How to fix "Uncaught SyntaxError: Unexpected token '...' is not valid JSON" error in JavaScript?
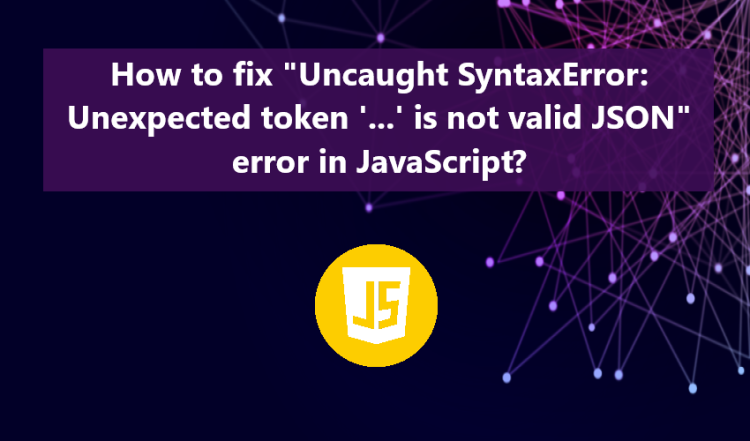
In this article, we will delve into the causes of and various solutions to resolve the JavaScript error message that appears as "Uncaught SyntaxError: Unexpected token '...' is not valid JSON". If you're encountering this JavaScript error and struggling to resolve it, this article will guide you through the fix and provide tips to prevent it from occurring again in the future.
Understanding SyntaxError in JavaScript
JavaScript SyntaxError is a type of error that occurs when an attempt is made to interpret an invalid syntax code. This error is thrown when there are tokens or token orders that do not adhere to the expected syntax during code parsing.
JavaScript encompasses various types of errors, and one of them is the SyntaxError. Alongside this error, there are other error messages such as TypeError, often triggered due to mistakes in writing JavaScript code, like the "TypeError: cannot set properties of undefined" error.
What Causes the "Uncaught SyntaxError: Unexpected token '...' is not valid JSON" Error in JavaScript?
The "Uncaught SyntaxError: Unexpected token '...' is not valid JSON" error message arises in JavaScript when you attempt to parse a JSON string into an object but provide an invalid string for parsing. Parsing a JSON string can be achieved using the JSON.parse() function, which requires a properly formatted string JSON as its argument.
For example, let's pretend that we have the following code:
- // Example Invalid JSON
- var data = "data1: 'Hello World!', data2: 'SourceCodester'";
- // Parsing JSON Data
- var parsedData = JSON.parse(data);
- // Output Parsed Data
- console.log(parsedData)
In the snippet provided above, we are attempting to create a JSON object from an invalid JSON string variable called data. Subsequently, we will attempt to parse the string value of this variable using the JSON.parse() function. Executing the provided script will result in an error, as shown in the following image:
Here's an another example script that leads to the error to emerge.
- // Example JSON
- var data = {data1: 'Hello World!', data2: 'SourceCodester'};
- // Parsing JSON Data
- var parsedData = JSON.parse(data);
- // Output Parsed Data
- console.log(parsedData)
In the case of the snippet above, the value of the variable data is a type of object. Attempting to parse a string JSON using the JSON.parse() function with an object type is not permissible because an object is not a valid JSON string.
Solutions
The most straightforward solution to resolve the "Uncaught SyntaxError: Unexpected token '...' is not valid JSON" error is to ensure that when we utilize or invoke the JSON.parse() function, we provide a valid JSON string to prevent the occurrence of the mentioned error. The following snippet illustrates the correct usage of this parsing function:
- // Example valid JSON
- var data = '{"data1":"Hello World!","data2":"SourceCodester"}';
- // Parsing JSON Data
- var parsedData = JSON.parse(data);
- // Output Parsed Data
- console.log(parsedData)
Furthermore, when implementing a dynamic script, it's considered a best practice to include code that validates or verifies the dynamic data to ensure the script's integrity and prevent errors that could lead to project execution or runtime crashes. The following sample snippet demonstrates how you can achieve this:
- // Example Invalid JSON
- var data1 = '"key1":"Hello World!","key2":"SourceCodester"';
- var data2 = '{"key1":"Hello World!","key2":"SourceCodester"}';
- // Parsing JSON Data1
- try{
- var parsedData1 = JSON.parse(data1);
- console.log(parsedData1)
- }catch (e){
- console.log("data1 variable is not a valid JSON");
- }
- // Parsing JSON Data1
- try{
- var parsedData2 = JSON.parse(data2);
- console.log(parsedData2)
- }catch (e){
- console.log("data2 variable is not a valid JSON");
- }
Conclusion
In simpler terms, the "Uncaught SyntaxError: Unexpected token '...' is not valid JSON" error occurs when there is an issue with the syntax of tokens or their order when executing the JSON.parse() function. This error can be resolved by ensuring that you provide a valid JSON string when using this function.
There you have it! I hope this article helps you resolve the issue you're currently facing and equips you with valuable knowledge to prevent errors from occurring during your project's development phase in the future.
You may also want to explore the following topics to enhance your programming knowledge and skills further:
- Fixing the JavaScript Error Message "DOMException: Failed to execute 'appendChild' on 'Node'"
- Returning Response Data from an Asynchronous Call in JavaScript
- Solving the JS "TypeError: Cannot read properties of null (reading 'addEventListener')" Issue
Explore more on this website for additional Free Source Code, Tutorials, and Articles covering various programming languages.
Happy Coding =)
- 7134 views