootExternal, "1.xls")
- Dim sheet1 As WritableSheet
- sheet1 = newWorkbook.AddSheet("Movies", 0)
- 'add the headers To the sheet
- 'we create a special format For the headers
- Dim cellFormat As WritableCellFormat
- cellFormat.Initialize2(cellFormat.FONT_ARIAL, 12, True, False, False, _
- cellFormat.COLOR_GREEN)
- cellFormat.HorizontalAlignment = cellFormat.HALIGN_CENTRE
- cellFormat.SetBorder(cellFormat.BORDER_ALL, _
- cellFormat.BORDER_STYLE_MEDIUM, cellFormat.COLOR_BLACK)
- cellFormat.SetBorder(cellFormat.BORDER_BOTTOM, cellFormat.BORDER_STYLE_THICK, _
- cellFormat.COLOR_BLUE)
- cellFormat.VertivalAlignment = cellFormat.VALIGN_CENTRE
- cellFormat.BackgroundColor = cellFormat.COLOR_GREY_25_PERCENT
- Dim col As Int = 0
- For Each lbl As Label In table1.Header
- Dim cell As WritableCell
- cell.InitializeText(col, 0, lbl.Text)
- cell.SetCellFormat(cellFormat)
- sheet1.AddCell(cell)
- sheet1.SetColumnWidth(col, 15)
- col = col + 1
- Next
- sheet1.SetColumnWidth(1, 40)
- sheet1.SetRowHeight(0, 15)
- 'add the data
- Dim rowsFormat As WritableCellFormat
- rowsFormat.Initialize
- rowsFormat.HorizontalAlignment = rowsFormat.HALIGN_CENTRE
- For col = 0 To table1.NumberOfColumns - 1
- For row = 0 To table1.Size - 1
- Dim cell As WritableCell
- cell.InitializeText(col, row + 1, table1.GetValue(col, row))
- cell.SetCellFormat(rowsFormat)
- sheet1.AddCell(cell)
- Next
- Next
- 'Must call write AND close To save the data.
- newWorkbook.Write
- newWorkbook.Close
- End Sub
- Sub Table1_CellClick(Col As Int, Row As Int)
- table1.SetValue(Col, Row, "xxx")
- End Sub
- Sub Activity_Resume
- End Sub
- Sub Activity_Pause (UserClosed As Boolean)
- End Sub
Comments
Add new comment
- Add new comment
- 436 views