Autonumber Generator using Visual Basic.Net
Submitted by joken on Wednesday, September 25, 2013 - 13:24.
In this lesson, I’m going to show you how to generate a customize autonumber using visual basic and Microsoft access as our database. We can use this autonumber to identify a unique record in a table, and it should only one autonumber field is allowed in each table. In our situation, we will generate autonumber by this mechanism start with the start and increment with the increment value. Meaning a Start value plus the Increment value. After completing this course the output of this application it looks like as shown below.
To start building this application, add two groupbox, five labels, two buttons and a datagridview.
Then, double click the form and add the following code:
This code will simply load all the records from the database table “tblauto” into datagridview.
To insert new autonumber in our database, double click our “btgenerate” button, add the following code:
And you can now test your application by pressing “F5”.
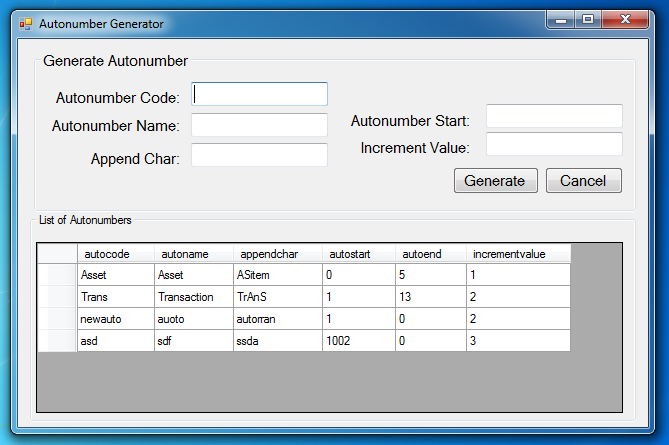
Object Property Settings Groupbox1 text Generate Autonumber Groupbox2 text list of Autonumbers Label1 text Autonumber code: Label2 text Autonumber Name: Label3 text Append Char: Label4 text Autonumber Start: Label5 text Increment Value: Button1 Name btngenerate Text Generate Button2 Name btncancel Text CancelThen, arrange all the object same with the final output shown above. Next, let’s add functionality to our application. Right the form, then click “view code” and add the following code.
- 'declare conn as connection and it will now a new connection because
- 'it is equal to Getconnection Function
- Dim con As OleDb.OleDbConnection = jokenconn()
- 'Represents an SQL statement or stored procedure to execute against a data source.
- Dim cmd As New OleDb.OleDbCommand
- Dim da As New OleDb.OleDbDataAdapter
- Public Function jokenconn() As OleDb.OleDbConnection
- Return New OleDb.OleDbConnection("Provider=Microsoft.Jet.OLEDB.4.0;Data Source=" & Application.StartupPath & "\autonumber.mdb")
- End Function
- Try
- con.Open()
- With cmd
- .Connection = con
- .CommandText = "Select * from tblauto"
- End With
- Dim publictable As New DataTable
- 'Gets or sets a Transact-SQL statement or stored procedure used
- 'to select records in the data source.
- da.SelectCommand = cmd
- da.Fill(publictable)
- 'fill the datagridview by getting fron dt
- DataGridView1.DataSource = publictable
- 'hide the first column of datagridview
- DataGridView1.Columns(0).Visible = False
- Catch ex As Exception
- End Try
- da.Dispose()
- Dim result As Integer
- Try
- con.Open()
- With cmd
- .Connection = con
- .CommandText = "Insert into tblauto(autocode,autoname,appendchar,autostart,incrementvalue) " & _
- " Values('" & txtcode.Text & "', '" & txtname.Text & "','" & txtchar.Text & "', " & _
- " '" & txtstart.Text & "','" & txtinc.Text & "')"
- result = cmd.ExecuteNonQuery
- If result = 0 Then
- Else
- End If
- End With
- Catch ex As Exception
- End Try
Comments
I NEED A CODE THAT CALCULATES AN AUTO NUMBER AND GIVES IT TO ME
Hi Joken,
I hope you're fine.
I just need a small code to create account numbers that are posted into access table.
Thank you
Adrian
Add new comment
- Add new comment
- 530 views