Inline CRUD in PHP with jQuery AJAX
Submitted by alpha_luna on Wednesday, September 7, 2016 - 09:09.
If you are looking for Inline CRUD in PHP with jQuery AJAX then you are at the right place. In this simple tutorial, we are going to perform Create, Read, Update and Delete functions within the table. In the previous tutorial, we already have seen an example for Inline Adding Data, Inline Editing Data and Inline Deleting Data. Inline CRUD you can add a new row and update the data automatically using jQuery AJAX.
You can also check the live demo of this simple tutorial, so you can get an idea and you can try this out, let's start coding.
Create Markup Editable
This simple source code displaying the data from the database and the user can edit the data in the table row and it will save automatically in the database via jQuery AJAX. The source code is,
Create Inlide Add
This simple script creates a simple row with SAVE and CANCEL options button. After the user entering the data in the row, the user clicks the SAVE button to insert in the database. Then, the data inserted in the database we are going to display it to the table as the new record or new data inserted.
Create Inline Edit and Delete
This script contains the Edit and Delete operations to the row. The script is,
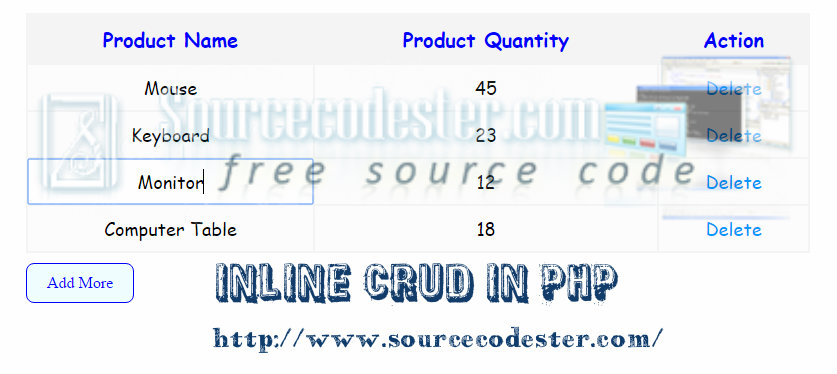
- <table class="tbl-qa">
- <thead>
- <tr>
- </tr>
- </thead>
- <tbody id="table-body">
- <?php
- if(!empty($row_product)) {
- foreach($row_product as $k=>$v) {
- ?>
- <tr class="table-row" id="table-row-<?php echo $row_product[$k]["id"]; ?>">
- <td contenteditable="true" onBlur="saveToDatabase(this,'product_name','<?php echo $row_product[$k]["id"]; ?>')" onClick="editRow(this);">
- <?php echo $row_product[$k]["product_name"]; ?>
- </td>
- <td contenteditable="true" onBlur="saveToDatabase(this,'product_qty','<?php echo $row_product[$k]["id"]; ?>')" onClick="editRow(this);">
