How to Fill DataGridView with Data Based on ComboBox
Submitted by janobe on Saturday, December 28, 2013 - 18:08.
Today, I will teach how to fill the data into a Combobox in the DataGridView Using Visual Basic 2008 and MySQL Database. This tutorial will show you how to retrieve the list of records that you have saved in MySQL Database into a ComboBox in the DataGridView.
Let’s Begin:
First, create a table in MySQL Database named "member"
Then, insert all the records in the table that you have created.
Open the Visual Basic 2008, create a Project and set up your Form just like this.
Double click the Form, then set up your connection in MySQL Database and declare all the variables and classes that you needed above the
After that, inside the
Complete Source Code is included.
- (1, 'Janno Palacios'),
- (2, 'Gerlie joy Castaños'),
- (3, 'John Paul Arroz'),
- (4, 'michael alejano'),
- (5, 'Jeszel R. alvarez'),
- (6, 'Mary Angelie I. Sitjar'),
- (7, 'Raffy Buendia'),
- (8, 'Jimmelyn Espraguera'),
- (9, 'joean mar m genit'),
- (10, 'Justine Ann Gomez'),
- (11, 'Amy Rose'),
- (12, 'Dolan Cuevas'),
- (13, 'clefhord john S macumao'),
- (14, 'Michael Ian C. Ruiz'),
- (15, 'lourdmaro'),
- (16, 'oscar neil s ilao'),
- (17, 'lyn rose'),
- (18, 'Ruselo P. Flores'),
- (19, 'Dennis Michael U. Berzuela'),
- (20, 'Jasper Gabat'),
- (21, 'Shanlee Marie Bongaita'),
- (22, 'ritchelle gatoc'),
- (23, 'Sherlyn F. Dolfo'),
- (24, 'july ganza'),
- (25, 'lanie pescasiosa'),
- (26, 'Marjorie'),
- (27, 'Giv Hamsleigh B. Javellana'),
- (28, 'Joshua J. Labrador'),
- (29, 'VANESSA G. BAÑEZ'),
- (30, 'Margie Rose Tondo'),
- (31, 'Gergil Luz Argamaso'),
- (32, 'Cheryl Tabasin'),
- (33, 'MYLIN I. MONTILLA'),
- (34, 'charkien palmos'),
- (35, 'Kim Ruel N Lo-on'),
- (36, 'james mesas'),
- (37, 'mario maravilla'),
- (38, 'janry apatan'),
- (39, 'janney joy kim')
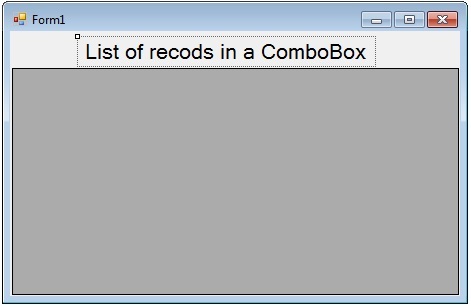
Form_Load
.
- Imports MySql.Data.MySqlClient
- Public Class Form1
- 'set up the MySQL Connection
- Dim con As MySqlConnection = New MySqlConnection("server=localhost;user id=root;database=member")
- 'a set of commands in MySQL
- Dim cmd As New MySqlCommand
- 'a Bridge between a database and datatable for retrieving and saving data.
- Dim da As New MySqlDataAdapter
- 'a specific table in the database
- Dim dt As New DataTable
- 'declare variable as string
- Dim sql As String
- Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
- End Sub
- End Class
Form_Load
, do the following codes for retrieving the list of records in MySQL Database into a ComboBox in the DataGridView and it will look like this.
- Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
- Try
- 'opening connection
- con.Open()
- 'stores your query in the varialble(sql)
- sql = "select name from names"
- 'set your MySQL COMMANDS for holding the data to be executed
- With cmd
- .Connection = con
- .CommandText = sql
- End With
- 'filling data in the table
- da = New MySqlDataAdapter(sql, con)
- dt = New DataTable
- da.Fill(dt)
- 'declaring variable that set as combobox in the column on the datagridview.
- Dim combo As New DataGridViewComboBoxColumn
- 'declaring variable that represents the row in the datatable
- Dim row As DataRow
- 'adding the data in a combobox
- With combo
- 'for each row in the datatable
- For Each row In dt.Rows
- 'adding a list of records in the combobox
- .Items.Add(row.Item("name").ToString)
- 'name of combobox
- .Name = "Name"
- Next
- End With
- 'add the combobox in the column in the datagridview
- DataGridView1.Columns.Add(combo)
- Catch ex As Exception
- End Try
- da.Dispose()
- 'Close the connection
- End Sub