Python - Pygame Adding Sound To The Game
Submitted by razormist on Tuesday, December 5, 2017 - 16:32.
In this tutorial we will do the Adding Sound to the Game. Pygame is a Free and Open Source python programming language framework for making game applications. It is highly portable and runs on nearly every platform and operating system. It is highly recommended for beginner to learn Pygame when making basic games. So let's now do the coding...
There you have it we successfully Add the Sound To The Game. I hope that this simple tutorial help you to what you are looking for and enhance your programming capabilities. For more updates and tutorials just kindly visit this site. Enjoy Coding!!
Getting Started
First you will have to download & install the Python IDLE's, here's the link for the Integrated Development And Learning Environment for Python https://www.python.org/downloads/. After Python IDLE's is installed, open the command prompt then type "python -m pip install pygame", and hit enter.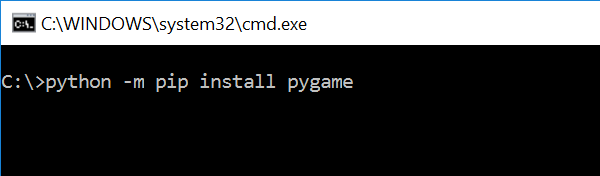
Importing Modules
After setting up the installation, run the IDLE and click file and then new file. After that a new window will appear containing a black file this will be the text editor for the python. Then copy code that I provided below and paste it inside the IDLE text editor- import pygame, os
- from pygame.locals import *
Writing The Variables
We will assign the a certain variables that we will need to declare later in the main loop. To do that just kindly write this code inside your text editor.- # Game Initialization
- pygame.init()
- # Initialize Sound Files
- pygame.mixer.music.load('Sounds/sound.wav')
- # Center the Game Application
- os.environ['SDL_VIDEO_CENTERED'] = '1'
- # Game Resolution
- screen_width=800
- screen_height=600
- screen=pygame.display.set_mode((screen_width, screen_height))
- # Color
- white=(255, 255, 255)
- black=(0, 0, 0)
- yellow=(255, 255, 0)
- #Text Renderer
- def text_format(text, textFont, textSize, textColor):
- font=pygame.font.Font(textFont, textSize)
- newText=font.render(text, 0, textColor)
- return newText
- # Framerate
- clock=pygame.time.Clock()
- fps=30
- # Fonts
- font='fonts/Retrotown.ttf'
Main Function
This is the Main Code for the Pygame application. The code contains a several input function that will allow to act accordingly and render to the application. To do that just write this code inside the IDLE text editor.- def main_menu():
- menu=True
- selected=""
- while menu:
- for event in pygame.event.get():
- if event.type==pygame.QUIT:
- pygame.quit()
- quit()
- if event.type==pygame.KEYDOWN:
- if event.key==pygame.K_ESCAPE:
- pygame.quit()
- quit()
- if event.key==pygame.K_UP:
- selected="play"
- elif event.key==pygame.K_DOWN:
- selected="stop"
- if event.key==pygame.K_SPACE:
- if selected=="play":
- # Play The Sound
- pygame.mixer.music.play(-1, 0.0)
- elif selected=="stop":
- # Stop The Sound
- pygame.mixer.music.stop()
- screen.fill(black)
- title=text_format("Pygame - Adding Sound To The Game", font, 40, white)
- if selected=="play":
- text_play = text_format("PLAY", font, 50, yellow)
- else:
- text_play = text_format("PLAY", font, 50, white)
- if selected=="stop":
- text_stop=text_format("STOP", font, 50, yellow)
- else:
- text_stop = text_format("STOP", font, 50, white)
- title_rect=title.get_rect()
- play_rect=text_play.get_rect()
- stop_rect=text_stop.get_rect()
- screen.blit(title, (screen_width/2 - (title_rect[2]/2), 50))
- screen.blit(text_play, (screen_width/2 - (play_rect[2]/2), 250))
- screen.blit(text_stop, (screen_width/2 - (stop_rect[2]/2), 400))
- pygame.display.update()
- clock.tick(fps)
- pygame.display.set_caption("Pygame - Adding Sound To The Game")
Initializing The Game
This is the Initialization code, this will run the entire code when the application start. It will render the Main Loop to display the specific methods. To do that just write this code inside the IDLE text editor.- # Initialize The Game
- main_menu()
- pygame.quit()
- quit()
Add new comment
- 357 views