Updating Data in VB.Net and SQL Server 2018
Submitted by janobe on Monday, October 14, 2019 - 19:10.
This is the continuation of my previous tutorial which is Retrieving Data in VB.Net and SQL Server 2018. This time I will teach you how to update the data in VB.Net and SQL Server 2018. In here, you will be able to update the data from the database. This method is so simple and easy to follow if you are a newbie in programming. Let's begin.
Creating Application
Step 1
Open Retrieving Data in VB.Net and SQL Server 2018.Step 2
Add a button in the Form and design the Form just like shown below.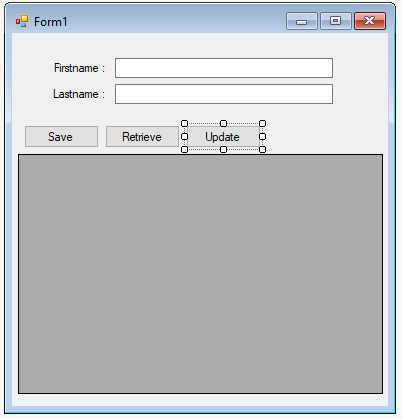
Step 3
Press F7 to open the code editor. In the code editor, add a method for retrieving single result data in the database.- Private Sub retrieveSingleResult(sql As String)
- Dim da As New SqlDataAdapter
- Dim dt As New DataTable
- Try
- con.Open()
- cmd = New SqlCommand
- With cmd
- .Connection = con
- .CommandText = sql
- End With
- da.SelectCommand = cmd
- da.Fill(dt)
- txtFname.Text = dt.Rows(0).Item(1)
- txtLname.Text = dt.Rows(0).Item(2)
- Catch ex As Exception
- MsgBox(ex.Message)
- Finally
- con.Close()
- da.Dispose()
- End Try
- End Sub
Step 4
Write the following code for passing the data from the datagridview to the textboxes when the current cell is clicked.- Private Sub DataGridView1_CellClick(sender As Object, e As DataGridViewCellEventArgs) Handles DataGridView1.CellClick
- sql = "SELECT * FROM tblperson WHERE PersonID=" & DataGridView1.CurrentRow.Cells(0).Value
- retrieveSingleResult(sql)
- End Sub
Step 5
Create a method for updating data in the database.- Private Sub updateData(sql As String)
- Try
- con.Open()
- cmd = New SqlCommand
- With cmd
- .Connection = con
- .CommandText = sql
- Result = .ExecuteNonQuery()
- End With
- If Result > 0 Then
- MsgBox("Data has been updated in the database")
- End If
- Catch ex As Exception
- MsgBox(ex.Message)
- Finally
- con.Close()
- End Try
- End Sub
Step 6
Go back to the design view, double click the “Update” button to open theclick event
handler of it. After that, add this code inside the “button3_clicked” event to update the data in the database.
- Private Sub Button3_Click(sender As Object, e As EventArgs) Handles Button3.Click
- sql = "UPDATE tblperson SET Fname='" & txtFname.Text & "',Lname='" & txtLname.Text & "' WHERE PersonID=" & DataGridView1.CurrentRow.Cells(0).Value
- saveData(sql)
- txtFname.Clear()
- txtLname.Clear()
- End Sub
Previous Tutorial
The complete source code is included. You can download it and run it on your computer. For any questions about this article. You can contact me @ Email – [email protected] Mobile No. – 09305235027 – TNT Or feel free to comment below.Add new comment
- 3356 views