How to return response data from an Asynchronous Call in JavaScript
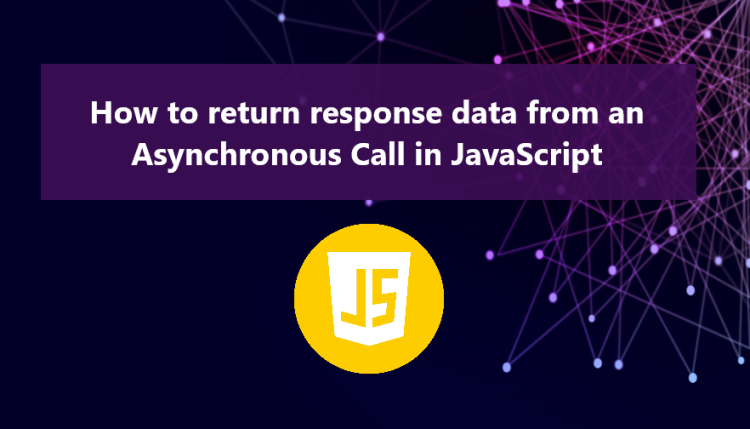
This article tackles into the subject of retrieving the output data from an asynchronous function in the JavaScript (or JS) programming language. Here, I'll present straightforward code examples that illustrate the common approach of obtaining response data. However, this approach fails when used within an asynchronous function call, and I'll also explain the remedy for this issue.
Understanding Asynchronous Calls/Functions in JavaScript
Asynchronous programming is a methodology that enables your software to initiate a task that might require a considerable amount of time to finalize. Meanwhile, your software remains responsive to other events without being forced to wait for the completion of that task. Once the task concludes, the resulting output becomes visible within your software.
Here's an example of an Asynchronous Function:
- function sampleFunction(){
- return new Promise((resolve)=>{
- setTimeout(()=>{
- resolve("Sample Function Process is done ...")
- }, 5000)
- })
- }
The provided code illustrates a basic Asynchronous function, which delivers a straightforward textual response upon the completion of a successful procedure.
Within a basic JavaScript function, we have the ability to directly yield the response without encountering errors, providing us with the precise outcome we anticipated. The following instance illustrates this concept:
- function sampleFunction(){
- return "Sample Function Process is done ...";
- }
The method demonstrated above is unsuitable for retrieving a response from an asynchronous function. If this technique is used, there's a likelihood of receiving null, undefined, or inaccurate response data. As mentioned earlier, asynchronous functions takes a while to complete the process.
Returning the Exact Response Data from Asynchronous Function
To return the correct response data from an Asynchronous function we can use await operator of Javascript
What is await opertor in JS?
The await operator is used to pause execution and obtain the value produced upon the resolution of a Promise. Its usage is limited to within an async function or at the module's highest level.
For Example:
- function sampleFunction(){
- return new Promise((resolve)=>{
- setTimeout(()=>{
- resolve("Sample Function Process is done ...")
- }, 5000)
- })
- }
- const SampleButton = document.getElementById('testButton')
- const SampleEl = document.getElementById('testEl')
- SampleButton.addEventListener('click', async function(){
- var response = await sampleFunction()
- SampleEl.innerText = response
- })
The provided code shows the proper method of obtaining data from an asynchronous function. Given that the response text data is derived from the sampleFunction and takes 5 seconds to return, the utilization of the await operator will temporarily pause the process until the function completes its execution.
There you go! I hope this How to return response data from an Asynchronous Call in JavaScript article helps you in your current situation and you find this useful knowledge for your future projects using JavaScript.
Explore more on this website for more Tutorials, Free Source Code, and Articles that tackles about fixing some possible error you may encounter in your project development.
Here are some other Articles that might help you also:
- "PHP Warning: foreach() argument must be of type array|object ..." [Solved]
- "TypeError: 'dict_values' object is not subscriptable" in Python [Solved]
- "AttributeError: 'DataFrame' object has no attribute 'concat'" in Python [Solved]
- 73 views