- <?php echo $row_product[$k]["product_qty"]; ?>
- </td>
- <td>
- <a class="ajax-action-links" onclick="deleteRecord(<?php echo $row_product[$k]["id"]; ?>);">
- Delete
- </a>
- </td>
- </tr>
- <?php
- }
- }
- ?>
- </tbody>
- </table>
- <script>
- function addToDatabase() {
- var product_name = $("#product_name").val();
- var product_qty = $("#product_qty").val();
- $("#confirmAdd").html('<img src="loaderIcon.gif" />');
- $.ajax({
- url: "add.php",
- type: "POST",
- data:'product_name='+product_name+'&product_qty='+product_qty,
- success: function(data){
- $("#new_row_ajax").remove();
- $("#add-more").show();
- $("#table-body").append(data);
- }
- });
- }
- </script>
- <script>
- function save_tO-Database(editableObj,column,id) {
- $(editableObj).css("background","#FFF url(loaderIcon.gif) no-repeat right");
- $.ajax({
- url: "edit.php",
- type: "POST",
- data:'column='+column+'&editval='+$(editableObj).text()+'&id='+id,
- success: function(data){
- $(editableObj).css("background","#FDFDFD");
- }
- });
- }
- function delete_Record_in_Database(id) {
- if(confirm("Are you sure you want to delete this row?")) {
- $.ajax({
- url: "delete.php",
- type: "POST",
- data:'id='+id,
- success: function(data){
- $("#table-row-"+id).remove();
- }
- });
- }
- }
- </script>
Output
- How to Add
- How to Edit
- How to Delete
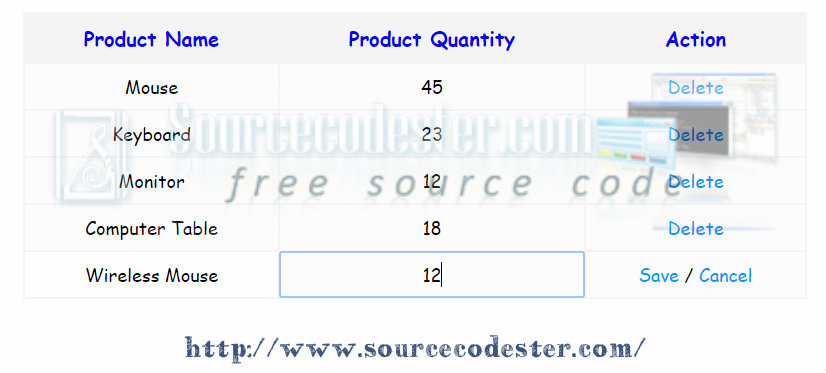
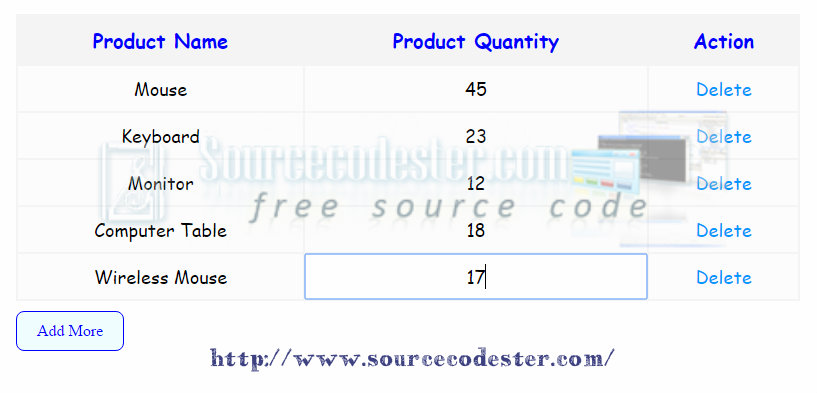
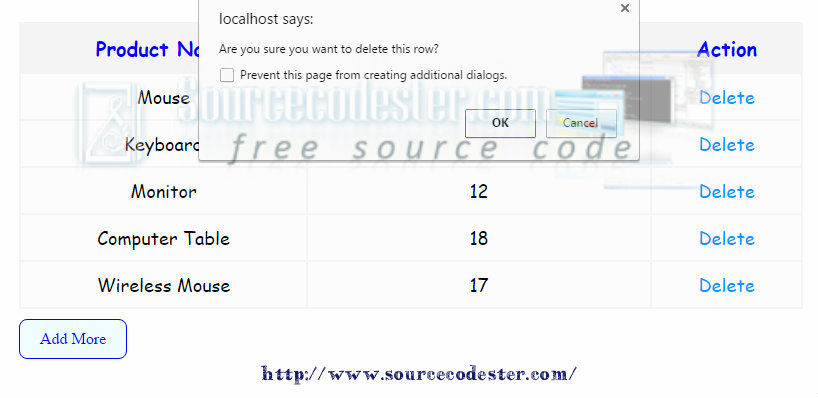
Learn by Examples
Examples are better than thousands of words. Examples are often easier to understand than text explanations. Share us your thoughts and comments below. Thank you so much for dropping by and reading this tutorial post. For more updates, don’t hesitate and feel free to visit this website more often and please share this with your friends or email me at [email protected]. Practice Coding. Thank you very much.Add new comment
- 878 views